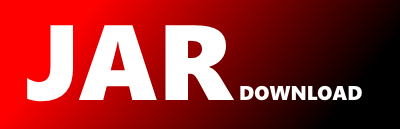
net.time4j.engine.BasicUnit Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (BasicUnit.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.engine;
/**
* Abstract time unit class which can define its own rule.
*
* @see TimePoint#plus(long, Object) TimePoint.plus(long, U)
* @see TimePoint#minus(long, Object) TimePoint.minus(long, U)
* @see TimePoint#until(TimePoint, Object) TimePoint.until(T, U)
*/
/*[deutsch]
* Abstrakte Zeiteinheitsklasse, die ihre eigene Regel definieren kann.
*
* @see TimePoint#plus(long, Object) TimePoint.plus(long, U)
* @see TimePoint#minus(long, Object) TimePoint.minus(long, U)
* @see TimePoint#until(TimePoint, Object) TimePoint.until(T, U)
*/
public abstract class BasicUnit
implements ChronoUnit {
//~ Konstruktoren -----------------------------------------------------
/**
* Subclasses usually define a singleton and have no public
* constructors.
*/
/*[deutsch]
* Subklassen werden normalerweise ein Singleton definieren und
* keine öffentlichen Konstruktoren anbieten.
*/
protected BasicUnit() {
super();
}
//~ Methoden ----------------------------------------------------------
@Override
public boolean isCalendrical() {
return (Double.compare(this.getLength(), 86400.0) >= 0);
}
/**
* Derives an optional unit rule for given chronology.
*
* This implementation yields {@code null} so that a chronology
* must register this unit. Specialized subclasses can define a rule
* however if the chronology has not registered this unit.
*
* @param generic type of time context
* @param chronology chronology an unit rule is searched for
* @return unit rule or {@code null} if given chronology is
* not supported
*/
/*[deutsch]
* Leitet eine optionale Einheitsregel für die angegebene
* Chronologie ab.
*
* Diese Implementierung liefert {@code null}, so daß eine
* gegebene Chronologie diese Einheit registrieren muß.
* Spezialisierte Subklassen können jedoch eine Regel definieren,
* wenn eine Chronologie diese Einheit nicht registriert hat.
*
* @param generic type of time context
* @param chronology chronology an unit rule is searched for
* @return unit rule or {@code null} if given chronology is
* not supported
*/
protected > UnitRule derive(
Chronology chronology
) {
return null;
}
}