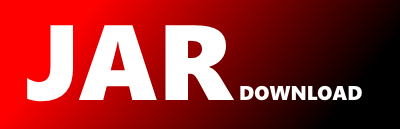
net.sourceforge.tink.model.impl.TinkProcessorImpl Maven / Gradle / Ivy
/**
* Copyright 2008,2009 Ivan SZKIBA
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* under the License.
*/
package net.sourceforge.tink.model.impl;
import net.sourceforge.tink.model.FileObject;
import net.sourceforge.tink.model.Output;
import net.sourceforge.tink.model.TemplateData;
import net.sourceforge.tink.model.TinkContext;
import net.sourceforge.tink.model.TinkException;
import net.sourceforge.tink.model.TinkProcessor;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.URI;
public class TinkProcessorImpl extends TinkProcessor
{
private static final String MSG_TRANSFORM = "Transforming file %s";
private static final String MSG_COPY = "Copying file %s";
private static final int BUFFER_SIZE = 1024;
private TinkComponent[] components;
private TinkParser parser;
private TinkResource resource;
private TinkTransformer transformer;
private TinkUtilImpl util;
public TinkProcessorImpl()
{
parser = new TinkParser();
resource = new TinkResource();
transformer = new TinkTransformer();
util = new TinkUtilImpl();
components = new TinkComponent[] { util, parser, resource, transformer };
}
@Override public void execute() throws TinkException
{
TinkContext context = new TinkContextImpl(getOutput());
Config fileManager = getConfig();
Output out = getOutput();
transformer.setTemplateFile(fileManager.getTemplate());
init(context);
TemplateData data = new TemplateData(context, util, parser.parseData(fileManager.getData()));
File outputTop = fileManager.getOutputDirectory();
File templateTop = fileManager.getTemplate().getTopFile();
out.info("Transforming file to %s", outputTop);
for (FileObject inputFile : fileManager.listInput())
{
data.setPage(parser.parsePage(inputFile));
FileObject outputFile = new FileObject(outputTop, inputFile.getRelativePath());
outputFile.getParentFile().mkdirs();
// apply page template
out.verbose(MSG_TRANSFORM, inputFile.getRelativePath());
transformer.transform(data, outputFile);
// copy resources
for (URI uri : resource.findResources(parser.parsePage(outputFile)))
{
if (!uri.isAbsolute())
{
FileObject resOut = new FileObject(outputFile.getTopFile(), outputFile, uri);
FileObject resIn = new FileObject(inputFile.getTopFile(), inputFile, uri);
// template resource should expand input resource should copy
if (resIn.exists())
{
out.verbose(MSG_COPY, resOut.getRelativePath());
copy(resIn, resOut);
}
else
{
resIn = new FileObject(templateTop, stripCommon(resIn.getRelativePath(), inputFile.getRelativePath()));
out.verbose(MSG_TRANSFORM, resOut.getRelativePath());
transformer.transform(resIn, data, resOut);
}
}
}
}
destroy();
}
protected void copy(FileObject inputFile, FileObject outputFile) throws TinkException
{
try
{
FileInputStream is = new FileInputStream(inputFile);
FileOutputStream os = new FileOutputStream(outputFile);
byte[] buff = new byte[BUFFER_SIZE];
int n;
while ((n = is.read(buff)) > 0)
{
os.write(buff, 0, n);
}
is.close();
os.close();
}
catch (IOException x)
{
throw new TinkException("Error copying file: " + inputFile.getAbsolutePath() + " -> " + outputFile.getAbsolutePath(), x);
}
}
protected void destroy() throws TinkException
{
for (int i = components.length - 1; i > 0; i--)
{
components[i].destroy();
}
}
protected void init(TinkContext context) throws TinkException
{
for (int i = 0; i < components.length; i++)
{
components[i].init(context);
}
}
private String stripCommon(String path, String base)
{
int i = 0;
int n = Math.min(path.length(), base.length());
while ((i < n) && (path.charAt(i) == base.charAt(i)))
{
i++;
}
return path.substring(Math.max(0, i - 1));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy