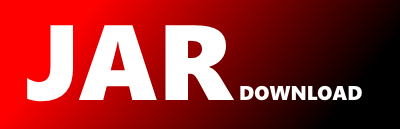
net.time4j.base.WallTime Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (WallTime.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.base;
/**
* Defines a wall time on an analogue clock conforming to ISO-8601
* in the range {@code T00:00:00 - T24:00:00}.
*
* Note: Implementations must document if they support the special value
* T24:00:00 or not. This value denotes midnight at the end of the day, that
* is midnight T00:00 at the begin of the following day.
*
* @author Meno Hochschild
*/
/*[deutsch]
* Definiert eine ISO-konforme Uhrzeit auf einer analogen Uhr im Bereich
* {@code T00:00:00 - T24:00:00}.
*
* Anmerkung: Implementierungen müssen dokumentieren, ob sie den
* Spezialwert T24:00:00 unterstützen oder nicht. Dieser Wert bezeichnet
* Mitternacht zum Ende eines Tages, also Mitternacht T00:00:00 zum Beginn
* des Folgetags.
*
* @author Meno Hochschild
*/
public interface WallTime {
//~ Methoden ----------------------------------------------------------
/**
* Yields the hour of day.
*
* @return hour in range {@code 0 - 24} (the value {@code 24} is only
* allowed if minute and second have the value {@code 0})
*/
/*[deutsch]
* Liefert die Stunde des Tages.
*
* @return hour in range {@code 0 - 24} (the value {@code 24} is only
* allowed if minute and second have the value {@code 0})
*/
int getHour();
/**
* Yields the minute of hour.
*
* @return minute in range {@code 0 - 59}
*/
/**
* Liefert die Minute (der aktuellen Stunde).
*
* @return minute in range {@code 0 - 59}
*/
int getMinute();
/**
* Yields the second of minute.
*
* Given this context and the fact that this interface describes
* an analogue clock without UTC reference, the special leapsecond
* value {@code 60} cannot be supported.
*
* @return second in range {@code 0 - 59}
*/
/*[deutsch]
* Liefert die Sekunde.
*
* Weil dieses Interface eine analoge Uhr ohne UTC-Bezug beschreibt,
* kann in diesem Kontext der spezielle Sekundenwert {@code 60} nicht
* unterstützt werden.
*
* @return second in range {@code 0 - 59}
*/
int getSecond();
/**
* Yields the nanosecond.
*
* Implementations which are not capable of nanosecond precision
* will just yield {@code 0} or another suitable rounded value.
*
* @return nanosecond in range {@code 0 - 999,999,999}
*/
/*[deutsch]
* Liefert die Nanosekunde.
*
* Implementierungen, die keine Nanosekundengenauigkeit haben,
* werden einfach {@code 0} oder einen anderen gerundeten Wert
* liefern.
*
* @return Nanosekunde im Bereich {@code 0 - 999.999.999}
*/
int getNanosecond();
/**
* Yields a canonical representation in ISO-format
* "Thh:mm" or "Thh:mm:ss".
*
* If this object also knows subseconds then a fractional part
* of second part is possible.
*
* @return wall time in ISO-8601 format
*/
/*[deutsch]
* Liefert eine kanonische Darstellung im ISO-Format
* "Thh:mm" oder "Thh:mm:ss".
*
* Falls dieses Objekt auch Subsekunden kennt, kann eine fraktionale
* Anzeige des Sekundenteils folgen.
*
* @return wall time in ISO-8601 format
*/
@Override
String toString();
}