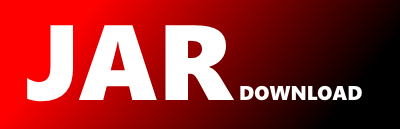
org.eclipse.elk.alg.layered.Layered.melk Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2015, 2020 Kiel University and others.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* SPDX-License-Identifier: EPL-2.0
*******************************************************************************/
package org.eclipse.elk.alg.layered
import java.util.List
import org.eclipse.elk.alg.layered.LayeredLayoutProvider
import org.eclipse.elk.alg.layered.components.ComponentOrderingStrategy
import org.eclipse.elk.core.math.ElkPadding
import org.eclipse.elk.core.options.Direction
import org.eclipse.elk.core.options.EdgeRouting
import org.eclipse.elk.core.options.HierarchyHandling
import org.eclipse.elk.core.options.PortAlignment
import org.eclipse.elk.core.util.ExclusiveBounds
import org.eclipse.elk.core.options.TopdownNodeTypes
/**
* Declarations for the ELK Layered layout algorithm.
*/
bundle {
metadataClass options.LayeredMetaDataProvider
idPrefix org.eclipse.elk.layered
}
algorithm layered(LayeredLayoutProvider) {
label "ELK Layered"
description
"Layer-based algorithm provided by the Eclipse Layout Kernel. Arranges as many edges as
possible into one direction by placing nodes into subsequent layers. This implementation
supports different routing styles (straight, orthogonal, splines); if orthogonal routing is
selected, arbitrary port constraints are respected, thus enabling the layout of block
diagrams such as actor-oriented models or circuit schematics. Furthermore, full layout of
compound graphs with cross-hierarchy edges is supported when the respective option is
activated on the top level."
metadataClass options.LayeredOptions
category org.eclipse.elk.layered
features self_loops, inside_self_loops, multi_edges, edge_labels, ports, compound, clusters
preview images/layered_layout.png
// general spacings
supports org.eclipse.elk.spacing.commentComment
supports org.eclipse.elk.spacing.commentNode
supports org.eclipse.elk.spacing.componentComponent
supports org.eclipse.elk.spacing.edgeEdge
supports org.eclipse.elk.spacing.edgeLabel
supports org.eclipse.elk.spacing.edgeNode
supports org.eclipse.elk.spacing.labelLabel
supports org.eclipse.elk.spacing.labelPortHorizontal
supports org.eclipse.elk.spacing.labelPortVertical
supports org.eclipse.elk.spacing.labelNode
supports org.eclipse.elk.spacing.nodeNode
supports org.eclipse.elk.spacing.nodeSelfLoop
supports org.eclipse.elk.spacing.portPort
supports org.eclipse.elk.spacing.individual
documentation "Most parts of the algorithm do not support this yet."
// layer-based spacings
supports org.eclipse.elk.alg.layered.spacing.baseValue
supports org.eclipse.elk.alg.layered.spacing.edgeEdgeBetweenLayers
supports org.eclipse.elk.alg.layered.spacing.edgeNodeBetweenLayers
supports org.eclipse.elk.alg.layered.spacing.nodeNodeBetweenLayers
// layer-based priorities
supports org.eclipse.elk.priority = 0
documentation "Used by the 'simple row graph placer' to decide which connected components to place first.
A component's priority is the sum of the node priorities,
and components with higher priorities will be placed
before components with lower priorities."
supports priority.direction
supports priority.shortness
documentation "Currently only supported by the network simplex layerer."
supports priority.straightness
// wrapping related
supports wrapping.strategy
supports wrapping.additionalEdgeSpacing
supports wrapping.correctionFactor
supports wrapping.cutting.strategy
supports wrapping.cutting.cuts
supports wrapping.cutting.msd.freedom
supports wrapping.validify.strategy
supports wrapping.validify.forbiddenIndices
supports wrapping.multiEdge.improveCuts
supports wrapping.multiEdge.distancePenalty
supports wrapping.multiEdge.improveWrappedEdges
// flexible nodes during node placement
supports nodePlacement.networkSimplex.nodeFlexibility
supports nodePlacement.networkSimplex.nodeFlexibility.^default
// splines
supports edgeRouting.splines.mode
supports edgeRouting.splines.sloppy.layerSpacingFactor
// topdown layout
supports org.eclipse.elk.topdownLayout
supports org.eclipse.elk.topdown.scaleFactor
supports org.eclipse.elk.topdown.hierarchicalNodeWidth
supports org.eclipse.elk.topdown.hierarchicalNodeAspectRatio
supports org.eclipse.elk.topdown.nodeType = TopdownNodeTypes.HIERARCHICAL_NODE
// other options
supports org.eclipse.elk.padding = new ElkPadding(12)
supports org.eclipse.elk.edgeRouting = EdgeRouting.ORTHOGONAL
supports org.eclipse.elk.port.borderOffset = 0
supports org.eclipse.elk.randomSeed = 1
supports org.eclipse.elk.aspectRatio = 1.6f
supports org.eclipse.elk.noLayout
supports org.eclipse.elk.portConstraints
supports org.eclipse.elk.port.side
supports org.eclipse.elk.debugMode
supports org.eclipse.elk.alignment
supports org.eclipse.elk.hierarchyHandling
supports org.eclipse.elk.separateConnectedComponents = true
supports org.eclipse.elk.insideSelfLoops.activate
supports org.eclipse.elk.insideSelfLoops.yo
supports org.eclipse.elk.nodeSize.constraints
supports org.eclipse.elk.nodeSize.options
supports org.eclipse.elk.nodeSize.fixedGraphSize
supports org.eclipse.elk.direction = Direction.UNDEFINED
supports org.eclipse.elk.nodeLabels.placement
supports org.eclipse.elk.nodeLabels.padding
supports org.eclipse.elk.portLabels.placement
supports org.eclipse.elk.portLabels.nextToPortIfPossible
supports org.eclipse.elk.portLabels.treatAsGroup
supports org.eclipse.elk.portAlignment.^default = PortAlignment.JUSTIFIED
supports org.eclipse.elk.portAlignment.north
supports org.eclipse.elk.portAlignment.south
supports org.eclipse.elk.portAlignment.west
supports org.eclipse.elk.portAlignment.east
supports unnecessaryBendpoints
supports org.eclipse.elk.alg.layered.layering.strategy
supports org.eclipse.elk.alg.layered.layering.nodePromotion.strategy
supports thoroughness
supports org.eclipse.elk.alg.layered.layering.layerConstraint
supports org.eclipse.elk.alg.layered.cycleBreaking.strategy
supports org.eclipse.elk.alg.layered.crossingMinimization.strategy
supports org.eclipse.elk.alg.layered.crossingMinimization.forceNodeModelOrder
supports org.eclipse.elk.alg.layered.crossingMinimization.greedySwitch.activationThreshold
supports org.eclipse.elk.alg.layered.crossingMinimization.greedySwitch.type
supports org.eclipse.elk.alg.layered.crossingMinimization.greedySwitchHierarchical.type
supports org.eclipse.elk.alg.layered.crossingMinimization.semiInteractive
supports mergeEdges
supports mergeHierarchyEdges
supports interactiveReferencePoint
supports org.eclipse.elk.alg.layered.nodePlacement.strategy
supports org.eclipse.elk.alg.layered.nodePlacement.bk.fixedAlignment
supports feedbackEdges
supports org.eclipse.elk.alg.layered.nodePlacement.linearSegments.deflectionDampening
supports org.eclipse.elk.alg.layered.edgeRouting.selfLoopDistribution
supports org.eclipse.elk.alg.layered.edgeRouting.selfLoopOrdering
supports org.eclipse.elk.contentAlignment
supports org.eclipse.elk.alg.layered.nodePlacement.bk.edgeStraightening
supports org.eclipse.elk.alg.layered.compaction.postCompaction.strategy
supports org.eclipse.elk.alg.layered.compaction.postCompaction.constraints
supports org.eclipse.elk.alg.layered.compaction.connectedComponents
supports org.eclipse.elk.alg.layered.highDegreeNodes.treatment
supports org.eclipse.elk.alg.layered.highDegreeNodes.threshold
supports org.eclipse.elk.alg.layered.highDegreeNodes.treeHeight
supports org.eclipse.elk.nodeSize.minimum
supports org.eclipse.elk.junctionPoints
supports org.eclipse.elk.edge.thickness
supports org.eclipse.elk.edgeLabels.placement
supports org.eclipse.elk.edgeLabels.inline
supports org.eclipse.elk.alg.layered.crossingMinimization.hierarchicalSweepiness
supports org.eclipse.elk.port.index
supports org.eclipse.elk.commentBox
supports org.eclipse.elk.hypernode
supports org.eclipse.elk.port.anchor
supports org.eclipse.elk.partitioning.activate
supports org.eclipse.elk.partitioning.partition
supports org.eclipse.elk.alg.layered.layering.minWidth.upperBoundOnWidth
supports org.eclipse.elk.alg.layered.layering.minWidth.upperLayerEstimationScalingFactor
supports org.eclipse.elk.position
supports allowNonFlowPortsToSwitchSides
supports org.eclipse.elk.alg.layered.layering.nodePromotion.maxIterations
supports edgeLabels.sideSelection
supports edgeLabels.centerLabelPlacementStrategy
supports org.eclipse.elk.margins
supports layering.coffmanGraham.layerBound
supports nodePlacement.favorStraightEdges
supports org.eclipse.elk.spacing.portsSurrounding
supports directionCongruency
supports portSortingStrategy
supports edgeRouting.polyline.slopedEdgeZoneWidth
supports crossingMinimization.inLayerPredOf
supports crossingMinimization.inLayerSuccOf
supports layering.layerChoiceConstraint
supports crossingMinimization.positionChoiceConstraint
supports org.eclipse.elk.interactiveLayout
supports layering.layerId
supports crossingMinimization.positionId
supports considerModelOrder.strategy
supports considerModelOrder.longEdgeStrategy
supports considerModelOrder.crossingCounterNodeInfluence
supports considerModelOrder.crossingCounterPortInfluence
supports considerModelOrder.noModelOrder
supports considerModelOrder.components
supports considerModelOrder.portModelOrder
supports generatePositionAndLayerIds
}
/* ------------------------
* phase 1
* ------------------------*/
group cycleBreaking {
option strategy: CycleBreakingStrategy {
label "Cycle Breaking Strategy"
description
"Strategy for cycle breaking. Cycle breaking looks for cycles in the graph and determines
which edges to reverse to break the cycles. Reversed edges will end up pointing to the
opposite direction of regular edges (that is, reversed edges will point left if edges
usually point right)."
default = CycleBreakingStrategy.GREEDY
targets parents
}
}
/* ------------------------
* phase 2
* ------------------------*/
group layering {
option strategy: LayeringStrategy {
label "Node Layering Strategy"
description
"Strategy for node layering."
default = LayeringStrategy.NETWORK_SIMPLEX
targets parents
}
advanced option layerConstraint: LayerConstraint {
label "Layer Constraint"
description
"Determines a constraint on the placement of the node regarding the layering."
default = LayerConstraint.NONE
targets nodes
}
advanced option layerChoiceConstraint: Integer {
label "Layer Choice Constraint"
description
"Allows to set a constraint regarding the layer placement of a node.
Let i be the value of teh constraint. Assumed the drawing has n layers and i < n.
If set to i, it expresses that the node should be placed in i-th layer.
Should i>=n be true then the node is placed in the last layer of the drawing.
Note that this option is not part of any of ELK Layered's default configurations
but is only evaluated as part of the `InteractiveLayeredGraphVisitor`,
which must be applied manually or used via the `DiagramLayoutEngine."
default = null
lowerBound = -1
targets nodes
}
output option layerId: int {
label "Layer ID"
description
"Layer identifier that was calculated by ELK Layered for a node.
This is only generated if interactiveLayot or generatePositionAndLayerIds is set."
default = -1
lowerBound = -1
targets nodes
}
group minWidth {
advanced option upperBoundOnWidth: int {
label "Upper Bound On Width [MinWidth Layerer]"
description
"Defines a loose upper bound on the width of the MinWidth layerer.
If set to '-1' multiple values are tested and the best result is selected."
default = 4
lowerBound = -1
targets parents
requires org.eclipse.elk.alg.layered.layering.strategy == LayeringStrategy.MIN_WIDTH
}
advanced option upperLayerEstimationScalingFactor: int {
label "Upper Layer Estimation Scaling Factor [MinWidth Layerer]"
description
"Multiplied with Upper Bound On Width for defining an upper bound on the width of layers which
haven't been determined yet, but whose maximum width had been (roughly) estimated by the MinWidth
algorithm. Compensates for too high estimations.
If set to '-1' multiple values are tested and the best result is selected."
default = 2
lowerBound = -1
targets parents
requires org.eclipse.elk.alg.layered.layering.strategy == LayeringStrategy.MIN_WIDTH
}
}
group nodePromotion {
advanced option strategy: NodePromotionStrategy {
label "Node Promotion Strategy"
description
"Reduces number of dummy nodes after layering phase (if possible)."
default = NodePromotionStrategy.NONE
targets parents
}
advanced option maxIterations: int {
label "Max Node Promotion Iterations"
description
"Limits the number of iterations for node promotion."
default = 0
lowerBound = 0
targets parents
requires org.eclipse.elk.alg.layered.layering.nodePromotion.strategy
}
}
group coffmanGraham {
advanced option layerBound: int {
label "Layer Bound"
description
"The maximum number of nodes allowed per layer."
default = Integer.MAX_VALUE
targets parents
requires org.eclipse.elk.alg.layered.layering.strategy == LayeringStrategy.COFFMAN_GRAHAM
}
}
}
/* ------------------------
* phase 3
* ------------------------*/
group crossingMinimization {
option strategy: CrossingMinimizationStrategy {
label "Crossing Minimization Strategy"
description
"Strategy for crossing minimization."
default = CrossingMinimizationStrategy.LAYER_SWEEP
targets parents
}
option forceNodeModelOrder: boolean {
label "Force Node Model Order"
description
"The node order given by the model does not change to produce a better layout. E.g. if node A
is before node B in the model this is not changed during crossing minimization. This assumes that the
node model order is already respected before crossing minimization. This can be achieved by setting
considerModelOrder.strategy to NODES_AND_EDGES."
default = false
targets parents
}
advanced option hierarchicalSweepiness: double {
label "Hierarchical Sweepiness"
description
"How likely it is to use cross-hierarchy (1) vs bottom-up (-1)."
default = 0.1
targets parents
requires org.eclipse.elk.hierarchyHandling == HierarchyHandling.INCLUDE_CHILDREN
}
group greedySwitch {
advanced option activationThreshold: int {
label "Greedy Switch Activation Threshold"
description
"By default it is decided automatically if the greedy switch is activated or not.
The decision is based on whether the size of the input graph (without dummy nodes)
is smaller than the value of this option. A '0' enforces the activation."
default = 40
targets parents
lowerBound = 0
}
advanced option type: GreedySwitchType {
label "Greedy Switch Crossing Minimization"
description
"Greedy Switch strategy for crossing minimization. The greedy switch heuristic is executed
after the regular crossing minimization as a post-processor.
Note that if 'hierarchyHandling' is set to 'INCLUDE_CHILDREN', the 'greedySwitchHierarchical.type'
option must be used."
default = GreedySwitchType.TWO_SIDED
targets parents
requires crossingMinimization.strategy == CrossingMinimizationStrategy.LAYER_SWEEP
}
}
group greedySwitchHierarchical {
advanced option type: GreedySwitchType {
label "Greedy Switch Crossing Minimization (hierarchical)"
description
"Activates the greedy switch heuristic in case hierarchical layout is used.
The differences to the non-hierarchical case (see 'greedySwitch.type') are:
1) greedy switch is inactive by default,
3) only the option value set on the node at which hierarchical layout starts is relevant, and
2) if it's activated by the user, it properly addresses hierarchy-crossing edges."
default = GreedySwitchType.OFF
targets parents
requires crossingMinimization.strategy == CrossingMinimizationStrategy.LAYER_SWEEP
requires org.eclipse.elk.hierarchyHandling == HierarchyHandling.INCLUDE_CHILDREN
}
}
advanced option semiInteractive: boolean {
label "Semi-Interactive Crossing Minimization"
description
"Preserves the order of nodes within a layer but still minimizes crossings between edges connecting
long edge dummies. Derives the desired order from positions specified by the 'org.eclipse.elk.position'
layout option. Requires a crossing minimization strategy that is able to process 'in-layer' constraints."
default = false
targets parents
requires crossingMinimization.strategy == CrossingMinimizationStrategy.LAYER_SWEEP
}
option inLayerPredOf: String {
label "In Layer Predecessor of"
description
"Allows to set a constraint which specifies of which node
the current node is the predecessor.
If set to 's' then the node is the predecessor of 's' and is in the same layer"
default = null
targets nodes
}
advanced option inLayerSuccOf: String {
label "In Layer Successor of"
description
"Allows to set a constraint which specifies of which node
the current node is the successor.
If set to 's' then the node is the successor of 's' and is in the same layer"
default = null
targets nodes
}
advanced option positionChoiceConstraint: Integer {
label "Position Choice Constraint"
description
"Allows to set a constraint regarding the position placement of a node in a layer.
Assumed the layer in which the node placed includes n other nodes and i < n.
If set to i, it expresses that the node should be placed at the i-th position.
Should i>=n be true then the node is placed at the last position in the layer.
Note that this option is not part of any of ELK Layered's default configurations
but is only evaluated as part of the `InteractiveLayeredGraphVisitor`,
which must be applied manually or used via the `DiagramLayoutEngine. "
default = null
lowerBound = -1
targets nodes
}
output option positionId: int {
label "Position ID"
description
"Position within a layer that was determined by ELK Layered for a node.
This is only generated if interactiveLayot or generatePositionAndLayerIds is set."
default = -1
lowerBound = -1
targets nodes
}
}
/* ------------------------
* phase 4
* ------------------------*/
group nodePlacement {
option strategy: NodePlacementStrategy {
label "Node Placement Strategy"
description
"Strategy for node placement."
default = NodePlacementStrategy.BRANDES_KOEPF
targets parents
}
option favorStraightEdges: boolean {
label "Favor Straight Edges Over Balancing"
description
"Favor straight edges over a balanced node placement.
The default behavior is determined automatically based on the used 'edgeRouting'.
For an orthogonal style it is set to true, for all other styles to false."
// no default, see description
targets parents
requires strategy == NodePlacementStrategy.NETWORK_SIMPLEX
requires strategy == NodePlacementStrategy.BRANDES_KOEPF
}
group bk {
advanced option edgeStraightening: EdgeStraighteningStrategy {
label "BK Edge Straightening"
description
"Specifies whether the Brandes Koepf node placer tries to increase the number of straight edges
at the expense of diagram size.
There is a subtle difference to the 'favorStraightEdges' option, which decides whether
a balanced placement of the nodes is desired, or not. In bk terms this means combining the four
alignments into a single balanced one, or not. This option on the other hand tries to straighten
additional edges during the creation of each of the four alignments."
default = EdgeStraighteningStrategy.IMPROVE_STRAIGHTNESS
targets parents
requires org.eclipse.elk.alg.layered.nodePlacement.strategy == NodePlacementStrategy.BRANDES_KOEPF
}
advanced option fixedAlignment: FixedAlignment {
label "BK Fixed Alignment"
description
"Tells the BK node placer to use a certain alignment (out of its four) instead of the
one producing the smallest height, or the combination of all four."
default = FixedAlignment.NONE
targets parents
requires org.eclipse.elk.alg.layered.nodePlacement.strategy == NodePlacementStrategy.BRANDES_KOEPF
}
}
group linearSegments {
advanced option deflectionDampening: double {
label "Linear Segments Deflection Dampening"
description
"Dampens the movement of nodes to keep the diagram from getting too large."
default = 0.3
lowerBound = ExclusiveBounds.greaterThan(0)
targets parents
requires org.eclipse.elk.alg.layered.nodePlacement.strategy == NodePlacementStrategy.LINEAR_SEGMENTS
}
}
group networkSimplex {
advanced option nodeFlexibility: NodeFlexibility {
label "Node Flexibility"
description
"Aims at shorter and straighter edges. Two configurations are possible:
(a) allow ports to move freely on the side they are assigned to (the order is always defined beforehand),
(b) additionally allow to enlarge a node wherever it helps.
If this option is not configured for a node, the 'nodeFlexibility.default' value is used,
which is specified for the node's parent."
targets nodes
requires nodePlacement.strategy == NodePlacementStrategy.NETWORK_SIMPLEX
}
group nodeFlexibility {
advanced option ^default: NodeFlexibility {
label "Node Flexibility Default"
description
"Default value of the 'nodeFlexibility' option for the children of a hierarchical node."
default = NodeFlexibility.NONE
targets parents
requires nodePlacement.strategy == NodePlacementStrategy.NETWORK_SIMPLEX
}
}
}
}
/* ------------------------
* phase 5
* ------------------------*/
group edgeRouting {
group splines {
option mode: SplineRoutingMode {
label "Spline Routing Mode"
description
"Specifies the way control points are assembled for each individual edge.
CONSERVATIVE ensures that edges are properly routed around the nodes
but feels rather orthogonal at times.
SLOPPY uses fewer control points to obtain curvier edge routes but may result in
edges overlapping nodes. "
default = SplineRoutingMode.SLOPPY
targets parents
requires org.eclipse.elk.edgeRouting == EdgeRouting.SPLINES
}
group sloppy {
option layerSpacingFactor: double {
label "Sloppy Spline Layer Spacing Factor"
description
"Spacing factor for routing area between layers when using sloppy spline routing."
default = 0.2
targets parents
requires org.eclipse.elk.edgeRouting == EdgeRouting.SPLINES // TODO this should be an AND
requires splines.mode == SplineRoutingMode.SLOPPY
}
}
}
group polyline {
advanced option slopedEdgeZoneWidth: double {
label "Sloped Edge Zone Width"
description
"Width of the strip to the left and to the right of each layer where the polyline edge router
is allowed to refrain from ensuring that edges are routed horizontally. This prevents awkward
bend points for nodes that extent almost to the edge of their layer."
documentation "@slopedEdgeZoneWidth.md"
default = 2.0
targets parents
requires org.eclipse.elk.edgeRouting == EdgeRouting.POLYLINE
}
}
option selfLoopDistribution: SelfLoopDistributionStrategy {
label "Self-Loop Distribution"
description
"Alter the distribution of the loops around the node. It only takes effect for PortConstraints.FREE."
default = SelfLoopDistributionStrategy.NORTH
targets nodes
}
option selfLoopOrdering: SelfLoopOrderingStrategy {
label "Self-Loop Ordering"
description
"Alter the ordering of the loops they can either be stacked or sequenced.
It only takes effect for PortConstraints.FREE."
default = SelfLoopOrderingStrategy.STACKED
targets nodes
}
}
/* ------------------------
* spacing
* ------------------------*/
group spacing {
option baseValue: double {
label "Spacing Base Value"
description
"An optional base value for all other layout options of the 'spacing' group. It can be used to conveniently
alter the overall 'spaciousness' of the drawing. Whenever an explicit value is set for the other layout
options, this base value will have no effect. The base value is not inherited, i.e. it must be set for
each hierarchical node."
targets parents
lowerBound = 0.0
}
option edgeNodeBetweenLayers: double {
label "Edge Node Between Layers Spacing"
description
"The spacing to be preserved between nodes and edges that are routed next to the node's layer.
For the spacing between nodes and edges that cross the node's layer 'spacing.edgeNode' is used."
default = 10
lowerBound = 0.0
targets parents
}
option edgeEdgeBetweenLayers: double {
label "Edge Edge Between Layer Spacing"
description
"Spacing to be preserved between pairs of edges that are routed between the same pair of layers.
Note that 'spacing.edgeEdge' is used for the spacing between pairs of edges crossing the same layer."
default = 10
lowerBound = 0.0
targets parents
}
option nodeNodeBetweenLayers: double {
label "Node Node Between Layers Spacing"
description
"The spacing to be preserved between any pair of nodes of two adjacent layers.
Note that 'spacing.nodeNode' is used for the spacing between nodes within the layer itself."
default = 20
lowerBound = 0.0
targets parents
}
}
/* ------------------------
* priority
* ------------------------*/
group priority {
advanced option direction: int {
label "Direction Priority"
description
"Defines how important it is to have a certain edge point into the direction of the overall layout.
This option is evaluated during the cycle breaking phase."
default = 0
lowerBound = 0
targets edges
}
advanced option shortness: int {
label "Shortness Priority"
description
"Defines how important it is to keep an edge as short as possible.
This option is evaluated during the layering phase."
default = 0
lowerBound = 0
targets edges
}
advanced option straightness: int {
label "Straightness Priority"
description
"Defines how important it is to keep an edge straight, i.e. aligned with one of the two axes.
This option is evaluated during node placement."
default = 0
lowerBound = 0
targets edges
}
}
/* ------------------------
* compaction
* ------------------------*/
group compaction {
group postCompaction {
advanced option strategy: GraphCompactionStrategy {
label "Post Compaction Strategy"
description
"Specifies whether and how post-process compaction is applied."
default = GraphCompactionStrategy.NONE
targets parents
}
advanced option constraints: ConstraintCalculationStrategy {
label "Post Compaction Constraint Calculation"
description
"Specifies whether and how post-process compaction is applied."
default = ConstraintCalculationStrategy.SCANLINE
targets parents
}
}
advanced option connectedComponents: boolean {
label "Connected Components Compaction"
description
"Tries to further compact components (disconnected sub-graphs)."
default = false
targets parents
requires org.eclipse.elk.separateConnectedComponents == true
}
}
/* ------------------------
* high degree nodes
* ------------------------*/
group highDegreeNodes {
advanced option treatment: boolean {
label "High Degree Node Treatment"
description
"Makes room around high degree nodes to place leafs and trees."
default = false
targets parents
}
advanced option threshold: int {
label "High Degree Node Threshold"
description
"Whether a node is considered to have a high degree."
default = 16
lowerBound = 0
targets parents
requires org.eclipse.elk.alg.layered.highDegreeNodes.treatment == true
}
advanced option treeHeight: int {
label "High Degree Node Maximum Tree Height"
description
"Maximum height of a subtree connected to a high degree node to be moved to separate layers."
default = 5
lowerBound = 0
targets parents
requires org.eclipse.elk.alg.layered.highDegreeNodes.treatment == true
}
}
/* ------------------------
* wrapping
* ------------------------*/
group wrapping {
advanced option strategy: WrappingStrategy {
label "Graph Wrapping Strategy"
description
"For certain graphs and certain prescribed drawing areas it may be desirable to
split the laid out graph into chunks that are placed side by side.
The edges that connect different chunks are 'wrapped' around from the end of
one chunk to the start of the other chunk.
The points between the chunks are referred to as 'cuts'."
targets parents
default = WrappingStrategy.OFF
}
advanced option additionalEdgeSpacing: double {
label "Additional Wrapped Edges Spacing"
description
"To visually separate edges that are wrapped from regularly routed edges an additional spacing value
can be specified in form of this layout option. The spacing is added to the regular edgeNode spacing."
default = 10
targets parents
requires wrapping.strategy == WrappingStrategy.SINGLE_EDGE
requires wrapping.strategy == WrappingStrategy.MULTI_EDGE
}
advanced option correctionFactor: double {
label "Correction Factor for Wrapping"
description
"At times and for certain types of graphs the executed wrapping may produce results that
are consistently biased in the same fashion: either wrapping to often or to rarely.
This factor can be used to correct the bias. Internally, it is simply multiplied with
the 'aspect ratio' layout option."
default = 1.0
targets parents
requires wrapping.strategy == WrappingStrategy.SINGLE_EDGE
requires wrapping.strategy == WrappingStrategy.MULTI_EDGE
}
group cutting {
advanced option strategy: CuttingStrategy {
label "Cutting Strategy"
description
"The strategy by which the layer indexes are determined at which the layering crumbles into chunks."
default = CuttingStrategy.MSD
targets parents
requires wrapping.strategy == WrappingStrategy.SINGLE_EDGE
requires wrapping.strategy == WrappingStrategy.MULTI_EDGE
}
advanced option cuts: List {
label "Manually Specified Cuts"
description
"Allows the user to specify her own cuts for a certain graph."
targets parents
requires wrapping.cutting.strategy == CuttingStrategy.MANUAL
}
group msd {
advanced option freedom: Integer {
label "MSD Freedom"
description
"The MSD cutting strategy starts with an initial guess on the number of chunks the graph
should be split into. The freedom specifies how much the strategy may deviate from this guess.
E.g. if an initial number of 3 is computed, a freedom of 1 allows 2, 3, and 4 cuts."
default = 1
lowerBound = 0
targets parents
requires wrapping.cutting.strategy == CuttingStrategy.MSD
}
}
}
group validify {
advanced option strategy: ValidifyStrategy {
label "Validification Strategy"
description
"When wrapping graphs, one can specify indices that are not allowed as split points.
The validification strategy makes sure every computed split point is allowed."
default = ValidifyStrategy.GREEDY
targets parents
requires wrapping.strategy == WrappingStrategy.SINGLE_EDGE
requires wrapping.strategy == WrappingStrategy.MULTI_EDGE
}
advanced option forbiddenIndices: List {
label "Valid Indices for Wrapping"
targets parents
requires wrapping.strategy == WrappingStrategy.SINGLE_EDGE
requires wrapping.strategy == WrappingStrategy.MULTI_EDGE
}
}
group singleEdge {
}
group multiEdge {
advanced option improveCuts: boolean {
label "Improve Cuts"
description
"For general graphs it is important that not too many edges wrap backwards.
Thus a compromise between evenly-distributed cuts and the total number of cut edges is sought."
default = true
targets parents
requires strategy == WrappingStrategy.MULTI_EDGE
}
advanced option distancePenalty: double {
label "Distance Penalty When Improving Cuts "
default = 2.0
targets parents
requires strategy == WrappingStrategy.MULTI_EDGE
requires improveCuts == true
}
advanced option improveWrappedEdges: boolean {
label "Improve Wrapped Edges"
description
"The initial wrapping is performed in a very simple way. As a consequence, edges that wrap from
one chunk to another may be unnecessarily long. Activating this option tries to shorten such edges."
default = true
targets parents
requires strategy == WrappingStrategy.MULTI_EDGE
}
}
}
/* ------------------------
* edgeLabels
* ------------------------*/
group edgeLabels {
option sideSelection: EdgeLabelSideSelection {
label "Edge Label Side Selection"
description
"Method to decide on edge label sides."
default = EdgeLabelSideSelection.SMART_DOWN
targets parents
}
advanced option centerLabelPlacementStrategy: CenterEdgeLabelPlacementStrategy {
label "Edge Center Label Placement Strategy"
description
"Determines in which layer center labels of long edges should be placed."
default = CenterEdgeLabelPlacementStrategy.MEDIAN_LAYER
targets parents, labels
}
}
/* ------------------------
* miscellaneous
* ------------------------*/
group considerModelOrder {
option strategy: OrderingStrategy {
label "Consider Model Order"
description
"Preserves the order of nodes and edges in the model file if this does not lead to additional edge
crossings. Depending on the strategy this is not always possible since the node and edge order might be
conflicting."
default = OrderingStrategy.NONE
targets parents
}
option portModelOrder: boolean {
label "Consider Port Order"
description
"If disabled the port order of output ports is derived from the edge order and input ports are ordered by
their incoming connections. If enabled all ports are ordered by the port model order."
default = false
targets parents
}
option noModelOrder: boolean {
label "No Model Order"
description
"Set on a node to not set a model order for this node even though it is a real node."
default = false
targets nodes
}
option components: ComponentOrderingStrategy {
label "Consider Model Order for Components"
description
"If set to NONE the usual ordering strategy (by cumulative node priority and size of nodes) is used.
INSIDE_PORT_SIDES orders the components with external ports only inside the groups with the same port side.
FORCE_MODEL_ORDER enforces the mode order on components. This option might produce bad alignments and sub
optimal drawings in terms of used area since the ordering should be respected."
targets parents
default = ComponentOrderingStrategy.NONE
requires org.eclipse.elk.separateConnectedComponents
}
option longEdgeStrategy: LongEdgeOrderingStrategy {
label "Long Edge Ordering Strategy"
description
"Indicates whether long edges are sorted under, over, or equal to nodes that have no connection to a
previous layer in a left-to-right or right-to-left layout. Under and over changes to right and left in a
vertical layout."
default = LongEdgeOrderingStrategy.DUMMY_NODE_OVER
targets parents
}
option crossingCounterNodeInfluence: double {
label "Crossing Counter Node Order Influence"
description
"Indicates with what percentage (1 for 100%) violations of the node model order are weighted against the
crossings e.g. a value of 0.5 means two model order violations are as important as on edge crossing.
This allows some edge crossings in favor of preserving the model order. It is advised to set this value to
a very small positive value (e.g. 0.001) to have minimal crossing and a optimal node order. Defaults to no
influence (0)."
default = 0
targets parents
requires org.eclipse.elk.alg.layered.considerModelOrder.strategy
}
option crossingCounterPortInfluence: double {
label "Crossing Counter Port Order Influence"
description
"Indicates with what percentage (1 for 100%) violations of the port model order are weighted against the
crossings e.g. a value of 0.5 means two model order violations are as important as on edge crossing.
This allows some edge crossings in favor of preserving the model order. It is advised to set this value to
a very small positive value (e.g. 0.001) to have minimal crossing and a optimal port order. Defaults to no
influence (0)."
default = 0
targets parents
requires org.eclipse.elk.alg.layered.considerModelOrder.strategy
}
}
advanced option directionCongruency: DirectionCongruency {
label "Direction Congruency"
description
"Specifies how drawings of the same graph with different layout directions compare to each other:
either a natural reading direction is preserved or the drawings are rotated versions of each other."
default = DirectionCongruency.READING_DIRECTION
targets parents
documentation "@transformation.md"
}
advanced option feedbackEdges: boolean {
label "Feedback Edges"
description
"Whether feedback edges should be highlighted by routing around the nodes."
default = false
targets parents
}
advanced option interactiveReferencePoint: InteractiveReferencePoint {
label "Interactive Reference Point"
description
"Determines which point of a node is considered by interactive layout phases."
default = InteractiveReferencePoint.CENTER
targets parents
requires org.eclipse.elk.alg.layered.cycleBreaking.strategy == CycleBreakingStrategy.INTERACTIVE
requires org.eclipse.elk.alg.layered.crossingMinimization.strategy == CrossingMinimizationStrategy.INTERACTIVE
}
advanced option mergeEdges: boolean {
label "Merge Edges"
description
"Edges that have no ports are merged so they touch the connected nodes at the same points.
When this option is disabled, one port is created for each edge directly connected to a
node. When it is enabled, all such incoming edges share an input port, and all outgoing
edges share an output port."
default = false
targets parents
}
advanced option mergeHierarchyEdges: boolean {
label "Merge Hierarchy-Crossing Edges"
description
"If hierarchical layout is active, hierarchy-crossing edges use as few hierarchical ports
as possible. They are broken by the algorithm, with hierarchical ports inserted as
required. Usually, one such port is created for each edge at each hierarchy crossing point.
With this option set to true, we try to create as few hierarchical ports as possible in
the process. In particular, all edges that form a hyperedge can share a port."
default = true
targets parents
}
advanced option allowNonFlowPortsToSwitchSides: boolean {
label "Allow Non-Flow Ports To Switch Sides"
description
"Specifies whether non-flow ports may switch sides if their node's port constraints are either FIXED_SIDE
or FIXED_ORDER. A non-flow port is a port on a side that is not part of the currently configured layout flow.
For instance, given a left-to-right layout direction, north and south ports would be considered non-flow ports.
Further note that the underlying criterium whether to switch sides or not solely relies on the
minimization of edge crossings. Hence, edge length and other aesthetics criteria are not addressed."
default = false
targets ports
legacyIds org.eclipse.elk.layered.northOrSouthPort
}
advanced option portSortingStrategy: PortSortingStrategy {
label "Port Sorting Strategy"
description
"Only relevant for nodes with FIXED_SIDE port constraints. Determines the way a node's ports are
distributed on the sides of a node if their order is not prescribed. The option is set on parent nodes."
default = PortSortingStrategy.INPUT_ORDER
targets parents
}
advanced option thoroughness: int {
label "Thoroughness"
description
"How much effort should be spent to produce a nice layout."
default = 7
lowerBound = 1
targets parents
}
advanced option unnecessaryBendpoints: boolean {
label "Add Unnecessary Bendpoints"
description
"Adds bend points even if an edge does not change direction. If true, each long edge dummy
will contribute a bend point to its edges and hierarchy-crossing edges will always get a
bend point where they cross hierarchy boundaries. By default, bend points are only added
where an edge changes direction."
default = false
targets parents
}
advanced option generatePositionAndLayerIds: boolean {
label "Generate Position and Layer IDs"
description
"If enabled position id and layer id are generated, which are usually only used internally
when setting the interactiveLayout option. This option should be specified on the root node."
default = false
targets parents
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy