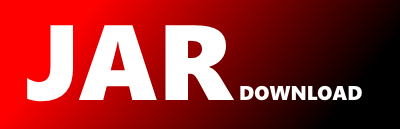
net.spals.appbuilder.app.examples.grpc.rest.UserServiceV2GrpcJerseyResource Maven / Gradle / Ivy
package net.spals.appbuilder.app.examples.grpc.rest;
import com.fullcontact.rpc.jersey.HttpHeaderInterceptors;
import com.fullcontact.rpc.jersey.JerseyUnaryObserver;
import com.fullcontact.rpc.jersey.JerseyStreamingObserver;
import com.fullcontact.rpc.jersey.RequestParser;
import com.google.protobuf.Descriptors;
import com.google.protobuf.Descriptors;
import com.google.protobuf.Message;
import java.io.OutputStream;
import java.io.IOException;
import javax.servlet.AsyncContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.ws.rs.*;
import javax.ws.rs.core.*;
import javax.ws.rs.container.AsyncResponse;
import javax.ws.rs.container.Suspended;
import static com.fullcontact.rpc.jersey.JerseyStreamingObserver.VARIANT_LIST;
@javax.annotation.Generated(
value = "by grpc-jersey compiler (version 0.3.2)",
comments = "Source: rest_routes_v2.proto")
@Produces({"application/json; charset=UTF-8"})
@Consumes({"application/json; charset=UTF-8"})
@Path("/")
public class UserServiceV2GrpcJerseyResource {
private net.spals.appbuilder.app.examples.grpc.rest.UserServiceV2Grpc.UserServiceV2Stub stub;
public UserServiceV2GrpcJerseyResource(net.spals.appbuilder.app.examples.grpc.rest.UserServiceV2Grpc.UserServiceV2Stub stub) {
this.stub = stub;
}
@DELETE
@Path("/v2/users/{id}")
public void DeleteUserV2_DELETE_0(
@PathParam("id") String id,
@Context UriInfo uriInfo,
@Context HttpHeaders headers
,@Suspended final AsyncResponse asyncResponse) throws IOException {
HttpHeaderInterceptors.HttpHeaderClientInterceptor interceptor =
HttpHeaderInterceptors.clientInterceptor(headers);
JerseyUnaryObserver observer = new JerseyUnaryObserver<>(asyncResponse, interceptor);
net.spals.appbuilder.app.examples.grpc.rest.DeleteUserRequestV2.Builder r = net.spals.appbuilder.app.examples.grpc.rest.DeleteUserRequestV2.newBuilder();
net.spals.appbuilder.app.examples.grpc.rest.UserServiceV2Grpc.UserServiceV2Stub stub = this.stub;
try {
stub = RequestParser.parseHeaders(headers, stub);
stub = stub.withInterceptors(interceptor);
RequestParser.parseQueryParams(uriInfo, r);
RequestParser.setFieldSafely(r, "id", id);
} catch(Exception e) {
observer.onError(e);
return;
}
stub.deleteUserV2(r.build(), observer);
}
@GET
@Path("/v2/users/{id}")
public void GetUserV2_GET_0(
@PathParam("id") String id,
@Context UriInfo uriInfo,
@Context HttpHeaders headers
,@Suspended final AsyncResponse asyncResponse) throws IOException {
HttpHeaderInterceptors.HttpHeaderClientInterceptor interceptor =
HttpHeaderInterceptors.clientInterceptor(headers);
JerseyUnaryObserver observer = new JerseyUnaryObserver<>(asyncResponse, interceptor);
net.spals.appbuilder.app.examples.grpc.rest.GetUserRequestV2.Builder r = net.spals.appbuilder.app.examples.grpc.rest.GetUserRequestV2.newBuilder();
net.spals.appbuilder.app.examples.grpc.rest.UserServiceV2Grpc.UserServiceV2Stub stub = this.stub;
try {
stub = RequestParser.parseHeaders(headers, stub);
stub = stub.withInterceptors(interceptor);
RequestParser.parseQueryParams(uriInfo, r);
RequestParser.setFieldSafely(r, "id", id);
} catch(Exception e) {
observer.onError(e);
return;
}
stub.getUserV2(r.build(), observer);
}
@POST
@Path("/v2/users")
public void PostUserV2_POST_0(
@Context UriInfo uriInfo,
@Context HttpHeaders headers
,String body
,@Suspended final AsyncResponse asyncResponse) throws IOException {
HttpHeaderInterceptors.HttpHeaderClientInterceptor interceptor =
HttpHeaderInterceptors.clientInterceptor(headers);
JerseyUnaryObserver observer = new JerseyUnaryObserver<>(asyncResponse, interceptor);
net.spals.appbuilder.app.examples.grpc.rest.PostUserRequestV2.Builder r = net.spals.appbuilder.app.examples.grpc.rest.PostUserRequestV2.newBuilder();
net.spals.appbuilder.app.examples.grpc.rest.UserServiceV2Grpc.UserServiceV2Stub stub = this.stub;
try {
stub = RequestParser.parseHeaders(headers, stub);
stub = stub.withInterceptors(interceptor);
RequestParser.handleBody("*", r, body);
} catch(Exception e) {
observer.onError(e);
return;
}
stub.postUserV2(r.build(), observer);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy