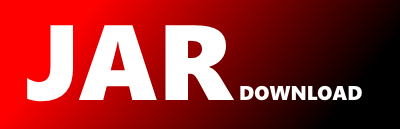
net.time4j.format.TextProvider Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2017 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (TextProvider.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.format;
import java.util.Locale;
import java.util.ResourceBundle;
/**
* This SPI-interface enables the access to calendrical
* standard text informations and will be instantiated by a
* {@code ServiceLoader}-mechanism.
*
* The motivation is mainly to override the language-dependent forms
* of JDK-defaults with respect to standard elements like months, weekdays
* etc. Specific text forms which are not contained in JDK will instead
* be supplied by help of properties-files in the "data"-folder.
*
*
* @author Meno Hochschild
* @since 2.0
* @doctags.spec Implementations must have a public no-arg constructor.
* @see java.util.ServiceLoader
*/
/*[deutsch]
* Dieses SPI-Interface ermöglicht den Zugriff
* auf kalendarische Standard-Textinformationen und wird über einen
* {@code ServiceLoader}-Mechanismus instanziert.
*
* Sinn und Zweck dieses Interface ist in erster Linie das sprachliche
* Ergänzen oder Überschreiben von JDK-Vorgaben bezüglich
* der Standardelemente Monat, Wochentag etc. Kalenderspezifische Texte,
* die gar nicht im JDK vorhanden sind, werden stattdessen mit Hilfe von
* properties-Dateien im data-Verzeichnis bereitgestellt.
*
* @author Meno Hochschild
* @since 2.0
* @doctags.spec Implementations must have a public no-arg constructor.
* @see java.util.ServiceLoader
*/
public interface TextProvider {
//~ Methoden ----------------------------------------------------------
/**
* Queries if a calendar type is supported by this text provider.
*
* @param calendarType the calendar type to be checked
* @return {@code true} if given calendar type is supported else {@code false}
* @since 3.32/4.27
* @see CalendarType
*/
/*[deutsch]
* Fragt die Unterstützung eines Kalendertyps durch diesen {@code TextProvider} ab.
*
* @param calendarType the calendar type to be checked
* @return {@code true} if given calendar type is supported else {@code false}
* @since 3.32/4.27
* @see CalendarType
*/
boolean supportsCalendarType(String calendarType);
/**
* Queries if a language is supported by this text provider.
*
* @param language the language to be checked
* @return {@code true} if given language is supported else {@code false}
* @since 3.32/4.27
*/
/*[deutsch]
* Fragt die Unterstützung einer Sprache durch diesen {@code TextProvider} ab.
*
* @param language the language to be checked
* @return {@code true} if given language is supported else {@code false}
* @since 3.32/4.27
*/
boolean supportsLanguage(Locale language);
/**
* Defines the supported calendar types.
*
* @return String-array with calendar types
* @see CalendarType
*/
/*[deutsch]
* Definiert die unterstützten Kalendertypen.
*
* @return String-array with calendar types
* @see CalendarType
*/
String[] getSupportedCalendarTypes();
/**
* Yields the supported languages.
*
* Only the language part will be evaluated.
*
* @return Locale-array
* @see Locale#getLanguage()
*/
/*[deutsch]
* Gibt die unterstützten Sprachen an.
*
* Nur der reine Sprachencode wird ausgewertet.
*
* @return Locale-array
* @see Locale#getLanguage()
*/
Locale[] getAvailableLocales();
/**
* See {@link CalendarText#getStdMonths}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @param outputContext output context
* @param leapForm use leap form (for example the hebrew
* month "Adar II")?
* @return unmodifiable sorted array of month names
*/
/*[deutsch]
* Siehe {@link CalendarText#getStdMonths}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @param outputContext output context
* @param leapForm use leap form (for example the hebrew
* month "Adar II")?
* @return unmodifiable sorted array of month names
*/
String[] months(
String calendarType,
Locale locale,
TextWidth textWidth,
OutputContext outputContext,
boolean leapForm
);
/**
* See {@link CalendarText#getQuarters}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @param outputContext output context
* @return unmodifiable sorted array of quarter names
*/
/*[deutsch]
* Siehe {@link CalendarText#getQuarters}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @param outputContext output context
* @return unmodifiable sorted array of quarter names
*/
String[] quarters(
String calendarType,
Locale locale,
TextWidth textWidth,
OutputContext outputContext
);
/**
* See {@link CalendarText#getWeekdays}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @param outputContext output context
* @return unmodifiable sorted array of weekday names
* in calendar specific order (ISO-8601 starts with monday)
*/
/*[deutsch]
* Siehe {@link CalendarText#getWeekdays}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @param outputContext output context
* @return unmodifiable sorted array of weekday names
* in calendar specific order (ISO-8601 starts with monday)
*/
String[] weekdays(
String calendarType,
Locale locale,
TextWidth textWidth,
OutputContext outputContext
);
/**
* See {@link CalendarText#getEras}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @return unmodifiable sorted array of era names
*/
/*[deutsch]
* Siehe {@link CalendarText#getEras}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @return unmodifiable sorted array of era names
*/
String[] eras(
String calendarType,
Locale locale,
TextWidth textWidth
);
/**
* See {@link CalendarText#getMeridiems}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @return unmodifiable sorted array of AM/PM-names
* @see #meridiems(String, Locale, TextWidth, OutputContext)
* @deprecated Will be removed in next major release!
*/
/*[deutsch]
* Siehe {@link CalendarText#getMeridiems}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @return unmodifiable sorted array of AM/PM-names
* @see #meridiems(String, Locale, TextWidth, OutputContext)
* @deprecated Will be removed in next major release!
*/
String[] meridiems(
String calendarType,
Locale locale,
TextWidth textWidth
);
/**
* See {@link CalendarText#getMeridiems}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @param outputContext output context
* @return unmodifiable sorted array of AM/PM-names
*/
/*[deutsch]
* Siehe {@link CalendarText#getMeridiems}.
*
* @param calendarType calendar type
* @param locale language of text output
* @param textWidth text width
* @param outputContext output context
* @return unmodifiable sorted array of AM/PM-names
*/
default String[] meridiems(
String calendarType,
Locale locale,
TextWidth textWidth,
OutputContext outputContext
) {
return this.meridiems(calendarType, locale, textWidth);
}
/**
* Returns a suitable object for controlling access to resources.
*
* @return helper object for accessing resources
* @since 2.2
*/
/*[deutsch]
* Liefert ein geeignetes Hilfsobjekt zur fein-granularen Kontrolle
* des Zugangs zu Ressourcen.
*
* @return helper object for accessing resources
* @since 2.2
*/
ResourceBundle.Control getControl();
}