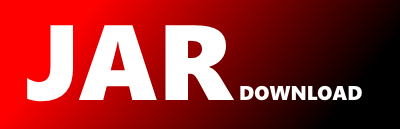
net.time4j.EnumElement Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (EnumElement.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j;
import net.time4j.engine.AttributeQuery;
import net.time4j.engine.ChronoDisplay;
import net.time4j.engine.ElementRule;
import net.time4j.format.Attributes;
import net.time4j.format.CalendarText;
import net.time4j.format.NumericalElement;
import net.time4j.format.OutputContext;
import net.time4j.format.TextAccessor;
import net.time4j.format.TextElement;
import net.time4j.format.TextWidth;
import java.io.IOException;
import java.io.InvalidObjectException;
import java.io.ObjectStreamException;
import java.text.ParsePosition;
import java.util.Locale;
import static net.time4j.format.CalendarText.ISO_CALENDAR_TYPE;
/**
* Allgemeines verstellbares chronologisches Element auf enum-Basis.
*
* @param generic enum type of element values
* @author Meno Hochschild
* @doctags.concurrency
*/
final class EnumElement>
extends AbstractDateElement
implements NavigableElement, NumericalElement, TextElement {
//~ Statische Felder/Initialisierungen --------------------------------
/** Element-Index. */
static final int MONTH = 101;
/** Element-Index. */
static final int DAY_OF_WEEK = 102;
/** Element-Index. */
static final int QUARTER_OF_YEAR = 103;
private static final long serialVersionUID = 2055272540517425102L;
//~ Instanzvariablen --------------------------------------------------
private transient final Class type;
private transient final V dmin;
private transient final V dmax;
private transient final int index;
private transient final char symbol;
private transient final ElementRule, V> rule;
//~ Konstruktoren -----------------------------------------------------
/**
* Konstruiert ein neues Element mit den angegebenen Details.
*
* @param name name of element
* @param type reified type of element values
* @param defaultMin default minimum
* @param defaultMax default maximum
* @param index element index
* @param symbol CLDR-symbol used in format patterns
*/
EnumElement(
String name,
Class type,
V defaultMin,
V defaultMax,
int index,
char symbol
) {
this(name, type, defaultMin, defaultMax, index, symbol, null);
}
/**
* Konstruiert ein neues Element mit den angegebenen Details.
*
* @param name name of element
* @param type reified type of element values
* @param defaultMin default minimum
* @param defaultMax default maximum
* @param index element index
* @param symbol CLDR-symbol used in format patterns
*/
EnumElement(
String name,
Class type,
V defaultMin,
V defaultMax,
int index,
char symbol,
ElementRule, V> rule
) {
super(name);
this.type = type;
this.dmin = defaultMin;
this.dmax = defaultMax;
this.index = index;
this.symbol = symbol;
this.rule = rule;
}
//~ Methoden ----------------------------------------------------------
@Override
public Class getType() {
return this.type;
}
@Override
public char getSymbol() {
return this.symbol;
}
@Override
public V getDefaultMinimum() {
return this.dmin;
}
@Override
public V getDefaultMaximum() {
return this.dmax;
}
@Override
public boolean isDateElement() {
return true;
}
@Override
public boolean isTimeElement() {
return false;
}
@Override
public ElementOperator setToNext(V value) {
return new NavigationOperator(
this, ElementOperator.OP_NAV_NEXT, value);
}
@Override
public ElementOperator setToPrevious(V value) {
return new NavigationOperator(
this, ElementOperator.OP_NAV_PREVIOUS, value);
}
@Override
public ElementOperator setToNextOrSame(V value) {
return new NavigationOperator(
this, ElementOperator.OP_NAV_NEXT_OR_SAME, value);
}
@Override
public ElementOperator setToPreviousOrSame(V value) {
return new NavigationOperator(
this, ElementOperator.OP_NAV_PREVIOUS_OR_SAME, value);
}
@Override
public int numerical(V value) {
return value.ordinal() + 1;
}
@Override
public void print(
ChronoDisplay context,
Appendable buffer,
AttributeQuery attributes
) throws IOException {
buffer.append(this.accessor(attributes).print(context.get(this)));
}
@Override
public V parse(
CharSequence text,
ParsePosition status,
AttributeQuery attributes
) {
return this.accessor(attributes).parse(
text,
status,
this.getType(),
attributes
);
}
/**
* Liefert einen Zugriffsindex zur Optimierung der Elementsuche.
*
* @return int
*/
int getIndex() {
return this.index;
}
private TextAccessor accessor(AttributeQuery attributes) {
CalendarText cnames =
CalendarText.getInstance(
attributes.get(Attributes.CALENDAR_TYPE, ISO_CALENDAR_TYPE),
attributes.get(Attributes.LANGUAGE, Locale.ROOT));
TextWidth textWidth =
attributes.get(Attributes.TEXT_WIDTH, TextWidth.WIDE);
switch (this.index) {
case MONTH:
return cnames.getStdMonths(
textWidth,
attributes.get(
Attributes.OUTPUT_CONTEXT,
OutputContext.FORMAT));
case DAY_OF_WEEK:
return cnames.getWeekdays(
textWidth,
attributes.get(
Attributes.OUTPUT_CONTEXT,
OutputContext.FORMAT));
case QUARTER_OF_YEAR:
return cnames.getQuarters(
textWidth,
attributes.get(
Attributes.OUTPUT_CONTEXT,
OutputContext.FORMAT));
default:
throw new UnsupportedOperationException(this.name());
}
}
private Object readResolve() throws ObjectStreamException {
Object element = PlainDate.lookupElement(this.name());
if (element == null) {
throw new InvalidObjectException(this.name());
} else {
return element;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy