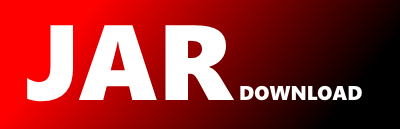
net.thucydides.plugins.jira.requirements.StructureRequirementsTree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thucydides-structure-plugin-requirements-provider Show documentation
Show all versions of thucydides-structure-plugin-requirements-provider Show documentation
Read Thucydides requirements from JIRA using the JIRA Structure plugin
package net.thucydides.plugins.jira.requirements;
import com.google.common.base.Splitter;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import java.util.Arrays;
import java.util.List;
import java.util.Stack;
public class StructureRequirementsTree {
public static class RequirementTreeNode {
final Integer id;
List children;
public RequirementTreeNode(Integer id) {
this.id = id;
this.children = Lists.newArrayList();
}
public void addChild(RequirementTreeNode child) {
children.add(child);
}
public Integer getId() {
return id;
}
public List getChildren() {
return ImmutableList.copyOf(children);
}
}
public static StructureRequirementsTree forFormula(String formula) {
List leaves = Lists.newArrayList(Splitter.on(",").omitEmptyStrings().split(formula));
List nodes = Lists.newArrayList();
Stack nodeStack = new Stack();
RequirementTreeNode currentNode = null;
int currentLevel = 0;
for(String leaf : leaves) {
int id = getIdOf(leaf);
int level = getLevelOf(leaf);
RequirementTreeNode node = new RequirementTreeNode(id);
if (top(level)) {
nodes.add(node);
currentNode = node;
currentLevel = level;
} else if (level > currentLevel) {
nodeStack.push(currentNode);
currentNode.addChild(node);
currentNode = node;
currentLevel = level;
} else if (level == currentLevel) {
nodeStack.peek().addChild(node);
} else if (level < currentLevel) {
nodeStack.pop();
nodeStack.peek().addChild(node);
currentNode = node;
currentLevel = level;
}
}
return new StructureRequirementsTree(nodes);
}
private static boolean top(int level) {
return level == 0;
}
private List nodes;
public StructureRequirementsTree(List nodes) {
this.nodes = nodes;
}
public List getNodes() {
return ImmutableList.copyOf(nodes);
}
private static Integer getIdOf(String leaf) {
int separator = leaf.indexOf(":");
return Integer.valueOf(leaf.substring(0, separator));
}
private static Integer getLevelOf(String leaf) {
int separator = leaf.indexOf(":");
return Integer.valueOf(leaf.substring(separator + 1));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy