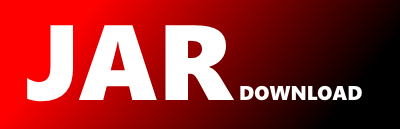
net.time4j.tz.TZID Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------
* Copyright © 2013-2016 Meno Hochschild,
* -----------------------------------------------------------------------
* This file (TZID.java) is part of project Time4J.
*
* Time4J is free software: You can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 2.1 of the License, or
* (at your option) any later version.
*
* Time4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Time4J. If not, see .
* -----------------------------------------------------------------------
*/
package net.time4j.tz;
/**
* Identifies a timezone.
*
* In most cases, the timezone ID has the Olson-format
* "{region}/{city}" or is an offset in the format
* "UTC±hh:mm". In latter case applications can
* instead directly use an instance of type {@code ZonalOffset},
* especially if the timezone offset for a given timepoint is
* already known.
*
* Provider-specific keys have the
* {@link ZoneModelProvider#getName() name} of the provider followed by the
* separator char "~" and the normal canonical identifier. This
* form can be used if a custom registered {@link ZoneModelProvider} shall be
* used instead of the default provider for lookup of the zonal data. For
* example following key will search the timezone data via the API of
* {@code java.util.TimeZone} even if there is configured another default
* zone provider: "java.util.TimeZone~Europe/Berlin"
*
* Lexical comparisons of IDs should always be done by the method
* {@link #canonical()} because an object of type {@code TZID} is only
* designed for encapsulating a canonical name. The comparison
* using the method {@code equals()} is not allowed.
*
* If a timezone offset is known for historical timezones before the year
* 1970 then users should generally prefer the class {@code ZonalOffset} because
* the timezone data associated with the enum constants are not necessarily
* correct.
*
* Specification:
* All implementations must be immutable, thread-safe and serializable.
*
* @author Meno Hochschild
*/
/*[deutsch]
* Identifiziert eine Zeitzone.
*
* Meistens liegt die ID im Olson-Format "{region}/{city}" oder
* als Offset-Angabe im Format "UTC±hh:mm" vor. In letzterem
* Fall kann und sollte auch direkt ein Objekt des Typs {@code ZonalOffset}
* in Betracht gezogen werden, insbesondere dann, wenn eine Verschiebung
* zu einem gegebenen Zeitpunkt schon bekannt ist.
*
* Provider-spezifische Schlüssel haben den
* {@link ZoneModelProvider#getName() Namen} des {@code ZoneModelProvider} gefolgt
* von der Tilde "~" und der normalen kanonischen ID. Diese
* Form kann verwendet werden, wenn ein registrierter benutzerdefinierter
* {@link ZoneModelProvider} an Stelle des Standard-Provider für die
* Suche nach den Zeitzonendaten herangezogen werden soll. Zum Beispiel
* wird folgender Schlüssel die Zeitzonendaten über das API von
* {@code java.util.TimeZone} suchen, sogar wenn ein anderer Standard-Provider
* eingestellt ist:
* "java.util.TimeZone~Europe/Berlin"
*
* Ein (lexikalischer) Vergleich von IDs sollte immer über die Methode
* {@link #canonical()} gemacht werden, weil ein {@code TZID} nur dem Zweck
* dient, einen kanonischen Namen zu kapseln. Der Vergleich über
* die Objekt-Methode {@code equals()} ist nicht erlaubt.
*
* Falls für historische Zeitangaben vor dem Jahr 1970 ein Offset
* wohlbekannt ist, ist generell der Klasse {@code ZonalOffset} der Vorzug
* vor den Enum-Konstanten zu geben, weil die mit den Enums verknüpften
* historischen Zeitzonendaten nicht notwendig korrekt sein müssen.
*
* Specification:
* All implementations must be immutable, thread-safe and serializable.
*
* @author Meno Hochschild
*/
public interface TZID {
//~ Methoden ----------------------------------------------------------
/**
* Represents the full canonical name of a timezone (for
* example "Europe/Paris" or "UTC+01:00").
*
* @return String in TZDB format (Olson-ID) or in canonical offset format
*/
/*[deutsch]
* Repräsentiert den vollständigen kanonischen Namen
* einer Zeitzone (zum Beispiel "Europe/Paris" oder
* "UTC+01:00").
*
* @return String in TZDB format (Olson-ID) or in canonical offset format
*/
String canonical();
}