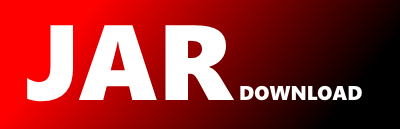
net.time4j.engine.ChronoDisplay Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2016 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (ChronoDisplay.java) is part of project Time4J.en
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.engine;
import net.time4j.tz.TZID;
/**
* Represents a view on a set of chronological elements associated
* with their temporal values.
*
* A {@code ChronoDisplay} serves mainly for formatted output.
*
* @author Meno Hochschild
* @since 2.0
*/
/*[deutsch]
* Repräsentiert ein Zeitwertobjekt, das einzelne Werte mit
* chronologischen Elementen assoziiert und einen Lesezugriff auf diese
* Werte erlaubt.
*
* Ein {@code ChronoDisplay} dient hauptsächlich der formatierten
* Darstellung.
*
* @author Meno Hochschild
* @since 2.0
*/
public interface ChronoDisplay {
//~ Methoden ----------------------------------------------------------
/**
* Queries if the value for given chronological element can be
* accessed via {@code get(element)}.
*
* If the argument is missing then this method will yield {@code false}.
* Note: Elements which are not registered but define a suitable rule
* are also accessible.
*
* @param element chronological element to be asked (optional)
* @return {@code true} if the element is registered or there is an element rule for evaluating the value
* else {@code false}
* @see #get(ChronoElement)
*/
/*[deutsch]
* Ist der Wert zum angegebenen chronologischen Element abfragbar
* beziehungsweise enthalten?
*
* Fehlt das Argument, dann liefert die Methode {@code false}. Zu
* beachten: Es werden hier nicht nur registrierte Elemente als abfragbar
* gewertet, sondern auch solche, die z.B. eine passende chronologische
* Regel definieren.
*
* @param element chronological element to be asked (optional)
* @return {@code true} if the element is registered or there is an element rule for evaluating the value
* else {@code false}
* @see #get(ChronoElement)
*/
boolean contains(ChronoElement> element);
/**
* Returns the partial value associated with given chronological
* element.
*
* @param generic type of element value
* @param element element which has the value
* @return associated element value as object (never {@code null})
* @throws ChronoException if there is no suitable element rule for evaluating the value
* or if the associated element value is not defined over the complete range of this instance
* @see #contains(ChronoElement)
*/
/*[deutsch]
* Fragt ein chronologisches Element nach seinem Wert als Objekt ab.
*
* @param generic type of element value
* @param element element which has the value
* @return associated element value as object (never {@code null})
* @throws ChronoException if there is no suitable element rule for evaluating the value
* or if the associated element value is not defined over the complete range of this instance
* @see #contains(ChronoElement)
*/
V get(ChronoElement element);
/**
* Returns the partial value associated with given chronological element.
*
* @param element element which has the value
* @return associated element value as int primitive or {@code Integer.MIN_VALUE} if not available
* @see #get(ChronoElement)
* @since 3.15/4.12
*/
/*[deutsch]
* Fragt ein chronologisches Element nach seinem Wert als Objekt ab.
*
* @param element element which has the value
* @return associated element value as int primitive or {@code Integer.MIN_VALUE} if not available
* @see #get(ChronoElement)
* @since 3.15/4.12
*/
default int getInt(ChronoElement element) {
try {
return this.get(element).intValue();
} catch (ChronoException ex) {
return Integer.MIN_VALUE;
}
}
/**
* Yields the minimum value of given chronological element in the
* current context of this object.
*
* The definition of a minimum and a maximum does generally not
* imply that every intermediate value between minimum and maximum
* is valid in this context. For example in the timezone Europe/Berlin
* the hour [T02:00] will be invalid if switching to summer time.
*
* In most cases the minimum value is not dependent on this
* context.
*
* @param generic type of element value
* @param element element whose minimum value is to be evaluated
* @return minimum maybe context-dependent element value
* @throws ChronoException if there is no suitable element rule for evaluating the minimum value
* or if the associated element value is not defined over the complete range of this instance
* @see ChronoElement#getDefaultMinimum()
* @see #getMaximum(ChronoElement)
*/
/*[deutsch]
* Ermittelt das Minimum des mit dem angegebenen chronologischen Element
* assoziierten Elementwerts.
*
* Die Definition eines Minimums und eines Maximums bedeutet im
* allgemeinen nicht, daß jeder Zwischenwert
* innerhalb des Bereichs im Kontext gültig sein muß. Zum
* Beispiel wird bei Sommerzeitumstellungen in der Zeitzone Europe/Berlin
* die Stunde [T02:00] komplett fehlen.
*
* Oft wird der Minimalwert von diesem Kontext unabhängig sein.
*
* @param generic type of element value
* @param element element whose minimum value is to be evaluated
* @return minimum maybe context-dependent element value
* @throws ChronoException if there is no suitable element rule for evaluating the minimum value
* or if the associated element value is not defined over the complete range of this instance
* @see ChronoElement#getDefaultMinimum()
* @see #getMaximum(ChronoElement)
*/
V getMinimum(ChronoElement element);
/**
* Yields the maximum value of given chronological element in the
* current context of this object.
*
* Maximum values are different from minimum case often dependent
* on the context. An example is the element SECOND_OF_MINUTE whose
* maximum is normally {@code 59} but can differ in UTC-context with
* leap seconds. Another more common example is the maximum of the
* element DAY_OF_MONTH (28-31) which is dependent on the month and year
* of this context (leap years!).
*
* Note: In timezone-related timestamps possible offset jumps
* inducing gaps on the local timeline will be conserved. That means
* that minimum and maximum do not guarantee a continuum of valid
* intermediate values.
*
* @param generic type of element value
* @param element element whose maximum value is to be evaluated
* @return maximum maybe context-dependent element value
* @throws ChronoException if there is no suitable element rule for evaluating the maximum value
* or if the associated element value is not defined over the complete range of this instance
* @see ChronoElement#getDefaultMaximum()
* @see #getMinimum(ChronoElement)
*/
/*[deutsch]
* Ermittelt das Maximum des mit dem angegebenen chronologischen Element
* assoziierten Elementwerts.
*
* Maximalwerte sind anders als Minima häufig vom Kontext
* abhängig. Ein Beispiel sind Sekundenelemente und die Frage der
* Existenz von Schaltsekunden im gegebenen Kontext (evtl. als Maximum
* die Werte {@code 58} oder {@code 60} statt {@code 59} möglich).
* Ein anderes Beispiel ist der Maximalwert eines Tags (28-31), der
* abhängig vom Monats- und Jahreskontext (Schaltjahre!) ist.
*
* Zu beachten: In zeitzonen-bezogenen Zeitstempeln bleiben eventuelle
* Zeitzonensprünge erhalten. Das bedeutet, daß Minimum und
* Maximum nicht berücksichtigen, ob sie in eine Lücke fallen
* oder zwischen ihnen ein Offset-Sprung existiert.
*
* @param generic type of element value
* @param element element whose maximum value is to be evaluated
* @return maximum maybe context-dependent element value
* @throws ChronoException if there is no suitable element rule for evaluating the maximum value
* or if the associated element value is not defined over the complete range of this instance
* @see ChronoElement#getDefaultMaximum()
* @see #getMinimum(ChronoElement)
*/
V getMaximum(ChronoElement element);
/**
* Queries if this object contains a timezone for display purposes.
*
* @return {@code true} if a timezone is available and can be achieved
* with {@link #getTimezone()} else {@code false}
*/
/*[deutsch]
* Ermittelt, ob eine Zeitzone für Anzeigezwecke vorhanden ist.
*
* @return {@code true} if a timezone is available and can be achieved
* with {@link #getTimezone()} else {@code false}
*/
boolean hasTimezone();
/**
* Returns the associated timezone ID for display purposes
* if available.
*
* Note: Although global types like {@code Moment} indeed have a
* timezone reference (namely UTC+00:00), such types will not support
* formatted output without explicitly giving a timezone for display
* purposes. Therefore calling this method on global types will throw
* an exception. This method is not just about any timezone reference
* but a timezone designed for display purposes.
*
* @return timezone identifier if available
* @throws ChronoException if the timezone is not available
* @see #hasTimezone()
*/
/*[deutsch]
* Liefert die assoziierte Zeitzonen-ID für Anzeigezwecke,
* wenn vorhanden.
*
* Hinweis: Obwohl globale Typen wie {@code Moment} sehr wohl einen
* Zeitzonenbezug haben (nämlich UTC+00:00), werden sie keine
* formatierte Ausgabe ohne eine explizite Zeitzonenangabe für
* Anzeigezwecke unterstützen. Deshalb wird der Aufruf dieser
* Methode bei globalen Typen eine Ausnahme werfen. Diese Methode ist
* nicht einfach auf irgendeine Zeitzone bezogen, die vorhanden sein
* mag, sondern explizit nur für Zeitzonen im Kontext von formatierten
* Ausgaben gedacht.
*
* @return timezone identifier if available
* @throws ChronoException if the timezone is not available
* @see #hasTimezone()
*/
TZID getTimezone();
}