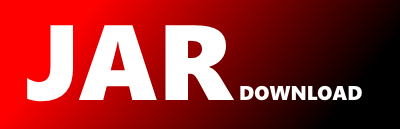
net.sourceforge.tink.model.helpers.ConsoleOutput Maven / Gradle / Ivy
/**
* Copyright 2008,2009 Ivan SZKIBA
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* under the License.
*/
package net.sourceforge.tink.model.helpers;
import net.sourceforge.tink.model.Output;
import java.io.PrintStream;
public class ConsoleOutput implements Output
{
public static enum Verbosity
{
DEBUG,
VERBOSE,
INFO,
WARNING,
ERROR;
public static Verbosity fromString(String str)
{
return valueOf(str.toUpperCase());
}
}
private PrintStream stream;
private Verbosity verbosity;
public ConsoleOutput()
{
this(Verbosity.VERBOSE, System.err);
}
public ConsoleOutput(Verbosity verbosity)
{
this(verbosity, System.err);
}
public ConsoleOutput(Verbosity verbosity, PrintStream stream)
{
this.verbosity = verbosity;
this.stream = stream;
}
@Override public boolean isDebugEnabled()
{
return verbosity.compareTo(Verbosity.DEBUG) <= 0;
}
@Override public boolean isErrorEnabled()
{
return verbosity.compareTo(Verbosity.ERROR) <= 0;
}
@Override public boolean isInfoEnabled()
{
return verbosity.compareTo(Verbosity.INFO) <= 0;
}
@Override public boolean isVerboseEnabled()
{
return verbosity.compareTo(Verbosity.VERBOSE) <= 0;
}
@Override public boolean isWarningEnabled()
{
return verbosity.compareTo(Verbosity.WARNING) <= 0;
}
@Override public void debug(String message, Object... params)
{
if (isDebugEnabled())
{
stream.format(message, params);
stream.println();
}
}
@Override public void debug(Throwable cause, String message, Object... params)
{
if (isDebugEnabled())
{
cause.printStackTrace(stream);
stream.format(message, params);
stream.println();
}
}
@Override public void error(String message, Object... params)
{
if (isErrorEnabled())
{
stream.format(message, params);
stream.println();
}
}
@Override public void error(Throwable cause, String message, Object... params)
{
if (isErrorEnabled())
{
stream.println(cause.toString());
stream.format(message, params);
stream.println();
}
}
@Override public void info(String message, Object... params)
{
if (isInfoEnabled())
{
stream.format(message, params);
stream.println();
}
}
@Override public void info(Throwable cause, String message, Object... params)
{
if (isInfoEnabled())
{
stream.println(cause.toString());
stream.format(message, params);
stream.println();
}
}
@Override public void verbose(String message, Object... params)
{
if (isVerboseEnabled())
{
stream.format(message, params);
stream.println();
}
}
@Override public void verbose(Throwable cause, String message, Object... params)
{
if (isVerboseEnabled())
{
cause.printStackTrace(stream);
stream.format(message, params);
stream.println();
}
}
@Override public void warning(String message, Object... params)
{
if (isWarningEnabled())
{
stream.format(message, params);
stream.println();
}
}
@Override public void warning(Throwable cause, String message, Object... params)
{
if (isWarningEnabled())
{
stream.println(cause.toString());
stream.format(message, params);
stream.println();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy