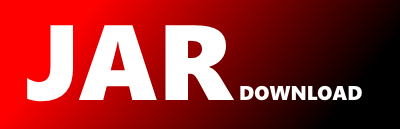
net.time4j.engine.FormattableElement Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2014 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (FormattableElement.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.engine;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* This {@code Annotation} can be used to document all chronological
* elements which allow formatted representations.
*
* Usage note: Usually only element constants with the modifiers
* static und final are target of this {@code Annotation}.
* The target type {@code ElementType.METHOD} is only permitted if
* elements are generated in a {@code ChronoExtension}.
*
* @author Meno Hochschild
* @see ChronoElement
* @see ChronoExtension
*/
/*[deutsch]
* Mit dieser {@code Annotation} können alle chronologischen Elemente
* dokumentiert werden, die formatierte Darstellungen erlauben.
*
* Verwendungshinweis: Ziel der {@code Annotation} sind normalerweise
* nur Elementkonstanten mit den Modifiern static und final.
* Der Zieltyp {@code ElementType.METHOD} ist lediglich dann erlaubt, wenn
* Elemente in einer {@code ChronoExtension} generiert werden.
*
* @author Meno Hochschild
* @see ChronoElement
* @see ChronoExtension
*/
@Documented
@Target({ElementType.FIELD, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
public @interface FormattableElement {
//~ Methoden --------------------------------------------------------------
/**
* Returns the associated format pattern symbol in the standard
* format context.
*
* Format pattern symbols should be unique among all registered
* elements of a given chronology. In standard elements they correspond
* to the format symbols defined by unicode organization in CLDR. The
* symbol is case-sensitive.
*
* The default value of an empty string means that the associated
* element is not primarily designed for formatting via a pattern
* expression.
*
* @return char
* @see net.time4j.format.OutputContext#FORMAT
*/
/*[deutsch]
* Liefert das zugehörige Formatmusterzeichen im normalen
* Formatkontext.
*
* Formatmusterzeichen sollten unter allen registrierten Elementen
* einer gegebenen Chronologie eindeutig sein. In Standardelementen
* entsprechen sie den in der vom Unicode-Konsortium definierten
* CLDR-Syntax festgelegten Formatsymbolen. Es wird zwischen Groß-
* und Kleinschreibung unterschieden.
*
* Der Standardwert einer leeren Zeichenkette bedeutet hier, daß
* kein Zeichen in einem Formatmuster vorgesehen ist.
*
* @return char
* @see net.time4j.format.OutputContext#FORMAT
*/
String format() default "";
/**
* Returns the associated format pattern symbol in the stand-alone
* context.
*
* Almost equivalent to {@code format()} with the difference that
* the element shall be formatted in a stand-alone context (for example
* nominative form "x January" instead of the ordinal form
* "x-th January"). However, the stand-alone context is not
* relevant for English - as "January" is still the same word,
* only relevant for some languages which make an explicit grammar
* difference.
*
* The default value of an empty string just means that the
* stand-alone context should use the same value as in the format
* context.
*
* @return char
* @see net.time4j.format.OutputContext#STANDALONE
*/
/*[deutsch]
* Liefert das zugehörige Formatmusterzeichen für die
* Verwendung in alleinstehendem Kontext.
*
* Entspricht weitgehend {@code format()} mit dem Unterschied,
* daß hier das Element in einem alleinstehenden Kontext
* formatiert werden soll (zum Beispiel nominativ "x Januar"
* statt der Ordinalform "x-ter Januar").
*
* Der Standardwert einer leeren Zeichenkette bedeutet nur, daß
* der gleiche Wert wie im normalen Formatkontext gelten soll.
*
* @return char
* @see net.time4j.format.OutputContext#STANDALONE
*/
String standalone() default "";
}