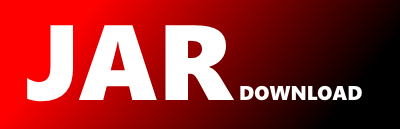
net.thucydides.core.steps.StepIndex Maven / Gradle / Ivy
package net.thucydides.core.steps;
import net.thucydides.core.annotations.AnnotatedFields;
import net.thucydides.core.annotations.Step;
import net.thucydides.core.annotations.StepProvider;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
/**
* The Step Index class is a way to allow the dynamic discovery of what test steps are available.
* This assumes you are writing your Page Objects and ScenarioStep classes in a separate artifact,
* which unit tests can then invoke. This is the most flexible way of implementing the page objects
* and steps.
*
* To use this class, override it and add a public array of classes containing the list of scenario step
* classes your test API provides. Then annotate this field using the '@StepProvider' annotation,
* as shown here:
*
public class MyWebSiteStepIndex extends StepIndex {
@StepProvider
public Class>[] stepClasses = {AddWidgetScenarioSteps.class, SearchWidgetsScenarioSteps.class};
}
*
*
* Users can then discover the scenario classes as shown here:
*
* List stepMethods = index.getStepsFor(scenarioClass);
*
*
* They can also discover the scenario step methods as shown here:
*
* StepIndex index = new MyWebSiteStepIndex();
List stepClasses = index.getStepClasses();
*
*
* @author johnsmart
*
*/
public abstract class StepIndex {
public List> getStepClasses() {
Field stepProviderField = getStepProviderField();
List> stepProviders;
try {
Class>[] providerFieldValue = (Class>[]) stepProviderField.get(this);
stepProviders = getStepProvidersFrom(providerFieldValue);
} catch (IllegalArgumentException e) {
throw new IllegalArgumentException("No step provider field found.", e);
} catch (IllegalAccessException e) {
throw new IllegalArgumentException("No step provider field found.", e);
}
return stepProviders;
}
public List getStepsFor(final Class extends ScenarioSteps> scenarioClass) {
List steps = new ArrayList();
Method[] methods = scenarioClass.getMethods();
for(Method method : methods) {
if (method.isAnnotationPresent(Step.class)) {
steps.add(method);
}
}
return steps;
}
@SuppressWarnings("unchecked")
private List> getStepProvidersFrom(final Class>[] providerFieldValue) {
List> stepProviders = new ArrayList>();
for(Class> providerClass : providerFieldValue) {
if (ScenarioSteps.class.isAssignableFrom(providerClass)) {
stepProviders.add((Class extends ScenarioSteps>) providerClass);
} else {
throw new IllegalArgumentException(providerClass + " needs to extend ScenarioSteps");
}
}
return stepProviders;
}
private Field getStepProviderField() {
for (Field field : AnnotatedFields.of(this.getClass()).allFields()) {
if (field.isAnnotationPresent(StepProvider.class)) {
return field;
}
}
throw new IllegalArgumentException("No step provider field found.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy