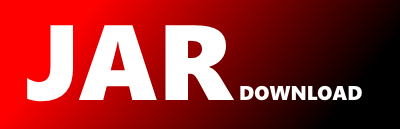
net.time4j.FormatSupport Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2015 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (FormatSupport.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j;
import net.time4j.engine.ChronoEntity;
import net.time4j.format.ChronoPattern;
import net.time4j.format.DisplayMode;
import net.time4j.format.FormatEngine;
import net.time4j.format.TemporalFormatter;
import net.time4j.tz.TZID;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Locale;
import java.util.ServiceLoader;
/**
* Defines some helper routines for format support of basic types.
*
* @author Meno Hochschild
* @since 3.0
*/
class FormatSupport {
//~ Statische Felder/Initialisierungen --------------------------------
private static final FormatEngine> DEFAULT_FORMAT_ENGINE;
static {
FormatEngine> last = null;
FormatEngine> best = null;
ClassLoader cl = Thread.currentThread().getContextClassLoader();
if (cl == null) {
cl = FormatEngine.class.getClassLoader();
}
if (cl == null) {
cl = ClassLoader.getSystemClassLoader();
}
for (FormatEngine> tmp : ServiceLoader.load(FormatEngine.class, cl)) {
if (tmp.isSupported(ChronoEntity.class)) {
best = tmp;
break;
} else {
last = tmp;
}
}
if (best == null) {
if (last == null) {
best = Platform.PATTERN.getFormatEngine();
} else {
best = last;
}
}
DEFAULT_FORMAT_ENGINE = best;
}
//~ Konstruktoren -----------------------------------------------------
private FormatSupport() {
// no instantiation
}
//~ Methoden ----------------------------------------------------------
/**
* Erzeugt einen Formatierer für lokale Entitäten.
*
* @param generic type of associated chronological entities
* @param generic type of pattern
* @param chronoType chronological type
* @param formatPattern pattern defining the structure of formatter
* @param patternType type of pattern indicating the format engine
* @param locale language and country setting
* @return new temporal formatter
* @since 3.0
*/
static , P extends ChronoPattern> TemporalFormatter createFormatter(
Class chronoType,
String formatPattern,
P patternType,
Locale locale
) {
FormatEngine formatEngine = patternType.getFormatEngine();
return formatEngine.create(chronoType, formatPattern, patternType, locale);
}
/**
*
Erzeugt einen Formatierer für globale Entitäten.
*
* @param generic type of associated chronological entities
* @param generic type of pattern
* @param chronoType chronological type
* @param formatPattern pattern defining the structure of formatter
* @param patternType type of pattern indicating the format engine
* @param locale language and country setting
* @param tzid timezone id
* @return new temporal formatter
* @since 3.0
*/
static , P extends ChronoPattern> TemporalFormatter createFormatter(
Class chronoType,
String formatPattern,
P patternType,
Locale locale,
TZID tzid
) {
return createFormatter(chronoType, formatPattern, patternType, locale).withTimezone(tzid);
}
/**
* Erzeugt einen Formatierer für lokale Entitäten.
*
* @param generic type of associated chronological entities
* @param chronoType chronological type
* @param formatPattern format pattern
* @param locale language and country setting
* @return new temporal formatter
* @since 3.0
*/
static > TemporalFormatter createFormatter(
Class chronoType,
String formatPattern,
Locale locale
) {
return createFormatter(chronoType, DEFAULT_FORMAT_ENGINE, formatPattern, locale);
}
/**
* Erzeugt einen Formatierer für globale Entitäten.
*
* @param generic type of associated chronological entities
* @param chronoType chronological type
* @param formatPattern format pattern
* @param locale language and country setting
* @param tzid timezone id
* @return new temporal formatter
* @since 3.0
*/
static > TemporalFormatter createFormatter(
Class chronoType,
String formatPattern,
Locale locale,
TZID tzid
) {
return createFormatter(chronoType, DEFAULT_FORMAT_ENGINE, formatPattern, locale).withTimezone(tzid);
}
/**
* Hilfsmethode zum Konvertieren des Anzeigestils in eine
* {@code DateFormat}-Konstante.
*
* @param mode Anzeigestil von Time4J
* @return JDK-Anzeigestil
* @since 3.0
*/
static int getFormatStyle(DisplayMode mode) {
switch (mode) {
case FULL:
return DateFormat.FULL;
case LONG:
return DateFormat.LONG;
case MEDIUM:
return DateFormat.MEDIUM;
case SHORT:
return DateFormat.SHORT;
default:
throw new UnsupportedOperationException("Unknown: " + mode);
}
}
/**
* Extrahiert ein Formatmuster, wenn möglich.
*
* @param df JDK-DateFormat
* @return format pattern
* @throws IllegalStateException if format pattern cannot be determined
* @since 3.0
*/
static String getFormatPattern(DateFormat df) {
if (df instanceof SimpleDateFormat) {
return SimpleDateFormat.class.cast(df).toPattern();
}
throw new IllegalStateException("Cannot retrieve format pattern.");
}
/**
* Yields the best available format engine.
*
* @return format engine
* @since 3.0
*/
static FormatEngine> getDefaultFormatEngine() {
return DEFAULT_FORMAT_ENGINE;
}
private static , P extends ChronoPattern> TemporalFormatter createFormatter(
Class chronoType,
FormatEngine formatEngine,
String formatPattern,
Locale locale
) {
return formatEngine.create(chronoType, formatPattern, formatEngine.getDefaultPatternType(), locale);
}
}