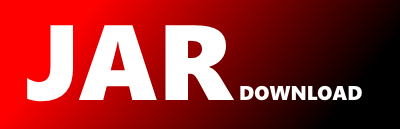
javarequirementstracer.TraceProperties Maven / Gradle / Ivy
/*
* Copyright 2008-2009 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package javarequirementstracer;
import java.io.File;
import java.util.Arrays;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.TreeMap;
import java.util.TreeSet;
/**
* Implementation of {@link JavaRequirementsTracer#init()}.
*
* @author Ronald Koster
*/
final class TraceProperties {
private static final Logger LOGGER = Logger.getInstance(TraceProperties.class);
static final String SEPARATOR = ",\\s*";
private static final String DEFAULT_EXPECTED_LABELS_FILENAME = "traceability_labels.properties";
private static final String DEFAULT_REPORT_FILENAME = "../../../target/traceability.html";
private static final String EXPECTED_LABELS_FILENAME_KEY = "expected.labels.filename";
private static final String REPORT_FILENAME_KEY = "report.filename";
private static final String PROJECT_NAME_KEY = "project.name";
private static final String ROOT_PACKAGE_NAME_KEY = "root.package.name";
private static final String INCLUDE_PACKAGE_NAMES_KEY = "include.package.names";
private static final String EXCLUDE_PACKAGE_NAMES_KEY = "exclude.package.names";
private static final String EXCLUDE_TYPE_NAMES_KEY = "exclude.type.names";
private static final String INCLUDE_TEST_CODE_KEY = "include.test.code";
private final UniqueProperties properties = new UniqueProperties();
private String expectedLabelsFilename;
private String reportFilename;
private String projectName;
private String rootPackageName = "com.logica";
private final SortedSet includePackageNames = new TreeSet();
private final SortedSet excludePackageNames = new TreeSet();
private final SortedSet excludeTypeNames = new TreeSet();
private boolean includeTestCode = false;
private String buildNumber;
private ClassLoader classLoader;
private final SortedMap expectedLabels = new TreeMap();
void loadParams(final String propertiesFilename) {
LOGGER.info("*** Run parameters: ");
clear();
this.properties.load(new File(propertiesFilename));
String baseDir = FileUtils.getParent(FileUtils.getCanonicalPath(propertiesFilename)) + "/";
this.expectedLabelsFilename = this.properties.getProperty(EXPECTED_LABELS_FILENAME_KEY, DEFAULT_EXPECTED_LABELS_FILENAME);
if (!FileUtils.isAbsolute(this.expectedLabelsFilename)) {
this.expectedLabelsFilename = baseDir + this.expectedLabelsFilename;
}
validateIsTrue(FileUtils.exists(this.expectedLabelsFilename), "File not found: " + this.expectedLabelsFilename);
this.reportFilename = this.properties.getProperty(REPORT_FILENAME_KEY, DEFAULT_REPORT_FILENAME);
if (!FileUtils.isAbsolute(this.reportFilename)) {
this.reportFilename = baseDir + this.reportFilename;
}
validateIsTrue(FileUtils.exists(FileUtils.getParent(this.reportFilename)), "Directory not found: " + this.reportFilename);
this.projectName = this.properties.getProperty(PROJECT_NAME_KEY);
validateNotEmpty(this.projectName, "Invalid " + PROJECT_NAME_KEY);
this.rootPackageName = this.properties.getProperty(ROOT_PACKAGE_NAME_KEY, this.rootPackageName);
final String includeNames = this.properties.getProperty(INCLUDE_PACKAGE_NAMES_KEY);
validateNotEmpty(includeNames, "Invalid " + INCLUDE_PACKAGE_NAMES_KEY);
this.includePackageNames.addAll(Arrays.asList(includeNames.split(SEPARATOR)));
validateIsTrue(this.includePackageNames.size() > 0, "Invalid " + INCLUDE_PACKAGE_NAMES_KEY);
final String exPackageNames = this.properties.getProperty(EXCLUDE_PACKAGE_NAMES_KEY);
if (!isEmpty(exPackageNames)) {
this.excludePackageNames.addAll(Arrays.asList(exPackageNames.split(SEPARATOR)));
}
final String exTypeNames = this.properties.getProperty(EXCLUDE_TYPE_NAMES_KEY);
if (!isEmpty(exTypeNames)) {
this.excludeTypeNames.addAll(Arrays.asList(exTypeNames.split(SEPARATOR)));
}
this.includeTestCode = "true".equals(this.properties.getProperty(INCLUDE_TEST_CODE_KEY));
LOGGER.info("projectName = " + this.projectName);
LOGGER.info("rootPackageName = " + this.rootPackageName);
LOGGER.info("includePackageNames = " + this.includePackageNames);
LOGGER.info("excludePackageNames = " + this.excludePackageNames);
LOGGER.info("excludeTypeNames = " + this.excludeTypeNames);
LOGGER.info("includeTestCode = " + this.includeTestCode);
loadExpectedLabels();
}
private void loadExpectedLabels() {
LOGGER.info("*** Expected labels: ");
final UniqueProperties labels = new UniqueProperties();
labels.load(new File(this.expectedLabelsFilename));
this.expectedLabels.putAll(labels.getAsSortedMap());
}
private void clear() {
this.properties.clear();
this.expectedLabels.clear();
this.includePackageNames.clear();
this.excludePackageNames.clear();
this.excludeTypeNames.clear();
}
private boolean isEmpty(String value) {
return value == null || value.trim().length() == 0;
}
private void validateNotEmpty(String value, String msg) {
if (isEmpty(value)) {
throw new IllegalArgumentException(msg);
}
}
private void validateIsTrue(boolean mustBeTrue, String msg) {
if (!mustBeTrue) {
throw new IllegalArgumentException(msg);
}
}
String getProjectName() {
return this.projectName;
}
String getRootPackageName() {
return this.rootPackageName;
}
String getShortTypeName(String typeName) {
if (this.rootPackageName.length() > 0 && typeName.startsWith(this.rootPackageName)) {
int index = this.rootPackageName.length();
return ".." + typeName.substring(index);
}
return typeName;
}
SortedSet getIncludePackageNames() {
return this.includePackageNames;
}
SortedSet getExcludePackageNames() {
return this.excludePackageNames;
}
SortedSet getExcludeTypeNames() {
return excludeTypeNames;
}
boolean isIncludeTestCode() {
return this.includeTestCode;
}
SortedMap getExpectedLabels() {
return this.expectedLabels;
}
String getBuildNumber() {
return this.buildNumber;
}
void setBuildNumber(String buildNumber) {
this.buildNumber = buildNumber;
}
ClassLoader getClassLoader() {
return this.classLoader;
}
void setClassLoader(ClassLoader classLoader) {
this.classLoader = classLoader;
}
String getExpectedLabelsFilename() {
return this.expectedLabelsFilename;
}
String getReportFilename() {
return this.reportFilename;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy