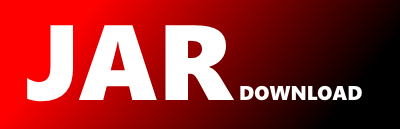
javarequirementstracer.RequirementsScanner Maven / Gradle / Ivy
/*
* Copyright 2008-2009 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package javarequirementstracer;
import java.lang.reflect.Constructor;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.TreeMap;
import java.util.TreeSet;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.core.type.filter.AnnotationTypeFilter;
import org.springframework.util.ClassUtils;
/**
* Implementation of {@link JavaRequirementsTracer#scanRequirements()}.
*
* @author Ronald Koster
*/
final class RequirementsScanner extends AbstractScanner {
static final String METHOD_SEPARATOR = "#";
RequirementsScanner(TraceProperties properties) {
super(properties);
}
SortedMap> run() {
final SortedMap> codeNamesTo = new TreeMap>();
for (Class> cl : getTypes(getProperties().getIncludePackageNames())) {
if (exclude(cl)) {
continue;
}
// NB. Requirements annotations cannot be inherited.
if (cl.isAnnotationPresent(Requirements.class)) {
Requirements reqs = cl.getAnnotation(Requirements.class);
addLabels(codeNamesTo, cl, reqs);
}
for (Constructor> con : cl.getDeclaredConstructors()) {
if (con.isAnnotationPresent(Requirements.class)) {
Requirements reqs = con.getAnnotation(Requirements.class);
addLabels(codeNamesTo, con, reqs);
}
}
for (Method meth : cl.getDeclaredMethods()) {
if (meth.isAnnotationPresent(Requirements.class)) {
Requirements reqs = meth.getAnnotation(Requirements.class);
addLabels(codeNamesTo, meth, reqs);
}
}
}
return codeNamesTo;
}
private Set> getTypes(final Set packageNames) {
final Set> list = new HashSet>();
// Scan for all classess in the classpath with the Requirements annotation.
final ClassPathScanner scanner = new ClassPathScanner(false);
setResourceLoader(scanner);
scanner.addIncludeFilter(new AnnotationTypeFilter(Requirements.class));
// Search the given packages.
for (String basePackageName : packageNames) {
final Set components = scanner.findCandidateComponents(basePackageName);
for (BeanDefinition component : components) {
String className = component.getBeanClassName();
list.add(ClassUtils.resolveClassName(className, getClassLoader()));
}
}
return list;
}
private void addLabels(final Map> codeNamesToLabels, final T type,
final Requirements reqs) {
final Set labels = getLabels(codeNamesToLabels, type);
labels.addAll(Arrays.asList(reqs.value()));
}
private Set getLabels(final Map> codeNamesToLabels, final T type) {
String typeName = getProperties().getShortTypeName(getTypeName(type));
SortedSet set = codeNamesToLabels.get(typeName);
if (set == null) {
set = new TreeSet();
codeNamesToLabels.put(typeName, set);
}
return set;
}
private String getTypeName(final Object type) {
if (type instanceof Class) {
Class> cl = (Class>) type;
return cl.getName();
} else if (type instanceof Constructor) {
Constructor> con = (Constructor>) type;
String name = con.getName();
int index = name.lastIndexOf('.');
return name + METHOD_SEPARATOR + name.substring(index + 1);
} else if (type instanceof Method) {
Method meth = (Method) type;
return meth.getDeclaringClass().getName() + METHOD_SEPARATOR + meth.getName();
}
throw new IllegalArgumentException("type=" + type);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy