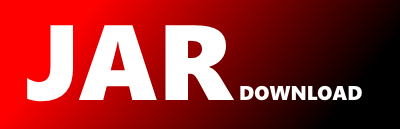
net.time4j.engine.CalendarDays Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2015 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (CalendarDays.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.engine;
import java.io.Serializable;
/**
* Represents a count of calendar days.
*
* @author Meno Hochschild
* @since 3.4/4.3
* @doctags.concurrency {immutable}
*/
/*[deutsch]
* Repräsentiert eine Anzahl von Kalendertagen.
*
* @author Meno Hochschild
* @since 3.4/4.3
* @doctags.concurrency {immutable}
*/
public final class CalendarDays
implements Comparable, Serializable {
//~ Statische Felder/Initialisierungen --------------------------------
/**
* Represents zero calendar days.
*/
/*[deutsch]
* Repräsentiert null Kalendertage.
*/
public static final CalendarDays ZERO = new CalendarDays(0);
/**
* Represents exactly one calendar day.
*/
/*[deutsch]
* Repräsentiert genau einen Kalendertag.
*/
public static final CalendarDays ONE = new CalendarDays(1);
//~ Instanzvariablen --------------------------------------------------
/**
* @serial count of days
*/
/*[deutsch]
* @serial Anzahl der Kalendertage
*/
private final long days;
//~ Konstruktoren -----------------------------------------------------
private CalendarDays(long days) {
super();
this.days = days;
}
//~ Methoden ----------------------------------------------------------
/**
* Wraps given count of calendar days.
*
* @param days count of calendar days
* @return new or cached instance of {@code CalendarDays}
* @since 3.4/4.3
*/
/*[deutsch]
* Kapselt die angegebenen Kalendertage als Objekt.
*
* @param days count of calendar days
* @return new or cached instance of {@code CalendarDays}
* @since 3.4/4.3
*/
public static CalendarDays of(long days) {
return ((days == 0) ? CalendarDays.ZERO : ((days == 1) ? CalendarDays.ONE : new CalendarDays(days)));
}
/**
* Yields the calendar days as primitive.
*
* @return count of calendar days, maybe zero or negative
* @since 3.4/4.3
*/
/*[deutsch]
* Liefert die Kalendertage als Java-Primitive.
*
* @return count of calendar days, maybe zero or negative
* @since 3.4/4.3
*/
public long getAmount() {
return this.days;
}
/**
* Is the count of calendar days equal to zero?
*
* @return boolean
* @since 3.4/4.3
*/
/*[deutsch]
* Ist die Anzahl der Kalendertage {@code 0}?
*
* @return boolean
* @since 3.4/4.3
*/
public boolean isZero() {
return (this.days == 0);
}
/**
* Is the count of calendar days smaller than zero?
*
* @return boolean
* @since 3.4/4.3
*/
/*[deutsch]
* Ist die Anzahl der Kalendertage negativ?
*
* @return boolean
* @since 3.4/4.3
*/
public boolean isNegative() {
return (this.days < 0);
}
/**
* Calculates the delta of calendar days between given calendar dates.
*
* @param start first calendar date (inclusive)
* @param end second calendar date (exclusive)
* @return count of calendar days between start and end
* @since 3.8/4.5
*/
/*[deutsch]
* Berechnet die Tagesdifferenz zwischen den angegebenen Datumsobjekten.
*
* @param start first calendar date (inclusive)
* @param end second calendar date (exclusive)
* @return count of calendar days between start and end
* @since 3.8/4.5
*/
public static CalendarDays between(
CalendarDate start,
CalendarDate end
){
long t1 = start.getDaysSinceEpochUTC();
long t2 = end.getDaysSinceEpochUTC();
return CalendarDays.of(Math.subtractExact(t2, t1));
}
/**
* Yields the absolute value of the represented calendar days.
*
* @return non-negative count of calendar days
* @since 3.4/4.3
*/
/*[deutsch]
* Liefert den absoluten Betrag der repräsentierten Kalendertage.
*
* @return non-negative count of calendar days
* @since 3.4/4.3
*/
public CalendarDays abs() {
return ((this.days < 0) ? CalendarDays.of(Math.negateExact(this.days)) : this);
}
/**
* Yields the sum of the represented calendar days of this instance and given argument.
*
* @param other calendar days to be added
* @return sum of calendar days
* @since 3.4/4.3
*/
/*[deutsch]
* Liefert die Summe der Kalendertage dieser Instanz und des angegebenen Arguments.
*
* @param other calendar days to be added
* @return sum of calendar days
* @since 3.4/4.3
*/
public CalendarDays plus(CalendarDays other) {
return CalendarDays.of(Math.addExact(this.days, other.days));
}
/**
* Yields the delta of the represented calendar days of this instance and given argument.
*
* @param other calendar days to be subtracted
* @return delta of calendar days
* @since 3.4/4.3
*/
/*[deutsch]
* Liefert die Differenz der Kalendertage dieser Instanz und des angegebenen Arguments.
*
* @param other calendar days to be subtracted
* @return delta of calendar days
* @since 3.4/4.3
*/
public CalendarDays minus(CalendarDays other) {
return CalendarDays.of(Math.subtractExact(this.days, other.days));
}
@Override
public int compareTo(CalendarDays other) {
return ((this.days < other.days) ? -1 : ((this.days > other.days) ? 1 : 0));
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
} else if (obj instanceof CalendarDays) {
return (this.days == ((CalendarDays) obj).days);
} else {
return false;
}
}
@Override
public int hashCode() {
return (int) (this.days ^ (this.days >>> 32));
}
/**
* Returns an ISO-8601-like duration representation in format "[-]P{n}D".
*
* @return String
*/
/*[deutsch]
* Liefert eine ISO-8601-ähnliche Darstellung im Format "[-]P{n}D".
*
* @return String
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
if (this.days < 0) {
sb.append('-');
}
sb.append('P');
sb.append(Math.abs(this.days));
sb.append('D');
return sb.toString();
}
}