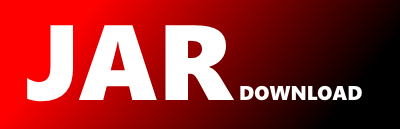
net.tirasa.connid.commons.db.PropertiesResolver Maven / Gradle / Ivy
/*
* ====================
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
*
* Copyright 2008-2009 Sun Microsystems, Inc. All rights reserved.
*
* The contents of this file are subject to the terms of the Common Development
* and Distribution License("CDDL") (the "License"). You may not use this file
* except in compliance with the License.
*
* You can obtain a copy of the License at
* http://opensource.org/licenses/cddl1.php
* See the License for the specific language governing permissions and limitations
* under the License.
*
* When distributing the Covered Code, include this CDDL Header Notice in each file
* and include the License file at http://opensource.org/licenses/cddl1.php.
* If applicable, add the following below this CDDL Header, with the fields
* enclosed by brackets [] replaced by your own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
* ====================
* Portions Copyrighted 2011 ConnId.
*/
package net.tirasa.connid.commons.db;
import java.util.*;
/**
* Resolver of properties in UNIX/ant style.
* Example of usage :
*
* Properties p = new Properties();
* p.setProperty("p1","value1");
* p.setProperty("p2","Value of p1 is ${p1}");
* p = PropertiesResolver.resolveProperties(p);
*
*
* It is shield against recursion.
*
* @author kitko
*
*/
public class PropertiesResolver {
private PropertiesResolver() {
//empty
}
/** Resolves properties
*
* @param properties properties we want to resolve
* @param resolvedProperties already known properties
* @return resolved properties
*/
public static Properties resolveProperties(Properties properties, Properties resolvedProperties) {
if (properties == null) {
return null;
}
return resolveProperties(copyProperties(properties), copyProperties(resolvedProperties), new HashSet(5));
}
/**
* Resolve properties containing already known values
*
* @param properties
* @return resolved properties
*/
public static Properties resolveProperties(Properties properties) {
if (properties == null) {
return null;
}
Properties copy = copyProperties(properties);
return resolveProperties(copy, new Properties(), new HashSet(5));
}
private static Properties resolveProperties(Properties properties, Properties resolvedProperties,
Set justResolving) {
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy