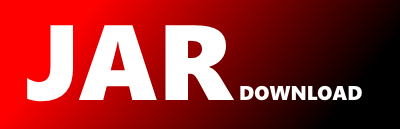
net.time4j.Quarter Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2016 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (Quarter.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j;
import net.time4j.base.GregorianDate;
import net.time4j.engine.ChronoCondition;
import net.time4j.format.CalendarText;
import net.time4j.format.OutputContext;
import net.time4j.format.TextWidth;
import java.util.Locale;
/**
* Represents a quarter (in most cases of a year).
*
* @author Meno Hochschild
*/
/*[deutsch]
* Repräsentiert ein Quartal (meist eines Jahres).
*
* @author Meno Hochschild
*/
public enum Quarter
implements ChronoCondition {
//~ Statische Felder/Initialisierungen --------------------------------
/**
* First quarter with the numerical value {@code 1}.
*/
/*[deutsch]
* Erstes Quartal mit dem numerischen Wert {@code 1}.
*/
Q1,
/**
* Second quarter with the numerical value {@code 2}.
*/
/*[deutsch]
* Zweites Quartal mit dem numerischen Wert {@code 2}.
*/
Q2,
/**
* Third quarter with the numerical value {@code 3}.
*/
/*[deutsch]
* Drittes Quartal mit dem numerischen Wert {@code 3}.
*/
Q3,
/**
* Last quarter with the numerical value {@code 4}.
*/
/*[deutsch]
* Letztes Quartal mit dem numerischen Wert {@code 4}.
*/
Q4;
private static final Quarter[] ENUMS = Quarter.values(); // Cache
//~ Methoden ----------------------------------------------------------
/**
* Gets the enum-constant which corresponds to the given numerical
* value.
*
* @param quarter value in the range [1-4]
* @return enum
* @throws IllegalArgumentException if given argument is out of range
*/
/*[deutsch]
* Liefert die zum chronologischen Integer-Wert passende
* Enum-Konstante.
*
* @param quarter value in the range [1-4]
* @return enum
* @throws IllegalArgumentException if given argument is out of range
*/
public static Quarter valueOf(int quarter) {
if ((quarter < 1) || (quarter > 4)) {
throw new IllegalArgumentException("Out of range: " + quarter);
}
return ENUMS[quarter - 1];
}
/**
* Gets the corresponding numerical value.
*
* @return int (Q1 = 1, Q2 = 2, Q3 = 3, Q4 = 4)
*/
/*[deutsch]
* Liefert den korrespondierenden chronologischen Integer-Wert.
*
* @return int (Q1 = 1, Q2 = 2, Q3 = 3, Q4 = 4)
*/
public int getValue() {
return (this.ordinal() + 1);
}
/**
* Rolls to the next quarter.
*
* The result is {@code Q1} if this method is applied on {@code Q4}.
*
* @return next quarter rolling at last quarter
*/
/*[deutsch]
* Ermittelt das nächste Quartal.
*
* Auf {@code Q4} angewandt ist das Ergebnis {@code Q1}.
*
* @return next quarter rolling at last quarter
*/
public Quarter next() {
return this.roll(1);
}
/**
* Rolls to the previous quarter.
*
* The result is {@code Q4} if this method is applied on {@code Q1}.
*
* @return previous quarter rolling at first quarter
*/
/*[deutsch]
* Ermittelt das vorherige Quartal.
*
* Auf {@code Q1} angewandt ist das Ergebnis {@code Q4}.
*
* @return previous quarter rolling at first quarter
*/
public Quarter previous() {
return this.roll(-1);
}
/**
* Rolls by given amount of quarters.
*
* @param quarters count of quarters (maybe negative)
* @return result of rolling operation
*/
/*[deutsch]
* Rollt um die angegebene Anzahl von Quartalen vor oder
* zurück.
*
* @param quarters count of quarters (maybe negative)
* @return result of rolling operation
*/
public Quarter roll(int quarters) {
return Quarter.valueOf(
(this.ordinal() + (quarters % 4 + 4)) % 4 + 1);
}
/**
* Equivalent to the expression
* {@code getDisplayName(locale, TextWidth.WIDE, OutputContext.FORMAT)}.
*
*
* @param locale language setting
* @return descriptive text (long form, never {@code null})
* @see #getDisplayName(Locale, TextWidth, OutputContext)
*/
/*[deutsch]
* Entspricht dem Ausdruck
* {@code getDisplayName(locale, TextWidth.WIDE, OutputContext.FORMAT)}.
*
*
* @param locale language setting
* @return descriptive text (long form, never {@code null})
* @see #getDisplayName(Locale, TextWidth, OutputContext)
*/
public String getDisplayName(Locale locale) {
return this.getDisplayName(
locale, TextWidth.WIDE, OutputContext.FORMAT);
}
/**
* Gets the description text dependent on the locale and style
* parameters.
*
* The second argument controls the width of description while the
* third argument is only relevant for languages which make a difference
* between stand-alone forms and embedded text forms (does not matter in
* English).
*
* @param locale language setting
* @param width text width
* @param context output context
* @return descriptive text for given locale and style (never {@code null})
*/
/*[deutsch]
* Liefert den sprachabhängigen Beschreibungstext.
*
* Über das zweite Argument kann gesteuert werden, ob eine kurze
* oder eine lange Form des Beschreibungstexts ausgegeben werden soll. Das
* ist besonders sinnvoll in Benutzeroberflächen, wo zwischen der
* Beschriftung und der detaillierten Erläuterung einer graphischen
* Komponente unterschieden wird. Das dritte Argument ist in Sprachen von
* Belang, die verschiedene grammatikalische Formen für die Ausgabe
* als alleinstehend oder eingebettet in formatierten Text kennen.
*
* @param locale language setting
* @param width text width
* @param context output context
* @return descriptive text for given locale and style (never {@code null})
*/
public String getDisplayName(
Locale locale,
TextWidth width,
OutputContext context
) {
return CalendarText.getIsoInstance(locale).getQuarters(width, context).print(this);
}
@Override
public boolean test(GregorianDate context) {
int month = context.getMonth();
return (this.getValue() == ((month - 1) / 3) + 1);
}
}