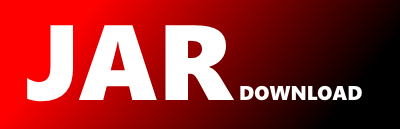
net.tascalate.concurrent.CompletableFutureWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of net.tascalate.concurrent Show documentation
Show all versions of net.tascalate.concurrent Show documentation
Implementation of blocking (IO-Bound) cancellable java.util.concurrent.CompletionStage
and related extensions to java.util.concurrent.ExecutorService-s
/**
* Copyright 2015-2020 Valery Silaev (http://vsilaev.com)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.tascalate.concurrent;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Executor;
import java.util.function.Function;
import net.tascalate.concurrent.decorators.CompletableFutureDecorator;
public class CompletableFutureWrapper extends CompletableFutureDecorator {
protected CompletableFutureWrapper() {
super();
}
protected CompletableFutureWrapper(CompletableFuture delegate) {
super(delegate);
}
protected boolean success(T value) {
return onSuccess(value);
}
@Deprecated
protected boolean onSuccess(T value) {
return delegate.complete(value);
}
protected boolean failure(Throwable ex) {
return onFailure(ex);
}
@Deprecated
protected boolean onFailure(Throwable ex) {
return delegate.completeExceptionally(ex);
}
boolean complete(T value, Throwable ex) {
return null == ex ? success(value) : failure(ex);
}
@Override
public CompletableFuture toCompletableFuture() {
// Return concrete subclass that neither completes nor cancels this wrapper
return (CompletableFuture)delegate.thenApply(Function.identity());
}
// Report self-origin
@Override
public CompletionStage α() {
return this;
}
@Override
protected Promise wrap(CompletionStage original) {
return new CompletableFutureWrapper<>((CompletableFuture)original);
}
// By default CompletableFuture doesn't interrupt a promise
// from thenCompose(fn) and exceptionallyCompose!
@Override
public Promise thenCompose(Function super T, ? extends CompletionStage> fn) {
CompletionStageRef ref = new CompletionStageRef<>();
return super.thenCompose(ref.captureResult(fn)).onCancel(ref.cancel);
}
@Override
public Promise thenComposeAsync(Function super T, ? extends CompletionStage> fn) {
CompletionStageRef ref = new CompletionStageRef<>();
return super.thenComposeAsync(ref.captureResult(fn)).onCancel(ref.cancel);
}
@Override
public Promise thenComposeAsync(Function super T, ? extends CompletionStage> fn,
Executor executor) {
CompletionStageRef ref = new CompletionStageRef<>();
return super.thenComposeAsync(ref.captureResult(fn), executor).onCancel(ref.cancel);
}
// Default CompletionStage API implementation for exceptionallyAsync / exceptionallyCompose[Async]
// doesn't handle cancellation well due to numerous orchestrated calls (handle->handleAsync->thenCompose
// Use own implementation here for safety
@Override
public Promise exceptionallyAsync(Function fn) {
return PromiseHelper.exceptionallyAsync(this, fn);
}
@Override
public Promise exceptionallyAsync(Function fn, Executor executor) {
return PromiseHelper.exceptionallyAsync(this, fn, executor);
}
@Override
public Promise exceptionallyCompose(Function> fn) {
return PromiseHelper.exceptionallyCompose(this, fn);
}
@Override
public Promise exceptionallyComposeAsync(Function> fn) {
return PromiseHelper.exceptionallyComposeAsync(this, fn);
}
@Override
public Promise exceptionallyComposeAsync(Function> fn,
Executor executor) {
return PromiseHelper.exceptionallyComposeAsync(this, fn, executor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy