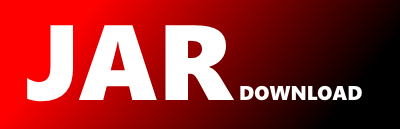
net.time4j.scale.LeapSecondProvider Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (LeapSecondProvider.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.scale;
import net.time4j.base.GregorianDate;
import java.util.Map;
/**
* This SPI-interface describes when
* UTC-leapseconds were introduced.
*
* Will be evaluated during loading of the class {@code LeapSeconds}.
* If any implementation defines no leapseconds then Time4J assumes
* that leapseconds will generally not be active, effectively resulting
* in POSIX-time instead of UTC.
*
* @author Meno Hochschild
* @doctags.spec All implementations must have a public no-arg constructor.
* @since 2.3
*/
/*[deutsch]
* Dieses SPI-Interface beschreibt, wann
* UTC-Schaltsekunden eingeführt worden sind.
*
* Wird beim Laden der Klasse {@code LeapSeconds} ausgewertet. Wenn
* eine Implementierung zum Beispiel per eigener Konfiguration keine
* Schaltsekunden definiert, so wird angenommen, daß generell
* keine Schaltsekunden verwendet werden sollen, also die POSIX-Zeit
* statt UTC.
*
* @author Meno Hochschild
* @doctags.spec All implementations must have a public no-arg constructor.
* @since 2.3
*/
public interface LeapSecondProvider {
//~ Methoden ----------------------------------------------------------
/**
* Yields all UTC-leapseconds with date and sign.
*
* The switch-over day in the UTC-timezone is considered as
* map key. The associated value is denotes the sign of the
* leapsecond. Is the value {@code +1} then it is a positive
* leapsecond. Is the value {@code -1} then it is a negative
* leapsecond. Other values are not supported.
*
* @return map from leap second event day to sign of leap second
* @since 2.0
*/
/*[deutsch]
* Liefert alle UTC-Schaltsekunden mit Datum und Vorzeichen.
*
* Als Schlüssel wird der Umstellungstag in der UTC-Zeitzone
* genommen. Der zugeordnete Wert bezeichnet das Vorzeichen der
* Schaltsekunde. Ist der Wert {@code +1}, dann handelt es sich um
* eine positive Schaltsekunde. Ist der Wert {@code -1}, dann wird
* eine negative Schaltsekunde angenommen. Andere Werte werden nicht
* unterstützt.
*
* @return map from leap second event day to sign of leap second
* @since 2.0
*/
Map getLeapSecondTable();
/**
* Queries if negative leapseconds are supported.
*
* Until now there has never been any negative leapseconds.
* As long as this is the case a {@code Provider} is allowed to
* return {@code false} in order to improve the performance.
*
* @return {@code true} if supported else {@code false}
* @since 2.0
*/
/*[deutsch]
* Werden auch negative Schaltsekunden unterstützt?
*
* Bis jetzt hat es real noch nie negative Schaltsekunden gegeben.
* Solange das der Fall ist, darf ein {@code Provider} aus
* Gründen der besseren Performance hier {@code false}
* zurückgeben.
*
* @return {@code true} if supported else {@code false}
* @since 2.0
*/
boolean supportsNegativeLS();
/**
* Creates the date of a leap second event.
*
* @param year proleptic gregorian year >= 1972
* @param month gregorian month
* @param dayOfMonth day of leap second switch
* @return immutable date of leap second event
* @since 2.3
*/
/*[deutsch]
* Erzeugt das Datum eines Schaltsekundenereignisses.
*
* @param year proleptic gregorian year >= 1972
* @param month gregorian month
* @param dayOfMonth day of leap second switch
* @return immutable date of leap second event
* @since 2.3
*/
GregorianDate getDateOfEvent(
int year,
int month,
int dayOfMonth
);
/**
* Determines the expiration date of underlying data.
*
* @return immutable date of expiration
* @since 2.3
*/
/*[deutsch]
* Bestimmt das Verfallsdatum der zugrundeliegenden Daten.
*
* @return immutable date of expiration
* @since 2.3
*/
GregorianDate getDateOfExpiration();
}