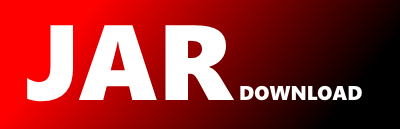
net.time4j.engine.ChronoMerger Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2017 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (ChronoMerger.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.engine;
import net.time4j.base.TimeSource;
import java.time.temporal.TemporalAccessor;
import java.util.Locale;
/**
* Merges any set of chronological informations to a new chronological
* entity.
*
* This interface abstracts the knowledge of the chronology how to
* construct a new entity based on any set of chronological informations.
* It is mainly used by a parser in order to interprete textual representations
* of chronological entities.
*
* Using this low-level-interface is usually reserved for Time4J and
* serves for internal format support. However, for constructing new
* calendar systems implementing this interface is an essential part
* for parsing support. Implementation note: All classes of this type
* must be immutable.
*
* @param generic type of time context
* (compatible to {@link ChronoEntity})
* @author Meno Hochschild
*/
/*[deutsch]
* Erzeugt aus chronologischen Informationen eine neue
* {@code ChronoEntity}.
*
* Dieses Interface abstrahiert das Wissen der jeweiligen Zeitwertklasse,
* wie aus beliebigen chronologischen Informationen eine neue Zeitwertinstanz
* zu konstruieren ist und wird zum Beispiel beim Parsen von textuellen
* Darstellungen zu Zeitwertobjekten benötigt.
*
* Die Benutzung dieses Low-Level-Interface bleibt in der Regel Time4J
* vorbehalten und dient vorwiegend der internen Formatunterstützung.
* Zur Konstruktion von neuen Kalendersystemen ist die Implementierung
* dieses Interface ein wichtiger Bestandteil. Implementierungshinweis:
* Alle Klassen dieses Typs müssen immutable, also
* unveränderlich sein.
*
* @param generic type of time context
* (compatible to {@link ChronoEntity})
* @author Meno Hochschild
*/
public interface ChronoMerger {
//~ Methoden ----------------------------------------------------------
/**
* Creates a new entity which reflects current time.
*
* In a date-only chronology this method will create the current date
* using the necessary timezone contained in given attributes.
*
* @param clock source for current time
* @param attributes configuration attributes which might contain
* the timezone to translate current time to
* local time
* @return new time context or {@code null} if given data are insufficient
*/
/*[deutsch]
* Konstruiert eine neue Entität, die der aktuellen Zeit
* entspricht.
*
* In einer rein datumsbezogenen kalendarischen Chronologie wird hier
* das aktuelle Tagesdatum erzeugt, indem zusätzlich über die
* Attribute die notwendige Zeitzone ermittelt wird.
*
* @param clock source for current time
* @param attributes configuration attributes which might contain
* the timezone to translate current time to
* local time
* @return new time context or {@code null} if given data are insufficient
*/
T createFrom(
TimeSource> clock,
AttributeQuery attributes
);
/**
* Creates a new entity of type T based on given chronological
* data.
*
* Typically the method will query the given {@code entity} with
* different priorities for elements which can compose a new chronological
* entity (per group). For example a calendar date can be composed either
* by epoch days or the group (year)-(month)-(day-of-month) or the group
* (year)-(day-of-year) etc.
*
* A text parser will call this method after having resolved a text
* into single chronological elements and values. Implementations should
* always validate the parsed values. In case of error, they are free to
* either throw an {@code IllegalArgumentException} or to generate
* and to save an error message by mean of the expression
* {@code entity.with(ValidationElement.ERROR_MESSAGE, message}.
*
* @deprecated use {@link #createFrom(ChronoEntity, AttributeQuery, boolean, boolean)} instead
* @param entity any chronological entity like parsed
* elements with their values
* @param attributes configuration attributes given by parser
* @param preparsing preparsing phase active?
* @return new time context or {@code null} if given data are insufficient
* @throws IllegalArgumentException in any case of inconsistent data
* @see ValidationElement#ERROR_MESSAGE
*/
/*[deutsch]
* Konstruiert eine neue Entität basierend auf den angegebenen
* chronologischen Daten.
*
* Typischerweise wird mit verschiedenen Prioritäten das Argument
* {@code entity} nach Elementen abgefragt, die gruppenweise einen
* Zeitwert konstruieren. Zum Beispiel kann ein Datum entweder über
* die Epochentage, die Gruppe Jahr-Monat-Tag oder die Gruppe Jahr und Tag
* des Jahres konstruiert werden.
*
* Ein Textinterpretierer ruft diese Methode auf, nachdem ein Text
* elementweise in chronologische Elemente und Werte aufgelöst
* wurde. Implementierungen sollten immer die interpretierten Werte
* validieren. Im Fehlerfall sind Implementierungen frei, entweder eine
* {@code IllegalArgumentException} zu werfen oder mittels des Ausdrucks
* {@code entity.with(ValidationElement.ERROR_MESSAGE, message} eine
* Fehlermeldung nur zu generieren und zu speichern.
*
* @deprecated use {@link #createFrom(ChronoEntity, AttributeQuery, boolean, boolean)} instead
* @param entity any chronological entity like parsed
* elements with their values
* @param attributes configuration attributes given by parser
* @param preparsing preparsing phase active?
* @return new time context or {@code null} if given data are insufficient
* @throws IllegalArgumentException in any case of inconsistent data
* @see ValidationElement#ERROR_MESSAGE
*/
@Deprecated
T createFrom(
ChronoEntity> entity,
AttributeQuery attributes,
boolean preparsing
);
/**
* Creates a new entity of type T based on given chronological data.
*
* Typically the method will query the given {@code entity} with
* different priorities for elements which can compose a new chronological
* entity (per group). For example a calendar date can be composed either
* by epoch days or the group (year)-(month)-(day-of-month) or the group
* (year)-(day-of-year) etc.
*
* A text parser will call this method after having resolved a text
* into single chronological elements and values. Implementations should
* always validate the parsed values. In case of error, they are free to
* either throw an {@code IllegalArgumentException} or to generate
* and to save an error message by mean of the expression
* {@code entity.with(ValidationElement.ERROR_MESSAGE, message}.
*
* @param entity any chronological entity like parsed
* elements with their values
* @param attributes configuration attributes given by parser
* @param lenient controls the leniency how to interprete invalid values
* @param preparsing preparsing phase active?
* @return new time context or {@code null} if given data are insufficient
* @throws IllegalArgumentException in any case of inconsistent data
* @see ValidationElement#ERROR_MESSAGE
* @since 3.15/4.12
*/
/*[deutsch]
* Konstruiert eine neue Entität basierend auf den angegebenen
* chronologischen Daten.
*
* Typischerweise wird mit verschiedenen Prioritäten das Argument
* {@code entity} nach Elementen abgefragt, die gruppenweise einen
* Zeitwert konstruieren. Zum Beispiel kann ein Datum entweder über
* die Epochentage, die Gruppe Jahr-Monat-Tag oder die Gruppe Jahr und Tag
* des Jahres konstruiert werden.
*
* Ein Textinterpretierer ruft diese Methode auf, nachdem ein Text
* elementweise in chronologische Elemente und Werte aufgelöst
* wurde. Implementierungen sollten immer die interpretierten Werte
* validieren. Im Fehlerfall sind Implementierungen frei, entweder eine
* {@code IllegalArgumentException} zu werfen oder mittels des Ausdrucks
* {@code entity.with(ValidationElement.ERROR_MESSAGE, message} eine
* Fehlermeldung nur zu generieren und zu speichern.
*
* @param entity any chronological entity like parsed
* elements with their values
* @param attributes configuration attributes given by parser
* @param lenient controls the leniency how to interprete invalid values
* @param preparsing preparsing phase active?
* @return new time context or {@code null} if given data are insufficient
* @throws IllegalArgumentException in any case of inconsistent data
* @see ValidationElement#ERROR_MESSAGE
* @since 3.15/4.12
*/
default T createFrom(
ChronoEntity> entity,
AttributeQuery attributes,
boolean lenient,
boolean preparsing
) {
return this.createFrom(entity, attributes, preparsing);
}
/**
* Transforms the current context/entity into another set of chronological
* values which finally shall be formatted using given attributes.
*
* @param context actual chronological context to be formatted
* @param attributes controls attributes during formatting
* @return replacement entity which will finally be used for formatting
* @throws IllegalArgumentException in any case of inconsistent data
*/
/*[deutsch]
* Transformiert den aktuellen Kontext unter Beachtung der Attribute
* bei Bedarf in den tatsächlich zu formatierenden Satz von
* chronologischen Werten.
*
* @param context actual chronological context to be formatted
* @param attributes controls attributes during formatting
* @return replacement entity which will finally be used for formatting
* @throws IllegalArgumentException in any case of inconsistent data
*/
default ChronoDisplay preformat(
T context,
AttributeQuery attributes
) {
try {
return (ChronoDisplay) context;
} catch (ClassCastException cce) {
throw new IllegalArgumentException(cce.getMessage());
}
}
/**
* This method defines a child chronology which can preparse
* a chronological text.
*
* @return preparsing chronology or {@code null} (default)
*/
/*[deutsch]
* Diese Methode definiert eine Kindschronologie, wenn eine
* Vorinterpretierung des chronologischen Texts notwendig ist.
*
* @return preparsing chronology or {@code null} (default)
*/
default Chronology> preparser() {
return null;
}
/**
* Creates a new entity of type T based on given chronological
* data.
*
* The default implementation always returns {@code null} so subclasses
* with better knowledge about their own state and needs should override it.
*
* @param threeten object of type {@code TemporalAccessor}
* @param attributes configuration attributes given by parser
* @return new time context or {@code null} if given data are insufficient
* @throws IllegalArgumentException in any case of inconsistent data
* @since 4.0
* @deprecated Use new {@link BridgeChronology bridge chronology}
*/
/*[deutsch]
* Konstruiert eine neue Entität basierend auf den angegebenen
* chronologischen Daten.
*
* Die Standardimplementierung liefert immer {@code null}, so daß
* Subklassen mit besserer Kenntnis ihres Zustands und ihrer Anforderungen
* diese Methode überschreiben sollten.
*
* @param threeten object of type {@code TemporalAccessor}
* @param attributes configuration attributes given by parser
* @return new time context or {@code null} if given data are insufficient
* @throws IllegalArgumentException in any case of inconsistent data
* @since 4.0
* @deprecated Use new {@link BridgeChronology bridge chronology}
*/
@Deprecated
default T createFrom(
TemporalAccessor threeten,
AttributeQuery attributes
) {
return null;
}
/**
* Defines a CLDR-compatible localized format pattern.
*
* @param style format style
* @param locale language and country setting
* @return localized format pattern
* @throws UnsupportedOperationException if given style is not supported
* or if no localized format pattern support is available
* @see net.time4j.format.LocalizedPatternSupport
* @since 3.10/4.7
*/
/*[deutsch]
* Definiert ein CLDR-kompatibles lokalisiertes Formatmuster.
*
* @param style format style
* @param locale language and country setting
* @return localized format pattern
* @throws UnsupportedOperationException if given style is not supported
* or if no localized format pattern support is available
* @see net.time4j.format.LocalizedPatternSupport
* @since 3.10/4.7
*/
default String getFormatPattern(
DisplayStyle style,
Locale locale
) {
throw new UnsupportedOperationException("Localized format patterns are not available.");
}
/**
* Determines the default start of day.
*
* @return start of day
* @since 3.11/4.8
*/
/*[deutsch]
* Bestimmt den üblichen Tagesbeginn.
*
* @return start of day
* @since 3.11/4.8
*/
default StartOfDay getDefaultStartOfDay() {
return StartOfDay.MIDNIGHT;
}
/**
* Determines the default pivot year which might be calendar specific and serves for the
* formatting of two-digit-years.
*
* Most calendar chronologies should choose a pivot year 20 years in the future. The standard
* implementation is based on the gregorian calendar.
*
* @return default pivot year (must not be smaller than {@code 100})
* @since 3.32/4.27
*/
/*[deutsch]
* Bestimmt das übliche kalender-spezifische Kippjahr, das zur Formatierung
* von zweistelligen Jahresangaben dient.
*
* Die meisten Kalenderchronologien sollten ein Kippjahr 20 Jahre in der Zukunft wählen.
* Die Standardimplementierung basiert auf dem gregorianischen Kalender.
*
* @return default pivot year (must not be smaller than {@code 100})
* @since 3.32/4.27
*/
default int getDefaultPivotYear() {
return IsoDefaultPivotYear.VALUE;
}
}