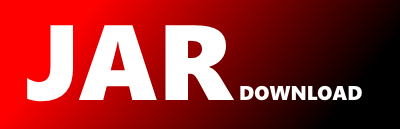
javarequirementstracer.XhtmlBuilder Maven / Gradle / Ivy
/*
* Copyright 2008-2009 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package javarequirementstracer;
import java.io.File;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* Helper class for creating XHTML.
*
* @author Ronald Koster
*/
final class XhtmlBuilder {
private static final String TABLE_START = "\n";
private final StringBuilder bldr = new StringBuilder();
private boolean parStarted = false;
@Override
public String toString() {
return this.bldr.toString();
}
XhtmlBuilder append(Object obj) {
this.bldr.append(obj);
return this;
}
XhtmlBuilder append(AttributeId spanId, Object obj) {
return spanStart(spanId).append(obj).spanEnd();
}
XhtmlBuilder start(String title) {
append("");
return append("\n\n\n").append(title).append(" ").append(STYLE).append("\n\n").heading(1, title);
}
XhtmlBuilder end() {
return append("\n\n\n");
}
XhtmlBuilder heading(int level, String title) {
return append("\n\n").append(title).append(" ");
}
XhtmlBuilder parStart() {
if (this.parStarted) {
throw new IllegalStateException("Paragraph not ended yet.");
}
this.parStarted = true;
return append(PAR_START);
}
XhtmlBuilder parEnd() {
if (!this.parStarted) {
throw new IllegalStateException("Paragraph not started yet.");
}
this.parStarted = false;
return append(PAR_END);
}
XhtmlBuilder spanStart(AttributeId id) {
this.bldr.append("");
return this;
}
XhtmlBuilder br() {
return append(BR);
}
XhtmlBuilder space() {
return append(SPACE);
}
XhtmlBuilder nbsp() {
return append(NBSP);
}
XhtmlBuilder tab() {
return append(TAB);
}
XhtmlBuilder table(final AttributeId id, final Map map, final String... headers) {
List> rows = new ArrayList>();
for (Map.Entry entry : map.entrySet()) {
List row = new ArrayList();
row.add(entry.getKey());
row.add(entry.getValue());
rows.add(row);
}
return table(id, rows, headers);
}
XhtmlBuilder table(final AttributeId id, final Collection> rows, final String... headers) {
validateTrue("At least rows or headers must not be empty.",
rows != null && rows.size() > 0 || headers != null && headers.length > 0);
this.bldr.append(TABLE_START);
attId(id);
if (headers != null && headers.length > 0) {
appendHeaders(headers);
}
if (rows != null) {
appendRows(rows);
}
return append(TABLE_END);
}
private void appendHeaders(final String... headers) {
append(ROW_START);
for (String header : headers) {
append(HEADER_START).append(header).append(HEADER_END);
}
append(ROW_END);
}
private void appendRows(final Collection> rows) {
for (Collection row : rows) {
append(ROW_START);
for (String column : row) {
append(COLUMN_START);
append(column == null || column.length() == 0 ? NBSP : column);
append(COLUMN_END);
}
append(ROW_END);
}
}
void write(final File reportFile) {
FileUtils.writeFile(reportFile, toString());
}
private void validateTrue(String msg, boolean condition) {
if (!condition) {
throw new IllegalArgumentException(msg);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy