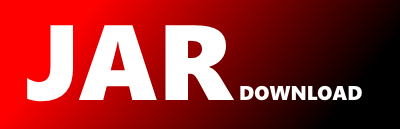
net.time4j.engine.ChronoFunction Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2015 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (ChronoFunction.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.engine;
import java.util.function.Function;
/**
* Represents any temporal query using the strategy pattern approach.
*
* @param generic type of source
* @param generic type of result
* @author Meno Hochschild
* @see ChronoEntity#get(ChronoFunction)
*/
/*[deutsch]
* Repräsentiert eine beliebige zeitliche Abfrage entsprechend
* dem Strategie-Entwurfsmuster.
*
* @param generic type of source
* @param generic type of result
* @author Meno Hochschild
* @see ChronoEntity#get(ChronoFunction)
*/
public interface ChronoFunction
extends Function {
//~ Methoden ----------------------------------------------------------
/**
* Reads and evaluates given time value context to a specific result
* of type R.
*
* Will be called by {@link ChronoEntity#get(ChronoFunction)}.
* Concrete implementations must document if they rather yield
* {@code null} or throw an exception in case of undefined results.
*
* @param context time context to be evaluated
* @return result of query or {@code null} if undefined
* @throws ChronoException if this query is not executable
*/
/*[deutsch]
* Liest und interpretiert den angegebenen Zeitwertkontext.
*
* Wird von {@link ChronoEntity#get(ChronoFunction)} aufgerufen.
* Konkrete Implementierungen müssen dokumentieren, ob
* sie im Fall von undefinierten Ergebnissen eher {@code null}
* zurückgeben oder stattdessen eine Ausnahme werfen.
*
* @param context time context to be evaluated
* @return result of query or {@code null} if undefined
* @throws ChronoException if this query is not executable
*/
R apply(T context);
}