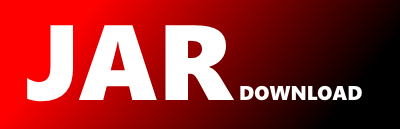
net.time4j.tz.ZoneNameProvider Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2016 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (ZoneNameProvider.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.tz;
import java.util.Locale;
import java.util.Set;
/**
* SPI interface which encapsulates the timezone name repository.
*
* Implementations are usually stateless and should normally not
* try to manage a cache. Instead Time4J uses its own cache. The
* fact that this interface is used per {@code java.util.ServiceLoader}
* requires a concrete implementation to offer a public no-arg
* constructor.
*
* @author Meno Hochschild
* @since 3.20/4.16
* @see java.util.ServiceLoader
*/
/*[deutsch]
* SPI-Interface, das eine Datenbank für Zeitzonennamen kapselt.
*
* Implementierungen sind in der Regel zustandslos und halten keinen
* Cache. Letzterer sollte normalerweise der Klasse {@code Timezone}
* vorbehalten sein. Weil dieses Interface mittels eines
* {@code java.util.ServiceLoader} genutzt wird, muß eine
* konkrete Implementierung einen öffentlichen Konstruktor ohne
* Argumente definieren.
*
* @author Meno Hochschild
* @since 3.20/4.16
* @see java.util.ServiceLoader
*/
public interface ZoneNameProvider {
//~ Methoden ----------------------------------------------------------
/**
* Gets a {@code Set} of preferred timezone IDs for given
* ISO-3166-country code.
*
* This information is necessary to enable parsing of ambivalent
* timezone names.
*
* @param locale ISO-3166-alpha-2-country to be evaluated
* @param smart if {@code true} then try to select zone ids such
* that there is only one preferred id per zone name
* @return unmodifiable set of preferred timezone ids
*/
/*[deutsch]
* Liefert die für einen gegebenen ISO-3166-Ländercode
* bevorzugten Zeitzonenkennungen.
*
* Diese Information ist für die Interpretation von mehrdeutigen Zeitzonennamen
* notwendig.
*
* @param locale ISO-3166-alpha-2-country to be evaluated
* @param smart if {@code true} then try to select zone ids such
* that there is only one preferred id per zone name
* @return unmodifiable set of preferred timezone ids
*/
Set getPreferredIDs(
Locale locale,
boolean smart
);
/**
* Returns the name of this timezone suitable for presentation to
* users in given style and locale.
*
* The first argument never contains the provider name as prefix. It is
* instead the part after the "~"-char (if not absent).
*
* @param zoneID timezone id (i.e. "Europe/London")
* @param style name style
* @param locale language setting
* @return localized timezone name for display purposes
* or empty if not supported
* @see java.util.TimeZone#getDisplayName(boolean,int,Locale)
* java.util.TimeZone.getDisplayName(boolean,int,Locale)
*/
/*[deutsch]
* Liefert den anzuzeigenden Zeitzonennamen.
*
* Das erste Argument enthält nie den Provider-Namen als
* Präfix. Stattdessen ist es der Teil nach dem Zeichen
* "~" (falls vorhanden).
*
* @param zoneID timezone id (i.e. "Europe/London")
* @param style name style
* @param locale language setting
* @return localized timezone name for display purposes
* or empty if not supported
* @see java.util.TimeZone#getDisplayName(boolean,int,Locale)
* java.util.TimeZone.getDisplayName(boolean,int,Locale)
*/
String getDisplayName(
String zoneID,
NameStyle style,
Locale locale
);
}