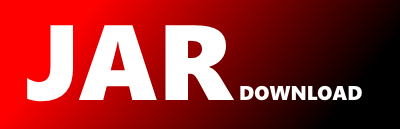
net.tascalate.concurrent.PromiseOperations Maven / Gradle / Ivy
/**
* Copyright 2015-2020 Valery Silaev (http://vsilaev.com)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.tascalate.concurrent;
import java.util.Optional;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Executor;
import java.util.function.Function;
import java.util.stream.Collector;
import java.util.stream.Stream;
public class PromiseOperations {
private PromiseOperations() {}
// Lifted is somewhat questionable, but here it exists for symmetry with dropped()
public static Promise> lift(CompletionStage extends T> promise) {
return lift(Promises.from(promise));
}
public static Promise> lift(Promise extends T> promise) {
return promise.dependent()
.thenApply(Promises::success, true)
.unwrap();
}
public static Promise drop(CompletionStage extends CompletionStage> promise) {
return drop(Promises.from(promise));
}
public static Promise drop(Promise extends CompletionStage> promise) {
return promise.dependent()
.thenCompose(Promises::from, true)
.unwrap();
}
public static Promise> streamResult(CompletionStage extends T> promise) {
return streamResult(Promises.from(promise));
}
public static Promise> streamResult(Promise extends T> promise) {
return promise.dependent()
.handle((r, e) -> null == e ? Stream.of(r) : Stream.empty(), true)
.unwrap();
}
public static Promise> optionalResult(CompletionStage extends T> promise) {
return optionalResult(Promises.from(promise));
}
public static Promise> optionalResult(Promise extends T> promise) {
return promise.dependent()
.handle((r, e) -> Optional.ofNullable(null == e ? r : null), true)
.unwrap();
}
public static Function, Promise>
tryApply(Function super R, ? extends T> fn) {
return resourcePromise -> Promises.tryApply(resourcePromise, fn);
}
public static Function, Promise>
tryApplyEx(Function super R, ? extends T> fn) {
return resourcePromise -> Promises.tryApplyEx(resourcePromise, fn);
}
public static Function, Promise>
tryCompose(Function super R, ? extends CompletionStage> fn) {
return resourcePromise -> Promises.tryCompose(resourcePromise, fn);
}
public static Function, Promise>
tryComposeEx(Function super R, ? extends CompletionStage> fn) {
return resourcePromise -> Promises.tryComposeEx(resourcePromise, fn);
}
public static Function>, Promise> partitionedItems(
int batchSize,
Function super T, CompletionStage extends T>> spawner,
Collector downstream) {
return p -> p.dependent()
.thenCompose(values ->
Promises.partitioned(values, batchSize, spawner, downstream), true)
.unwrap();
}
public static Function>, Promise> partitionedItems(
int batchSize,
Function super T, CompletionStage extends T>> spawner,
Collector downstream,
Executor downstreamExecutor) {
return p -> p.dependent()
.thenCompose(values ->
Promises.partitioned(values, batchSize, spawner, downstream, downstreamExecutor), true)
.unwrap();
}
public static Function>, Promise> partitionedStream(
int batchSize,
Function super T, CompletionStage extends T>> spawner,
Collector downstream) {
return p -> p.dependent()
.thenCompose(values ->
Promises.partitioned(values, batchSize, spawner, downstream), true)
.unwrap();
}
public static Function>, Promise> partitionedStream(
int batchSize,
Function super T, CompletionStage extends T>> spawner,
Collector downstream,
Executor downstreamExecutor) {
return p -> p.dependent()
.thenCompose(values ->
Promises.partitioned(values, batchSize, spawner, downstream, downstreamExecutor), true)
.unwrap();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy