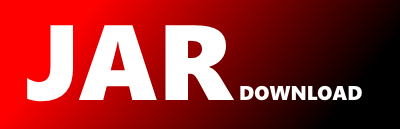
net.time4j.ElementOperator Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2015 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (ElementOperator.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j;
import net.time4j.engine.ChronoElement;
import net.time4j.engine.ChronoOperator;
import net.time4j.tz.TZID;
import net.time4j.tz.Timezone;
import net.time4j.tz.ZonalOffset;
/**
* Defines any manipulation of date or wall time objects following the
* strategy design pattern.
*
* @param generic target type this operator is applied to
* @author Meno Hochschild
*/
/*[deutsch]
* Definiert eine Manipulation von Datums- oder Uhrzeitobjekten nach
* dem Strategy-Entwurfsmuster.
*
* @param generic target type this operator is applied to
* @author Meno Hochschild
*/
public abstract class ElementOperator
implements ChronoOperator {
//~ Statische Felder/Initialisierungen --------------------------------
static final int OP_NEW_VALUE = -1;
static final int OP_MINIMIZE = 0;
static final int OP_MAXIMIZE = 1;
static final int OP_DECREMENT = 2;
static final int OP_INCREMENT = 3;
static final int OP_FLOOR = 4;
static final int OP_CEILING = 5;
static final int OP_LENIENT = 6;
static final int OP_WIM = 7;
static final int OP_YOW = 8;
static final int OP_NAV_NEXT = 9;
static final int OP_NAV_PREVIOUS = 10;
static final int OP_NAV_NEXT_OR_SAME = 11;
static final int OP_NAV_PREVIOUS_OR_SAME = 12;
static final int OP_ROUND_FULL_HOUR = 13;
static final int OP_ROUND_FULL_MINUTE = 14;
static final int OP_NEXT_FULL_HOUR = 15;
static final int OP_NEXT_FULL_MINUTE = 16;
static final int OP_FIRST_DAY_OF_NEXT_MONTH = 17;
static final int OP_FIRST_DAY_OF_NEXT_QUARTER = 18;
static final int OP_FIRST_DAY_OF_NEXT_YEAR = 19;
static final int OP_LAST_DAY_OF_PREVIOUS_MONTH = 20;
static final int OP_LAST_DAY_OF_PREVIOUS_QUARTER = 21;
static final int OP_LAST_DAY_OF_PREVIOUS_YEAR = 22;
//~ Instanzvariablen --------------------------------------------------
private final ChronoElement> element;
private final int type;
//~ Konstruktoren -----------------------------------------------------
/**
* Paket-privater Konstruktor.
*
* @param element element this operator will be applied on
* @param type operator type
*/
ElementOperator(
ChronoElement> element,
int type
) {
super();
this.element = element;
this.type = type;
}
//~ Methoden ----------------------------------------------------------
/**
* Creates an operator which can adjust a {@link Moment} in the
* system timezone.
*
* Note: Usually the operator converts the given {@code Moment} to
* a {@code PlainTimestamp} then processes this local timestamp and
* finally converts the result back to a new {@code Moment}. A special
* case are incrementing and decrementing of (sub-)second elements which
* eventually operate directly on the UTC timeline.
*
* @return operator with the default system timezone reference,
* applicable on instances of {@code Moment}
*/
/*[deutsch]
* Erzeugt einen Operator, der einen {@link Moment} mit
* Hilfe der Systemzeitzonenreferenz anpassen kann.
*
* Hinweis: Der Operator wandelt meist den gegebenen {@code Moment}
* in einen lokalen Zeitstempel um, bearbeitet dann diese lokale
* Darstellung und konvertiert das Ergebnis in einen neuen {@code Moment}
* zurück. Ein Spezialfall sind Inkrementierungen und Dekrementierungen
* von (Sub-)Sekundenelementen, bei denen ggf. direkt auf dem globalen
* Zeitstrahl operiert wird.
*
* @return operator with the default system timezone reference,
* applicable on instances of {@code Moment}
*/
public ChronoOperator inStdTimezone() {
return new Moment.Operator(this.onTimestamp(), this.element, this.type);
}
/**
* Creates an operator which can adjust a {@link Moment} in the
* given timezone.
*
* Note: Usually the operator converts the given {@code Moment} to
* a {@code PlainTimestamp} then processes this local timestamp and
* finally converts the result back to a new {@code Moment}. A special
* case are incrementing and decrementing of (sub-)second elements which
* eventually operate directly on the UTC timeline.
*
* @param tzid timezone id
* @return operator with the given timezone reference, applicable on
* instances of {@code Moment}
* @throws IllegalArgumentException if given timezone cannot be loaded
*/
/*[deutsch]
* Erzeugt einen Operator, der einen {@link Moment} mit
* Hilfe einer Zeitzonenreferenz anpassen kann.
*
* Hinweis: Der Operator wandelt meist den gegebenen {@code Moment}
* in einen lokalen Zeitstempel um, bearbeitet dann diese lokale
* Darstellung und konvertiert das Ergebnis in einen neuen {@code Moment}
* zurück. Ein Spezialfall sind Inkrementierungen und Dekrementierungen
* von (Sub-)Sekundenelementen, bei denen ggf. direkt auf dem globalen
* Zeitstrahl operiert wird.
*
* @param tzid timezone id
* @return operator with the given timezone reference, applicable on
* instances of {@code Moment}
* @throws IllegalArgumentException if given timezone cannot be loaded
*/
public final ChronoOperator inTimezone(TZID tzid) {
return new Moment.Operator(
this.onTimestamp(),
this.element,
this.type,
Timezone.of(tzid)
);
}
/**
* Creates an operator which can adjust a {@link Moment} in the
* given timezone.
*
* Note: Usually the operator converts the given {@code Moment} to
* a {@code PlainTimestamp} then processes this local timestamp and
* finally converts the result back to a new {@code Moment}. A special
* case are incrementing and decrementing of (sub-)second elements which
* eventually operate directly on the UTC timeline.
*
* @param tz timezone
* @return operator with the given timezone reference, applicable on
* instances of {@code Moment}
*/
/*[deutsch]
* Erzeugt einen Operator, der einen {@link Moment} mit
* Hilfe einer Zeitzonenreferenz anpassen kann.
*
* Hinweis: Der Operator wandelt meist den gegebenen {@code Moment}
* in einen lokalen Zeitstempel um, bearbeitet dann diese lokale
* Darstellung und konvertiert das Ergebnis in einen neuen {@code Moment}
* zurück. Ein Spezialfall sind Inkrementierungen und Dekrementierungen
* von (Sub-)Sekundenelementen, bei denen ggf. direkt auf dem globalen
* Zeitstrahl operiert wird.
*
* @param tz timezone
* @return operator with the given timezone reference, applicable on
* instances of {@code Moment}
*/
public final ChronoOperator in(Timezone tz) {
if (tz == null) {
throw new NullPointerException("Missing timezone.");
}
return new Moment.Operator(
this.onTimestamp(),
this.element,
this.type,
tz
);
}
/**
* Equivalent to {@code at(ZonalOffset.UTC)}.
*
* @return operator for UTC+00:00, applicable on instances
* of {@code Moment}
* @since 1.2
* @see #at(ZonalOffset)
*/
/*[deutsch]
* Äquivalent zu {@code at(ZonalOffset.UTC)}.
*
* @return operator for UTC+00:00, applicable on instances
* of {@code Moment}
* @since 1.2
* @see #at(ZonalOffset)
*/
public final ChronoOperator atUTC() {
return this.at(ZonalOffset.UTC);
}
/**
* Creates an operator which can adjust a {@link Moment} at the
* given timezone offset.
*
* Note: Usually the operator converts the given {@code Moment} to
* a {@code PlainTimestamp} then processes this local timestamp and
* finally converts the result back to a new {@code Moment}. A special
* case are incrementing and decrementing of (sub-)second elements which
* eventually operate directly on the UTC timeline.
*
* @param offset timezone offset
* @return operator with the given timezone offset, applicable on
* instances of {@code Moment}
* @since 1.2
*/
/*[deutsch]
* Erzeugt einen Operator, der einen {@link Moment} mit
* Hilfe eines Zeitzonen-Offsets anpassen kann.
*
* Hinweis: Der Operator wandelt meist den gegebenen {@code Moment}
* in einen lokalen Zeitstempel um, bearbeitet dann diese lokale
* Darstellung und konvertiert das Ergebnis in einen neuen {@code Moment}
* zurück. Ein Spezialfall sind Inkrementierungen und Dekrementierungen
* von (Sub-)Sekundenelementen, bei denen ggf. direkt auf dem globalen
* Zeitstrahl operiert wird.
*
* @param offset timezone offset
* @return operator with the given timezone offset, applicable on
* instances of {@code Moment}
* @since 1.2
*/
public final ChronoOperator at(ZonalOffset offset) {
return new Moment.Operator(
this.onTimestamp(),
this.element,
this.type,
Timezone.of(offset)
);
}
/**
* Liefert eine Operatorvariante für den
* {@code PlainTimestamp}-Kontext.
*
* @return cached operator
*/
abstract ChronoOperator onTimestamp();
/**
* Liefert das interne Element.
*
* @return element reference
*/
ChronoElement> getElement() {
return this.element;
}
/**
* Liefert den Operatortyp.
*
* @return type of this operator
*/
int getType() {
return this.type;
}
}