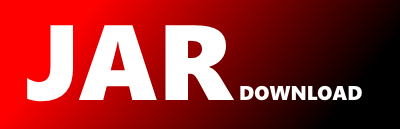
net.time4j.format.NumberSymbolProvider Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2016 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (NumberSymbolProvider.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.format;
import java.text.DecimalFormatSymbols;
import java.util.Locale;
/**
* This SPI-interface enables the access to localized
* number properties like zero digits and is instantiated via a
* {@code ServiceLoader}-mechanism.
*
* If there is no external {@code NumberSymbolProvider} then Time4J will
* just delegate to the internal resources or to the JDK.
*
* @author Meno Hochschild
* @since 2.1
* @see java.util.ServiceLoader
* @see java.text.DecimalFormatSymbols#getZeroDigit
* @doctags.spec Implementations must have a public no-arg constructor.
*/
/*[deutsch]
* Dieses SPI-Interface ermöglicht den Zugriff
* auf {@code Locale}-abhängige Zahleigenschaften wie Nullziffern
* und wird über einen {@code ServiceLoader}-Mechanismus instanziert.
*
* Wird kein externer {@code NumberSymbolProvider} gefunden, wird intern
* eine Instanz erzeugt, die an die internen Ressourcen oder das JDK delegiert.
*
* @author Meno Hochschild
* @since 2.1
* @see java.util.ServiceLoader
* @see java.text.DecimalFormatSymbols#getZeroDigit
* @doctags.spec Implementations must have a public no-arg constructor.
*/
public interface NumberSymbolProvider {
//~ Statische Felder/Initialisierungen --------------------------------
/**
* Default provider which delegates to standard JVM resources.
*
* @see DecimalFormatSymbols
*/
/*[deutsch]
* Standardimplementierung, die an die Ressourcen der JVM delegiert.
*
* @see DecimalFormatSymbols
*/
NumberSymbolProvider DEFAULT =
new NumberSymbolProvider() {
@Override
public Locale[] getAvailableLocales() {
return DecimalFormatSymbols.getAvailableLocales();
}
@Override
public char getZeroDigit(Locale locale) {
return getSymbols(locale).getZeroDigit();
}
@Override
public char getDecimalSeparator(Locale locale) {
return getSymbols(locale).getDecimalSeparator();
}
@Override
public String getPlusSign(Locale locale) {
if (locale.getLanguage().equals("ar")) {
return "\u200F+";
}
return String.valueOf('+');
}
@Override
public String getMinusSign(Locale locale) {
if (locale.getLanguage().equals("ar")) {
return "\u200F\u002D";
}
return String.valueOf(getSymbols(locale).getMinusSign());
}
private DecimalFormatSymbols getSymbols(Locale loc) {
return DecimalFormatSymbols.getInstance(loc);
}
};
//~ Methoden ----------------------------------------------------------
/**
* Yields the supported languages.
*
* @return Locale-array
*/
/*[deutsch]
* Gibt die unterstützten Sprachen an.
*
* @return Locale-array
*/
Locale[] getAvailableLocales();
/**
* Returns the localized zero digit.
*
* @param locale language and country setting
* @return zero digit of associated numbering system
*/
/*[deutsch]
* Liefert die lokalisierte Nullziffer.
*
* @param locale language and country setting
* @return zero digit of associated numbering system
*/
char getZeroDigit(Locale locale);
/**
* Returns the localized decimal separator.
*
* @param locale language and country setting
* @return localized decimal separator
*/
/*[deutsch]
* Liefert das lokalisierte Dezimaltrennzeichen.
*
* @param locale language and country setting
* @return localized decimal separator
*/
char getDecimalSeparator(Locale locale);
/**
* Returns the localized plus sign.
*
* @param locale language and country setting
* @return localized plus sign, possibly including RLM- or LRM-markers
* @since 3.13/4.10
*/
/*[deutsch]
* Liefert das lokalisierte Plus-Zeichen.
*
* @param locale language and country setting
* @return localized plus sign, possibly including RLM- or LRM-markers
* @since 3.13/4.10
*/
default String getPlusSign(Locale locale) {
return String.valueOf('+');
}
/**
* Returns the localized minus sign.
*
* @param locale language and country setting
* @return localized minus sign, possibly including RLM- or LRM-markers
*/
/*[deutsch]
* Liefert das lokalisierte Minus-Zeichen.
*
* @param locale language and country setting
* @return localized minus sign, possibly including RLM- or LRM-markers
*/
String getMinusSign(Locale locale);
/**
* Obtains the standard number system for given locale.
*
* @param locale language and country setting
* @return default number system
* @since 3.23/4.19
*/
/*[deutsch]
* Ermitelt das Standardzahlsystem für die angegebene Sprache.
*
* @param locale language and country setting
* @return default number system
* @since 3.23/4.19
*/
default NumberSystem getDefaultNumberSystem(Locale locale) {
return NumberSystem.ARABIC; // worldwide standard
}
}