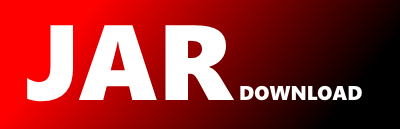
net.time4j.engine.ChronoElement Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (ChronoElement.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.engine;
import java.util.Comparator;
/**
* Represents a chronological element which refers to a partial value of
* the whole temporal value and mainly serves for formatting purposes (as
* a carrier of a chronological information).
*
* Each chronological system knows a set of elements whose values
* compose the total temporal value, usually the associated time coordinates
* on a time axis. Each element can be associated with a value of type V.
* Normally this value is an integer or an enum. Examples are the hour on
* a clock or the month of a calendar date.
*
* The associated values are often defined as continuum without any
* gaps as integer within a given value range. However, there is no
* guarantee for such a continuum, for example daylight-saving-jumps or
* hebrew leap months.
*
* @param generic type of element values, usually extending the interface
* {@code java.lang.Comparable} (or it can be converted to any other
* sortable form)
* @author Meno Hochschild
* @see ChronoEntity#get(ChronoElement)
* @doctags.spec All implementations must be immutable and serializable.
*/
/*[deutsch]
* Repräsentiert ein chronologisches Element, das einen Teil eines
* Zeitwerts referenziert und als Träger einer chronologischen Information
* in erster Linie Formatierzwecken dient.
*
* Jedes chronologische System kennt einen Satz von Elementen, deren Werte
* zusammen den Gesamtzeitwert festlegen, in der Regel die zugehörige Zeit
* als Punkt auf einem Zeitstrahl. Jedes Element kann mit einem Wert vom Typ V
* assoziiert werden. Normalerweise handelt es sich um einen Integer-Wert oder
* einen Enum-Wert. Beispiele sind die Stunde einer Uhrzeit oder der Monat
* eines Datums.
*
* Die zugehörigen Werte sind, wenn vorhanden, oft als Kontinuum
* ohne Lücken als Integer im angegebenen Wertebereich definiert.
* Garantiert ist ein Kontinuum aber nicht, siehe zum Beispiel
* Sommerzeit-Umstellungen oder hebräische Schaltmonate.
*
* @param generic type of element values, usually extending the interface
* {@code java.lang.Comparable} (or it can be converted to any other
* sortable form)
* @author Meno Hochschild
* @see ChronoEntity#get(ChronoElement)
* @doctags.spec All implementations must be immutable and serializable.
*/
public interface ChronoElement
extends Comparator {
//~ Methoden ----------------------------------------------------------
/**
* Returns the name which is unique within the context of a given
* chronology.
*
* The name can also serve as resource key together with the name of
* a chronology for a localized description.
*
* @return name of element, unique within a chronology
*/
/*[deutsch]
* Liefert den innerhalb einer Chronologie eindeutigen Namen.
*
* Zusammen mit dem Namen der zugehörigen Chronologie kann
* der Name des chronologischen Elements auch als Ressourcenschlüssel
* für eine lokalisierte Beschreibung dienen.
*
* @return name of element, unique within a chronology
*/
String name();
/**
* Yields the reified value type.
*
* @return type of associated values
*/
/*[deutsch]
* Liefert die Wertklasse.
*
* @return type of associated values
*/
Class getType();
/**
* Defines the default format symbol which is used in format patterns
* to refer to this element.
*
* In most cases the symbol should closely match the symbol-mapping
* as defined by the CLDR-standard of unicode-organization. Is the
* element not designed for formatting using patterns then this method
* just yields the ASCII-char "\u0000" (Codepoint 0).
*
* @return format symbol as char
* @see FormattableElement
*/
/*[deutsch]
* Definiert das Standard-Formatsymbol, mit dem diese Instanz in
* Formatmustern referenziert wird.
*
* So weit wie möglich handelt es sich um ein Symbol nach der
* CLDR-Norm des Unicode-Konsortiums. Ist das Element nicht für
* Formatierungen per Formatmuster vorgesehen, liefert diese Methode das
* ASCII-Zeichen "\u0000" (Codepoint 0).
*
* @return format symbol as char
* @see FormattableElement
*/
char getSymbol();
/**
* Applies an element-orientated sorting of any chronological
* entities.
*
* The value type V is usually a subtype of the interface
* {@code Comparable} so that this method will usually be based on
* the expression {@code o1.get(this).compareTo(o2.get(this))}. In
* other words, this method compares the element values of given
* chronological entities. It is even permitted to compare entities
* of different chronological types as long as the entities both
* support this element.
*
* It should be noted however that a element value comparison does
* often not induce any temporal (complete) order. Counter examples
* are the year of gregorian BC-era (reversed temporal order before
* Jesu birth) or the clock display of hours (12 is indeed the begin
* at midnight).
*
* @param o1 the first object to be compared
* @param o2 the second object to be compared
* @return a negative integer, zero, or a positive integer as the first
* argument is less than, equal to, or greater than the second
* @throws ChronoException if this element is not registered in any entity
* and/or if no element rule exists to extract the element value
*/
/*[deutsch]
* Führt eine elementorientierte Sortierung von beliebigen
* chronologischen Objekten bezüglich dieses Elements ein .
*
* Der Werttyp V ist typischerweise eine Implementierung des Interface
* {@code Comparable}, so daß diese Methode oft auf dem Ausdruck
* {@code o1.get(this).compareTo(o2.get(this))} beruhen wird. Mit anderen
* Worten, im Normalfall werden in den angegebenen chronologischen Objekten
* die Werte bezüglich dieses Elements verglichen. Es dürfen
* anders als im {@code Comparable}-Interface möglich hierbei auch
* Objekte verschiedener chronologischer Typen miteinander verglichen
* werden solange die zu vergleichenden Objekte dieses Element
* unterstützen.
*
* Es ist zu betonen, daß ein Elementwertvergleich im allgemeinen
* keine (vollständige) zeitliche Sortierung indiziert. Gegenbeispiele
* sind das Jahr innerhalb der gregorianischen BC-Ära (umgekehrte
* zeitliche Reihenfolge vor Christi Geburt) oder die Ziffernblattanzeige
* von Stunden (die Zahl 12 ist eigentlich der Beginn zu Mitternacht).
*
* @param o1 the first object to be compared
* @param o2 the second object to be compared
* @return a negative integer, zero, or a positive integer as the first
* argument is less than, equal to, or greater than the second
* @throws ChronoException if this element is not registered in any entity
* and/or if no element rule exists to extract the element value
*/
@Override
int compare(
ChronoDisplay o1,
ChronoDisplay o2
);
/**
* Returns the default minimum of this element which is not dependent
* on the chronological context.
*
* Note: This minimum does not necessarily define a minimum for all
* possible circumstances but only the default minimum under normal
* conditions such that the default minimum always exists and can be
* used as prototypical value. An example is the start of day which
* is usually midnight in ISO-8601 and can only deviate in specialized
* timezone context.
*
* @return default minimum value of element
* @see #getDefaultMaximum()
*/
/*[deutsch]
* Gibt das übliche chronologieunabhängige Minimum des
* assoziierten Elementwerts zurück.
*
* Hinweis: Dieses Minimum definiert keineswegs eine untere Schranke
* für alle gültigen Elementwerte, sondern nur das Standardminimum
* unter chronologischen Normalbedingungen, unter denen es immer existiert
* und daher als prototypischer Wert verwendet werden kann. Ein Beispiel
* ist der Start des Tages in ISO-8601, welcher nur unter bestimmten
* Zeitzonenkonfigurationen von Mitternacht abweichen kann.
*
* @return default minimum value of element
* @see #getDefaultMaximum()
*/
V getDefaultMinimum();
/**
* Returns the default maximum of this element which is not dependent
* on the chronological context.
*
* Note: This maximum does not necessarily define a maximum for all
* possible circumstances but only the default maximum under normal
* conditions. An example is the second of minute whose default maximum
* is {@code 59} while the largest maximum can be {@code 60} in context
* of UTC-leapseconds.
*
* @return default maximum value of element
* @see #getDefaultMinimum()
*/
/*[deutsch]
* Gibt das übliche chronologieunabhängige Maximum des
* assoziierten Elementwerts zurück.
*
* Hinweis: Dieses Maximum definiert keineswegs eine obere Schranke
* für alle gültigen Elementwerte, sondern nur das Standardmaximum
* unter chronologischen Normalbedingungen . Ein Beispiel ist das
* Element SECOND_OF_MINUTE, dessen Standardmaximum {@code 59} ist,
* während das größte Maximum bedingt durch
* UTC-Schaltsekunden {@code 60} sein kann.
*
* @return default maximum value of element
* @see #getDefaultMinimum()
*/
V getDefaultMaximum();
/**
* Queries if this element represents a calendar date element.
*
* @return boolean
* @see #isTimeElement()
*/
/*[deutsch]
* Ist dieses Element eine Teilkomponente, die ein Datumselement
* darstellt?
*
* @return boolean
* @see #isTimeElement()
*/
boolean isDateElement();
/**
* Queries if this element represents a wall time element.
*
* @return boolean
* @see #isDateElement()
*/
/*[deutsch]
* Ist dieses Element eine Teilkomponente, die ein Uhrzeitelement
* darstellt?
*
* @return boolean
* @see #isDateElement()
*/
boolean isTimeElement();
/**
* Queries if setting of element values is performed in a lenient
* way.
*
* @return {@code true} if lenient else {@code false} (standard)
* @see ElementRule#withValue(Object, Object, boolean)
* ElementRule.withValue(T, V, boolean)
*/
/*[deutsch]
* Soll das Setzen von Werten fehlertolerant ausgeführt werden?
*
* @return {@code true} if lenient else {@code false} (standard)
* @see ElementRule#withValue(Object, Object, boolean)
* ElementRule.withValue(T, V, boolean)
*/
boolean isLenient();
}