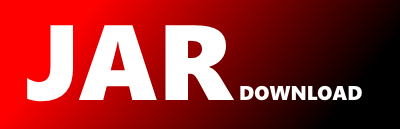
javarequirementstracer.UniqueProperties Maven / Gradle / Ivy
/*
* Copyright 2008-2009 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package javarequirementstracer;
import java.io.Closeable;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Map;
import java.util.Properties;
import java.util.SortedMap;
import java.util.TreeMap;
/**
* Like {@link Properties} but enforces the properties loaded from a file must be unique,
* though enforcing a property must not be set twice.
*
* @author Ronald Koster
*/
final class UniqueProperties extends Properties {
private static final long serialVersionUID = 1L;
private static final Logger LOGGER = Logger.getInstance(UniqueProperties.class);
/**
* @throws IllegalArgumentException in case a property is set twice.
*/
@Override
public Object put(Object key, Object value) {
if (containsKey(key)) {
throw new IllegalArgumentException("Unique violation: " + key);
}
return super.put(key, value);
}
void load(final File propertiesFile) {
LOGGER.info("$user.dir = " + System.getProperty("user.dir"));
LOGGER.info("propertiesFilename = " + propertiesFile.getAbsolutePath());
FileInputStream input = null;
try {
input = new FileInputStream(propertiesFile);
load(input);
} catch (FileNotFoundException fnfex) {
LOGGER.error(fnfex);
throw new UncheckedException(fnfex);
} catch (IOException ioex) {
LOGGER.error(ioex);
throw new UncheckedException(ioex);
} finally {
if (input != null) {
close(input);
}
}
}
private void close(Closeable stream) {
try {
stream.close();
} catch (IOException ioex) {
LOGGER.error(ioex);
throw new UncheckedException(ioex);
}
}
SortedMap getAsSortedMap() {
final SortedMap map = new TreeMap();
for (Map.Entry
© 2015 - 2024 Weber Informatics LLC | Privacy Policy