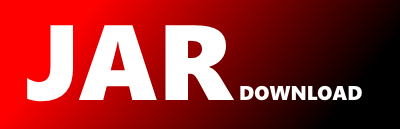
net.sourceforge.schemaspy.view.HtmlRelationshipsPage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of schemaspy Show documentation
Show all versions of schemaspy Show documentation
SchemaSpy generates HTML and PNG-based entity relationship diagrams from JDBC-enabled databases.
The newest version!
/*
* This file is a part of the SchemaSpy project (http://schemaspy.sourceforge.net).
* Copyright (C) 2004, 2005, 2006, 2007, 2008, 2009, 2010 John Currier
*
* SchemaSpy is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* SchemaSpy is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*/
package net.sourceforge.schemaspy.view;
import java.io.File;
import java.io.IOException;
import java.util.Set;
import java.util.logging.Level;
import java.util.logging.Logger;
import net.sourceforge.schemaspy.model.Database;
import net.sourceforge.schemaspy.model.TableColumn;
import net.sourceforge.schemaspy.util.Dot;
import net.sourceforge.schemaspy.util.LineWriter;
/**
* The page that contains the overview entity relationship diagrams.
*
* @author John Currier
*/
public class HtmlRelationshipsPage extends HtmlDiagramFormatter {
private static final HtmlRelationshipsPage instance = new HtmlRelationshipsPage();
private static final boolean fineEnabled = Logger.getLogger(HtmlRelationshipsPage.class.getName()).isLoggable(Level.FINE);
/**
* Singleton: Don't allow instantiation
*/
private HtmlRelationshipsPage() {
}
/**
* Singleton accessor
*
* @return the singleton instance
*/
public static HtmlRelationshipsPage getInstance() {
return instance;
}
public boolean write(Database db, File diagramDir, String dotBaseFilespec, boolean hasOrphans, boolean hasRealRelationships, boolean hasImpliedRelationships, Set excludedColumns, LineWriter html) {
File compactRelationshipsDotFile = new File(diagramDir, dotBaseFilespec + ".real.compact.dot");
File compactRelationshipsDiagramFile = new File(diagramDir, dotBaseFilespec + ".real.compact.png");
File largeRelationshipsDotFile = new File(diagramDir, dotBaseFilespec + ".real.large.dot");
File largeRelationshipsDiagramFile = new File(diagramDir, dotBaseFilespec + ".real.large.png");
File compactImpliedDotFile = new File(diagramDir, dotBaseFilespec + ".implied.compact.dot");
File compactImpliedDiagramFile = new File(diagramDir, dotBaseFilespec + ".implied.compact.png");
File largeImpliedDotFile = new File(diagramDir, dotBaseFilespec + ".implied.large.dot");
File largeImpliedDiagramFile = new File(diagramDir, dotBaseFilespec + ".implied.large.png");
try {
Dot dot = getDot();
if (dot == null) {
writeHeader(db, null, "All Relationships", hasOrphans, html);
html.writeln("");
writeInvalidGraphvizInstallation(html);
html.writeln("");
writeFooter(html);
return false;
}
writeHeader(db, "All Relationships", hasOrphans, hasRealRelationships, hasImpliedRelationships, html);
html.writeln("");
html.writeln("");
writeGeneratedBy(db.getConnectTime(), html);
html.writeln(" ");
html.writeln("");
writeLegend(false, html);
html.writeln(" ");
if (!hasRealRelationships) {
html.writeln("");
if (hasImpliedRelationships) {
html.writeln("No 'real' Foreign Key relationships were detected in the schema.
");
html.writeln("Displayed relationships are implied by a column's name/type/size matching another table's primary key.");
}
else
html.writeln("No relationships were detected in the schema.");
html.writeln("
");
}
html.writeln("");
html.writeln("");
html.writeln("
");
}
@Override
protected boolean isRelationshipsPage() {
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy