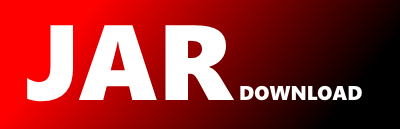
net.thucydides.junit.pipeline.CSVData Maven / Gradle / Ivy
package net.thucydides.junit.pipeline;
import au.com.bytecode.opencsv.CSVReader;
import net.thucydides.core.csv.FieldName;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.Reader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class CSVData {
private final String path;
public static CSVData fromFile(String path) throws FileNotFoundException {
return new CSVData(path);
}
public CSVData(String path) {
this.path = path;
}
private Reader readerFor(String path) throws FileNotFoundException {
if (isAClasspathResource(path)) {
try {
return new InputStreamReader(Thread.currentThread().getContextClassLoader().getResourceAsStream(path));
} catch(Throwable e) {
throw new FileNotFoundException("Could not load test data from " + path);
}
}
return new FileReader(new File(path));
}
private boolean isAClasspathResource(final String path) {
return (!validFileSystemPath(path));
}
private boolean validFileSystemPath(final String path) {
File file = new File(path);
return file.exists();
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy