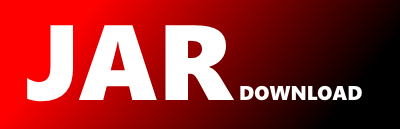
net.sourceforge.tink.model.helpers.ProcessorBuilder Maven / Gradle / Ivy
/**
* Copyright 2008,2009 Ivan SZKIBA
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* under the License.
*/
package net.sourceforge.tink.model.helpers;
import net.sourceforge.tink.model.FileObject;
import net.sourceforge.tink.model.Output;
import net.sourceforge.tink.model.TinkException;
import net.sourceforge.tink.model.TinkProcessor;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.types.FileSet;
import java.io.File;
import java.util.Arrays;
public class ProcessorBuilder extends AbstractBuilder
{
private final Config config;
private final Project project;
public ProcessorBuilder(Config config)
{
this.config = config;
this.project = new Project();
this.project.init();
}
public ProcessorBuilder(Config config, Project project)
{
this.config = config;
this.project = project;
}
public TinkProcessor buildProcessor() throws TinkException
{
if ((config.getDestdir() != null) && !config.getDestdir().exists())
{
config.getDestdir().mkdir();
}
requireDirectory(config.getDestdir(), "destdir", "Destination");
requireFile(config.getTemplate(), "template", "Template");
requireFile(config.getData(), "data", "Data");
if (config.getSrc() == null)
{
requireDirectory(config.getSrcdir(), "srcdir", "Source");
}
FileSet set = getSourceSet();
TinkProcessor processor = TinkProcessor.newInstance();
TinkProcessor.SimpleConfig simpleConfig = new TinkProcessor.SimpleConfig();
simpleConfig.setOutputDirectory(config.getDestdir());
simpleConfig.setTemplate(new FileObject(config.getTemplate().getAbsolutePath()));
simpleConfig.setData(new FileObject(config.getData().getAbsolutePath()));
simpleConfig.setInputDirectory(set.getDir(project));
simpleConfig.getInputFiles().addAll(Arrays.asList(set.getDirectoryScanner().getIncludedFiles()));
processor.setConfig(simpleConfig);
processor.setOutput(config.getOutput());
return processor;
}
protected FileSet getSourceSet()
{
FileSet ret = config.getSrc();
if (ret == null)
{
ret = new FileSet();
ret.setProject(project);
ret.setDir(config.getSrcdir());
if (config.getIncludes() != null)
{
ret.setIncludes(config.getIncludes());
}
if (config.getExcludes() != null)
{
ret.setExcludes(config.getExcludes());
}
if (config.getIncludesfile() != null)
{
ret.setIncludesfile(config.getIncludesfile());
}
if (config.getExcludesfile() != null)
{
ret.setExcludesfile(config.getExcludesfile());
}
}
return ret;
}
public static interface Config
{
File getData();
File getDestdir();
String getExcludes();
File getExcludesfile();
String getIncludes();
File getIncludesfile();
Output getOutput();
FileSet getSrc();
File getSrcdir();
File getTemplate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy