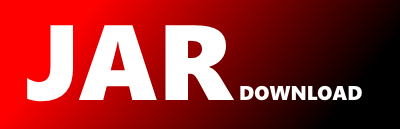
net.time4j.engine.ChronoUnit Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (ChronoUnit.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.engine;
/**
* External time units which are not registered on any chronology (time axis)
* can implement this interface in order to support standard calculations in
* time spans and symbol formatting.
*
* Naming convention: Time units have Java-names in
* plural form.
*
* @author Meno Hochschild
* @doctags.spec Implementations must be always immutable.
*/
/*[deutsch]
* Externe Zeiteinheiten, die nicht in einer Chronologie (Zeitachse)
* registriert sind, können dieses Interface implementieren, um
* Standardberechnungen in Zeitspannen und Symbolformatierungen zu
* unterstützen.
*
* Namenskonvention: Zeiteinheiten haben Java-Namen in
* Pluralform.
*
* @author Meno Hochschild
* @doctags.spec Implementations must be always immutable.
*/
public interface ChronoUnit {
//~ Methoden ----------------------------------------------------------
/**
* Defines the typical length of this time unit in seconds without
* taking into account anomalies like timezone effects or leap seconds.
*
* Important note: This method can only yield an
* estimated value and is not intended to assist in calculations of
* durations, but only in sorting of units.
*
* @return estimated decimal value in seconds
*/
/*[deutsch]
* Definiert die typische Länge dieser Zeiteinheit in Sekunden
* ohne Berücksichtigung von Anomalien wie Zeitzoneneffekten oder
* Schaltsekunden.
*
* Wichtiger Hinweis: Diese Methode kann nur einen
* Schätzwert liefern und ist für die Berechnung einer jedweden
* Dauer ungeeignet. Stattdessen darf und soll die Methode zum Sortieren
* von Zeiteinheiten verwendet werden.
*
* @return estimated decimal value in seconds
*/
double getLength();
/**
* Queries if this time unit is calendrical respective is at least
* as long as a calendar day.
*
* Implementation note: The method must be consistent with the typical
* length of the unit. The expression
* {@code Double.compare(unit.getLength(), 86400.0) >= 0} is
* equivalent to {@code unit.isCalendrical()}.
*
* @return {@code true} if at least as long as a day else {@code false}
*/
/*[deutsch]
/**
* Ist diese Zeiteinheit kalendarisch beziehungsweise mindestens
* so lange wie ein Kalendertag?
*
* Implementierungshinweis: Die Methode muß konsistent
* mit der typischen Standardlänge sein. Der Ausdruck
* {@code Double.compare(unit.getLength(), 86400.0) >= 0} ist
* äquivalent zu {@code unit.isCalendrical()}.
*
* @return {@code true} if at least as long as a day else {@code false}
*/
boolean isCalendrical(); // TODO: Ab Java 8 (Time4J-2.0) default-Methode
}