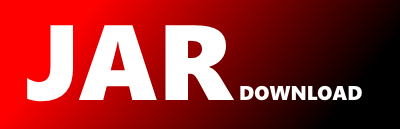
net.time4j.WallTimeElement Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (WallTimeElement.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j;
/**
* Represents the wall time.
*
* Defines additional operators for moving a timestamp to a new wall time
* possibly changing the day.
*
* @author Meno Hochschild
* @since 1.2
*/
/*[deutsch]
* Repräsentiert die Uhrzeit.
*
* Definiert weitere Operatoren, die einen Zeitstempel zu einer neuen
* Uhrzeit bewegen und bei Bedarf den Tag wechseln.
*
* @author Meno Hochschild
* @since 1.2
*/
public interface WallTimeElement
extends ZonalElement {
//~ Methoden ----------------------------------------------------------
/**
* Moves a timestamp to the next given wall time and change the day
* if necessary.
*
* @param value new wall time which is after current wall time
* @return operator directly applicable on {@code PlainTimestamp}
* @since 1.2
* @see PlainTimestamp#with(ElementOperator)
*/
/*[deutsch]
* Setzt einen Zeitpunkt auf die nächste angegebene Uhrzeit und
* wechselt bei Bedarf den Tag.
*
* @param value new wall time which is after current wall time
* @return operator directly applicable on {@code PlainTimestamp}
* @since 1.2
* @see PlainTimestamp#with(ElementOperator)
*/
ElementOperator> setToNext(PlainTime value);
/**
* Moves a timestamp to the previous given wall time and change the day
* backwards if necessary.
*
* @param value new wall time which is before current wall time
* @return operator directly applicable on {@code PlainTimestamp}
* @since 1.2
* @see PlainTimestamp#with(ElementOperator)
*/
/*[deutsch]
* Setzt einen Zeitpunkt auf die vorherige angegebene Uhrzeit und
* wechselt bei Bedarf den Tag rückwärts.
*
* @param value new wall time which is before current wall time
* @return operator directly applicable on {@code PlainTimestamp}
* @since 1.2
* @see PlainTimestamp#with(ElementOperator)
*/
ElementOperator> setToPrevious(PlainTime value);
/**
* Moves a timestamp to the next or same given wall time and change
* the day if necessary.
*
* @param value new wall time which is not before current wall time
* @return operator directly applicable on {@code PlainTimestamp}
* @since 1.2
* @see PlainTimestamp#with(ElementOperator)
*/
/*[deutsch]
* Setzt einen Zeitpunkt auf die nächste oder gleiche angegebene
* Uhrzeit und wechselt bei Bedarf den Tag.
*
* @param value new wall time which is not before current wall time
* @return operator directly applicable on {@code PlainTimestamp}
* @since 1.2
* @see PlainTimestamp#with(ElementOperator)
*/
ElementOperator> setToNextOrSame(PlainTime value);
/**
* Moves a timestamp to the previous or same given wall time and
* change the day backwards if necessary.
*
* @param value new wall time which is not after current wall time
* @return operator directly applicable on {@code PlainTimestamp}
* @since 1.2
* @see PlainTimestamp#with(ElementOperator)
*/
/*[deutsch]
* Setzt einen Zeitpunkt auf die vorherige oder gleiche angegebene
* Uhrzeit und wechselt bei Bedarf den Tag rückwärts.
*
* @param value new wall time which is not after current wall time
* @return operator directly applicable on {@code PlainTimestamp}
* @since 1.2
* @see PlainTimestamp#with(ElementOperator)
*/
ElementOperator> setToPreviousOrSame(PlainTime value);
/**
* Performs rounding to full hour in half rounding mode.
*
* @return operator also applicable on {@code PlainTimestamp}
* @since 1.2
*/
/*[deutsch]
* Rundet kaufmännisch zur vollen Stunde.
*
* @return operator also applicable on {@code PlainTimestamp}
* @since 1.2
*/
ElementOperator roundedToFullHour();
/**
* Performs rounding to full minute in half rounding mode.
*
* @return operator also applicable on {@code PlainTimestamp}
* @since 1.2
*/
/*[deutsch]
* Rundet kaufmännisch zur vollen Minute.
*
* @return operator also applicable on {@code PlainTimestamp}
* @since 1.2
*/
ElementOperator roundedToFullMinute();
/**
* Adjusts to next full hour.
*
* @return operator also applicable on {@code PlainTimestamp}
* @since 1.2
*/
/*[deutsch]
* Verstellt zur nächsten vollen Stunde.
*
* @return operator also applicable on {@code PlainTimestamp}
* @since 1.2
*/
ElementOperator setToNextFullHour();
/**
* Adjusts to next full minute.
*
* @return operator also applicable on {@code PlainTimestamp}
* @since 1.2
*/
/*[deutsch]
* Verstellt zur nächsten vollen Minute.
*
* @return operator also applicable on {@code PlainTimestamp}
* @since 1.2
*/
ElementOperator setToNextFullMinute();
}