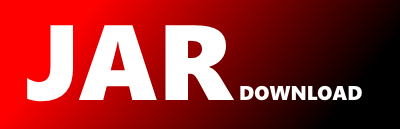
net.time4j.AdjustableElement Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (AdjustableElement.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j;
import net.time4j.engine.ChronoElement;
/**
* Extends a chronological element by some standard ways of
* manipulation.
*
* @param generic type of element values
* @param generic type of target entity an operator is applied to
* @author Meno Hochschild
*/
/*[deutsch]
* Erweitert ein chronologisches Element um diverse
* Standardmanipulationen.
*
* @param generic type of element values
* @param generic type of target entity an operator is applied to
* @author Meno Hochschild
*/
public interface AdjustableElement
extends ZonalElement {
//~ Methoden ----------------------------------------------------------
/**
* Sets any local entity to given new value of this element.
*
* @param value new element value
* @return operator directly applicable also on {@code PlainTimestamp}
* @since 2.0
* @see net.time4j.engine.ChronoEntity#with(ChronoElement,Object)
* ChronoEntity.with(ChronoElement, V)
*/
/*[deutsch]
* Setzt eine beliebige Entität auf den angegebenen Wert.
*
* @param value new element value
* @return operator directly applicable also on {@code PlainTimestamp}
* @since 2.0
* @see net.time4j.engine.ChronoEntity#with(ChronoElement,Object)
* ChronoEntity.with(ChronoElement, V)
*/
ElementOperator newValue(V value);
/**
* Sets any local entity to the minimum of this element.
*
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Setzt eine beliebige Entität auf das Elementminimum.
*
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator minimized();
/**
* Sets any local entity to the maximum of this element.
*
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Setzt eine beliebige Entität auf das Elementmaximum.
*
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator maximized();
/**
* Adjusts any local entity such that this element gets the previous
* value.
*
* The operator throws a {@code ChronoException} if there is no
* base unit available for this element.
*
* @return operator directly applicable also on {@code PlainTimestamp}
* and requiring a base unit in given chronology for decrementing
* @see net.time4j.engine.TimeAxis#getBaseUnit(ChronoElement)
*/
/*[deutsch]
* Passt eine beliebige Entität so an, daß dieses Element
* den vorherigen Wert bekommt.
*
* Der Operator wirft eine {@code ChronoException}, wenn er auf einen
* Zeitpunkt angewandt wird, dessen Zeitachse keine Basiseinheit zu diesem
* Element kennt.
*
* @return operator directly applicable also on {@code PlainTimestamp}
* and requiring a base unit in given chronology for decrementing
* @see net.time4j.engine.TimeAxis#getBaseUnit(ChronoElement)
*/
ElementOperator decremented();
/**
* Adjusts any local entity such that this element gets the next
* value.
*
* The operator throws a {@code ChronoException} if there is no
* base unit available for this element.
*
* @return operator directly applicable also on {@code PlainTimestamp}
* and requiring a base unit in given chronology for incrementing
* @see net.time4j.engine.TimeAxis#getBaseUnit(ChronoElement)
*/
/*[deutsch]
* Passt eine beliebige Entität so an, daß dieses Element
* den nächsten Wert bekommt.
*
* Der Operator wirft eine {@code ChronoException}, wenn er auf einen
* Zeitpunkt angewandt wird, dessen Zeitachse keine Basiseinheit zu diesem
* Element kennt.
*
* @return operator directly applicable also on {@code PlainTimestamp}
* and requiring a base unit in given chronology for incrementing
* @see net.time4j.engine.TimeAxis#getBaseUnit(ChronoElement)
*/
ElementOperator incremented();
/**
* Rounds down an entity by setting all child elements to minimum.
*
* @return operator directly applicable on local types without timezone
*/
/*[deutsch]
* Rundet eine Entität ab, indem alle Kindselemente dieses
* Elements auf ihr Minimum gesetzt werden.
*
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator atFloor();
/**
* Rounds up an entity by setting all child elements to maximum.
*
* @return operator directly applicable on local types without timezone
*/
/*[deutsch]
* Rundet eine Entität auf, indem alle Kindselemente dieses
* Elements auf ihr Maximum gesetzt werden.
*
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator atCeiling();
}