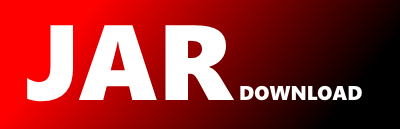
net.time4j.format.FormatPatternProvider Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2015 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (FormatPatternProvider.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.format;
import java.util.Locale;
/**
* This SPI-interface enables the access to localized gregorian
* date-, time- or interval patterns according to the CLDR-specifiation and is instantiated via a
* {@code ServiceLoader}-mechanism.
*
* If there is no external {@code FormatPatternProvider} then Time4J will
* just delegate to the JDK.
*
* Specification:
* Implementations must have a public no-arg constructor.
*
* @author Meno Hochschild
* @since 3.10/4.7
* @see java.util.ServiceLoader
* @see java.text.SimpleDateFormat#toPattern()
*/
/*[deutsch]
* Dieses SPI-Interface ermöglicht den Zugriff auf gregorianische
* {@code Locale}-abhängige Formatmuster für Datum, Uhrzeit oder Intervalle
* entsprechend der CLDR-Spezifikation und wird über einen {@code ServiceLoader}-Mechanismus
* instanziert.
*
* Wird kein externer {@code FormatPatternProvider} gefunden, wird intern
* eine Instanz erzeugt, die an das JDK delegiert.
*
* Specification:
* Implementations must have a public no-arg constructor.
*
* @author Meno Hochschild
* @since 3.10/4.7
* @see java.util.ServiceLoader
* @see java.text.SimpleDateFormat#toPattern()
*/
public interface FormatPatternProvider {
//~ Methoden ----------------------------------------------------------
/**
* Returns the localized date pattern suitable for formatting of objects
* of type {@code PlainDate}.
*
* @param mode display mode
* @param locale language and country setting
* @return localized date pattern
* @see net.time4j.PlainDate
*/
/*[deutsch]
* Liefert das lokalisierte Datumsmuster geeignet für
* die Formatierung von Instanzen des Typs{@code PlainDate}.
*
* @param mode display mode
* @param locale language and country setting
* @return localized date pattern
* @see net.time4j.PlainDate
*/
String getDatePattern(
DisplayMode mode,
Locale locale
);
/**
* Returns the localized time pattern suitable for formatting of objects
* of type {@code PlainTime}.
*
* @param mode display mode
* @param locale language and country setting
* @return localized time pattern
* @see net.time4j.PlainTime
*/
/*[deutsch]
* Liefert das lokalisierte Uhrzeitmuster geeignet für die
* Formatierung von Instanzen des Typs {@code PlainTime}.
*
* @param mode display mode
* @param locale language and country setting
* @return localized time pattern
* @see net.time4j.PlainTime
*/
String getTimePattern(
DisplayMode mode,
Locale locale
);
/**
* Returns the localized date-time pattern suitable for formatting of objects
* of type {@code Moment} or {@code PlainTimestamp}.
*
* Expressions of the form "{0}" will be interpreted as the time component
* and expressions of the form "{1}" will be interpreted as the date component.
* All other chars of the pattern will be treated as literals.
*
* @param dateMode display mode of date part
* @param timeMode display mode of time part
* @param locale language and country setting
* @return localized date-time pattern
* @see net.time4j.Moment
* @see net.time4j.PlainTimestamp
*/
/*[deutsch]
* Liefert das lokalisierte Datums- und Uhrzeitmuster geeignet für die Formatierung
* von Instanzen des Typs {@code Moment} oder {@code PlainTimestamp}.
*
* Die Ausdrücke "{0}" und "{1}" werden als Formathalter für die
* Uhrzeit- und Datumskomponenten interpretiert. Alle anderen Zeichen des Musters werden wie
* Literale behandelt.
*
* @param dateMode display mode of date part
* @param timeMode display mode of time part
* @param locale language and country setting
* @return localized date-time pattern
* @see net.time4j.Moment
* @see net.time4j.PlainTimestamp
*/
String getDateTimePattern(
DisplayMode dateMode,
DisplayMode timeMode,
Locale locale
);
/**
* Returns the localized interval pattern.
*
* Expressions of the form "{0}" will be interpreted as the start boundary format
* and expressions of the form "{1}" will be interpreted as the end boundary format.
* All other chars of the pattern will be treated as literals.
*
* @param locale language and country setting
* @return localized interval pattern
*/
/*[deutsch]
* Liefert das lokalisierte Intervallmuster.
*
* Die Ausdrücke "{0}" und "{1}" werden als Formathalter für die
* Start- und End-Intervallgrenzen interpretiert. Alle anderen Zeichen des Musters werden wie
* Literale behandelt.
*
* @param locale language and country setting
* @return localized interval pattern
*/
String getIntervalPattern(Locale locale);
}