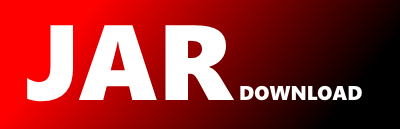
net.spals.appbuilder.graph.model.ServiceTree Maven / Gradle / Ivy
package net.spals.appbuilder.graph.model;
import com.google.common.base.Preconditions;
import org.jgrapht.DirectedGraph;
import org.jgrapht.experimental.dag.DirectedAcyclicGraph;
import org.jgrapht.graph.DefaultEdge;
import org.jgrapht.graph.GraphDelegator;
import java.util.Collections;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import static net.spals.appbuilder.graph.model.ServiceTreeVertex.createTreeRoot;
/**
* An implementation of a {@link org.jgrapht.DirectedGraph}
* which stores relationships between micro-services.
*
* @author tkral
*/
public class ServiceTree
extends GraphDelegator, DefaultEdge>
implements DirectedGraph, DefaultEdge> {
private final IServiceTreeVertex> root;
ServiceTree(final IServiceDAGVertex> root) {
this(createTreeRoot(root));
}
ServiceTree(final IServiceTreeVertex> root) {
super(new DirectedAcyclicGraph<>(DefaultEdge.class));
Preconditions.checkArgument(root.isRoot());
this.root = root;
addVertex(root);
}
public IServiceTreeVertex> getRoot() {
return root;
}
public Set> getSiblings(final IServiceTreeVertex> vertex) {
if (vertex.isRoot()) {
return Collections.emptySet();
}
final IServiceTreeVertex> parent = vertex.getParent().get();
final Set parentEdges = outgoingEdgesOf(parent);
return parentEdges.stream()
.map(parentEdge -> getEdgeTarget(parentEdge))
.filter(sibling -> !sibling.equals(vertex))
.collect(Collectors.toSet());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy