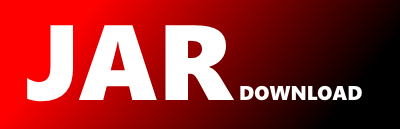
net.time4j.tz.TransitionStrategy Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2015 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (TransitionStrategy.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.tz;
import net.time4j.base.GregorianDate;
import net.time4j.base.WallTime;
/**
* Serves for resolving of local timestamps to a global UNIX timestamp,
* escpecially if there are conflicts due to gaps or overlaps on the local
* timeline.
*
* Specification:
* All implementations must be immutable, thread-safe and serializable.
*
* @author Meno Hochschild
*/
/*[deutsch]
* Dient der Auflösung von lokalen Zeitangaben zu einer UTC-Weltzeit,
* wenn wegen Lücken oder Überlappungen auf dem lokalen Zeitstrahl
* Konflikte auftreten.
*
* Specification:
* All implementations must be immutable, thread-safe and serializable.
*
* @author Meno Hochschild
*/
public interface TransitionStrategy {
//~ Methoden ----------------------------------------------------------
/**
* Calculates a suitable global timestamp for given local timestamp.
*
* The nanosecond fraction of given wall time will not be taken
* into account.
*
* @param localDate local calendar date in given timezone
* @param localTime local wall time in given timezone
* @param timezone timezone data containing offset history
* @return global timestamp as full seconds since UNIX epoch (posix time)
* @since 1.2.1
* @see net.time4j.scale.TimeScale#POSIX
* @see net.time4j.PlainTimestamp#in(Timezone)
* @see Timezone#with(TransitionStrategy)
*/
/*[deutsch]
* Bestimmt einen geeigneten globalen Zeitstempel für eine
* lokale Zeitangabe.
*
* Der Nanosekundenteil der angegebenen Uhrzeit bleibt
* unberücksichtigt.
*
* @param localDate local calendar date in given timezone
* @param localTime local wall time in given timezone
* @param timezone timezone data containing offset history
* @return global timestamp as full seconds since UNIX epoch (posix time)
* @since 1.2.1
* @see net.time4j.scale.TimeScale#POSIX
* @see net.time4j.PlainTimestamp#in(Timezone)
* @see Timezone#with(TransitionStrategy)
*/
long resolve(
GregorianDate localDate,
WallTime localTime,
Timezone timezone
);
/**
* Calculates a suitable offset for given local timestamp.
*
* @param localDate local calendar date in given timezone
* @param localTime local wall time in given timezone
* @param timezone timezone data containing offset history
* @return ZonalOffset
* @since 1.2.1
* @see net.time4j.PlainTimestamp#in(Timezone)
* @see Timezone#with(TransitionStrategy)
*/
/*[deutsch]
* Bestimmt einen geeigneten Offset für eine lokale Zeitangabe.
*
* @param localDate local calendar date in given timezone
* @param localTime local wall time in given timezone
* @param timezone timezone data containing offset history
* @return ZonalOffset
* @since 1.2.1
* @see net.time4j.PlainTimestamp#in(Timezone)
* @see Timezone#with(TransitionStrategy)
*/
ZonalOffset getOffset(
GregorianDate localDate,
WallTime localTime,
Timezone timezone
);
}