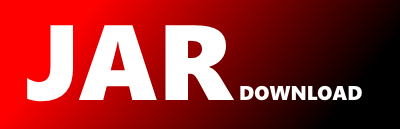
net.time4j.NavigableElement Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (NavigableElement.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j;
/**
* Defines additional enum-based operators for setting new element values
* taking into account the old element value.
*
* @param generic enum type of element values
* @author Meno Hochschild
*/
/*[deutsch]
* Definiert weitere enum-basierte Operatoren zum gezielten Setzen eines
* neuen Elementwerts unter Berücksichtigung des aktuellen Werts.
*
* @param generic enum type of element values
* @author Meno Hochschild
*/
public interface NavigableElement>
extends AdjustableElement {
//~ Methoden ----------------------------------------------------------
/**
* Moves a time point to the first given element value which is after
* the current element value.
*
* Example for a date which shall be moved to a special weekday:
*
*
* import static net.time4j.Month.MARCH;
* import static net.time4j.PlainDate.DAY_OF_WEEK;
* import static net.time4j.Weekday.MONDAY;
*
* PlainDate date = PlainDate.of(2013, MARCH, 7); // Thursday
* System.out.println(date.with(DAY_OF_WEEK.setToNext(MONDAY)));
* // output: 2013-03-11 (first monday after march 7th)
*
*
* @param value new element value which is after current value
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Setzt einen Zeitpunkt auf den ersten angegebenen Wert, der nach dem
* aktuellen Wert liegt.
*
* Beispiel für ein Datum, das auf einen bestimmten Wochentag
* gesetzt werden soll:
*
*
* import static net.time4j.Month.MARCH;
* import static net.time4j.PlainDate.DAY_OF_WEEK;
* import static net.time4j.Weekday.MONDAY;
*
* PlainDate date = PlainDate.of(2013, MARCH, 7); // Donnerstag
* System.out.println(date.with(DAY_OF_WEEK.setToNext(MONDAY)));
* // Ausgabe: 2013-03-11 (erster Montag nach dem 7. März)
*
*
* @param value new element value which is after current value
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToNext(V value);
/**
* Moves a time point to the first given element value which is before
* the current element value.
*
* Example for a date which shall be moved to a special weekday:
*
*
* import static net.time4j.IsoElement.DAY_OF_WEEK;
* import static net.time4j.Month.MARCH;
* import static net.time4j.Weekday.THURSDAY;
*
* PlainDate date = PlainDate.of(2013, MARCH, 7); // Thursday
* System.out.println(date.with(DAY_OF_WEEK.setToPrevious(THURSDAY)));
* // output: 2013-02-28 (Thursday one week earlier)
*
*
* @param value new element value which is before current value
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Setzt einen Zeitpunkt auf den ersten angegebenen Wert, der vor dem
* aktuellen Wert liegt.
*
* Beispiel für ein Datum, das auf einen bestimmten Wochentag
* gesetzt werden soll:
*
*
* import static net.time4j.IsoElement.DAY_OF_WEEK;
* import static net.time4j.Month.MARCH;
* import static net.time4j.Weekday.THURSDAY;
*
* PlainDate date = PlainDate.of(2013, MARCH, 7); // Donnerstag
* System.out.println(date.with(DAY_OF_WEEK.setToPrevious(THURSDAY)));
* // Ausgabe: 2013-02-28 (Donnerstag eine Woche früher)
*
*
* @param value new element value which is before current value
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToPrevious(V value);
/**
* Moves a time point to the first given element value which is after
* or equal to the current element value.
*
* Is the current element value equal to the given element value then
* there is no movement.
*
* @param value new element value which is either after current value
* or the same
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Setzt einen Zeitpunkt auf den ersten angegebenen Wert setzt, der nach
* oder gleich dem aktuellen Wert liegt.
*
* Ist der aktuelle Wert gleich dem angegebenen Wert, gibt es keine
* Verschiebung des Zeitpunkts.
*
* @param value new element value which is either after current value
* or the same
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToNextOrSame(V value);
/**
* Moves a time point to the first given element value which is before
* or equal to the current element value.
*
* Is the current element value equal to the given element value then
* there is no movement.
*
* @param value new element value which is either before current value
* or the same
* @return operator directly applicable also on {@code PlainTimestamp}
*/
/*[deutsch]
* Setzt einen Zeitpunkt auf den ersten angegebenen Wert, der vor oder
* gleich dem aktuellen Wert liegt.
*
* Ist der aktuelle Wert gleich dem angegebenen Wert, gibt es keine
* Verschiebung des Zeitpunkts.
*
* @param value new element value which is either before current value
* or the same
* @return operator directly applicable also on {@code PlainTimestamp}
*/
ElementOperator setToPreviousOrSame(V value);
}