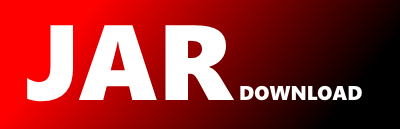
net.time4j.format.WeekdataProvider Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2015 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (WeekdataProvider.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.format;
import java.util.Locale;
/**
* This SPI-interface enables the access to localized
* week rules and is instantiated via a {@code ServiceLoader}-mechanism.
*
* If there is no external {@code WeekdataProvider} then Time4J will use
* an internal implementation which is based on all informations contained
* in the JDK and also the CLDR-data of unicode consortium. Especially
* the data which define a weekend will be preferably read from the resource
* file "data/weekend.data".
*
* Specification:
* Implementations must have a public no-arg constructor.
*
* @author Meno Hochschild
* @see java.util.ServiceLoader
*/
/*[deutsch]
* Dieses SPI-Interface ermöglicht den Zugriff
* auf {@code Locale}-abhängige Wochenregeln und wird über einen
* {@code ServiceLoader}-Mechanismus instanziert.
*
* Wird kein externer {@code WeekdataProvider} gefunden, wird intern
* eine Instanz erzeugt, die auf den im JDK enthaltenen Informationen und
* auf den CLDR-Daten des Unicode-Konsortiums beruht. Speziell die ein
* Wochenende definierenden Daten werden bevorzugt aus der Textdatei
* "data/weekend.data" geladen.
*
* Specification:
* Implementations must have a public no-arg constructor.
*
* @author Meno Hochschild
* @see java.util.ServiceLoader
*/
public interface WeekdataProvider {
//~ Methoden ----------------------------------------------------------
/**
* Defines the first day of a calendar week.
*
* @param country country or region
* @return weekday (Mon=1, Tue=2, Wed=3, Thu=4, Fri=5, Sat=6, Sun=7)
*/
/*[deutsch]
* Definiert den ersten Tag einer Kalenderwoche.
*
* @param country Länderangabe
* @return Wochentag (Mo=1, Di=2, Mi=3, Do=4, Fr=5, Sa=6, So=7)
*/
int getFirstDayOfWeek(Locale country);
/**
* Defines the minimum count of days which the first calendar week
* of the year or month must contain.
*
* @param country country or region
* @return int in range {@code 1 - 7}
*/
/*[deutsch]
* Definiert die minimale Anzahl von Tagen, die die erste
* Kalenderwoche eines Jahres oder Monats enthalten muß.
*
* @param country Länderangabe
* @return Anzahl im Bereich {@code 1 - 7}
*/
int getMinimalDaysInFirstWeek(Locale country);
/**
* Defines the first day of weekend.
*
* @param country country or region
* @return weekday (Mon=1, Tue=2, Wed=3, Thu=4, Fri=5, Sat=6, Sun=7)
*/
/*[deutsch]
* Definiert den ersten Tag des Wochenendes.
*
* @param country Länderangabe
* @return Wochentag (Mo=1, Di=2, Mi=3, Do=4, Fr=5, Sa=6, So=7)
*/
int getStartOfWeekend(Locale country);
/**
* Defines the last day of weekend.
*
* @param country country or region
* @return weekday (Mon=1, Tue=2, Wed=3, Thu=4, Fri=5, Sat=6, Sun=7)
*/
/*[deutsch]
* Definiert den letzten Tag des Wochenendes.
*
* @param country Länderangabe
* @return Wochentag (Mo=1, Di=2, Mi=3, Do=4, Fr=5, Sa=6, So=7)
*/
int getEndOfWeekend(Locale country);
}