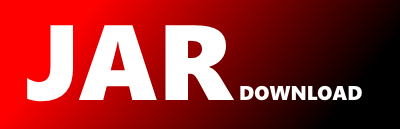
net.time4j.OrdinalWeekdayElement Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (OrdinalWeekdayElement.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j;
/**
* The element for the ordinal weekday in month.
*
* An instance can be obtained using the expression
* {@link PlainDate#WEEKDAY_IN_MONTH}. This interface inherits from
* {@code AdjustableElement} and offers additional operator for setting
* the weekday in month.
*
* @author Meno Hochschild
*/
/*[deutsch]
* Das Element für den x-ten Wochentag im Monat.
*
* Eine Instanz ist erhältlich über den Ausdruck
* {@link PlainDate#WEEKDAY_IN_MONTH}. Dieses Interface bietet neben
* den vom Interface {@code AdjustableElement} geerbten Methoden
* weitere Spezialmethoden zum Setzen des Wochentags im Monat.
*
* @author Meno Hochschild
*/
public interface OrdinalWeekdayElement
extends AdjustableElement {
//~ Methoden ----------------------------------------------------------
/**
* Defines an operator which moves a date to the first given weekday
* in month.
*
* @param dayOfWeek first day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Definiert einen Versteller, der ein Datum auf den ersten angegebenen
* Wochentag eines Monats setzt.
*
* @param dayOfWeek first day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToFirst(Weekday dayOfWeek);
/**
* Defines an operator which moves a date to the second given weekday
* in month.
*
* @param dayOfWeek second day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Definiert einen Versteller, der ein Datum auf den zweiten angegebenen
* Wochentag eines Monats setzt.
*
* @param dayOfWeek second day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToSecond(Weekday dayOfWeek);
/**
* Defines an operator which moves a date to the third given weekday
* in month.
*
* @param dayOfWeek third day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Definiert einen Versteller, der ein Datum auf den dritten angegebenen
* Wochentag eines Monats setzt.
*
* @param dayOfWeek third day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToThird(Weekday dayOfWeek);
/**
* Defines an operator which moves a date to the fourth given weekday
* in month.
*
* @param dayOfWeek fourth day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Definiert einen Versteller, der ein Datum auf den vierten angegebenen
* Wochentag eines Monats setzt.
*
* @param dayOfWeek fourth day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToFourth(Weekday dayOfWeek);
/**
* Defines an operator which moves a date to the last given weekday
* in month.
*
* @param dayOfWeek last day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Definiert einen Versteller, der ein Datum auf den letzten angegebenen
* Wochentag eines Monats setzt.
*
* @param dayOfWeek last day of week in month
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToLast(Weekday dayOfWeek);
}