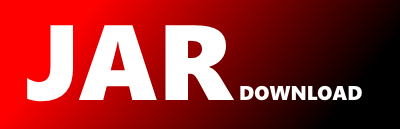
net.urosk.mifss.services.ApiKeyServiceImpl Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
**************************************************************************
* MIFSS - content storage system
*
*
* @uthors: [email protected] (Uroš Kristan ) Urosk.NET
* [email protected] (Jernej Švigelj)
*/
package net.urosk.mifss.services;
import net.urosk.mifss.configurations.pojos.ApiKey;
import net.urosk.mifss.configurations.pojos.ApiMode;
import net.urosk.mifss.lib.exceptions.ApiKeyHandlerException;
import net.urosk.mifss.services.interfaces.ApiKeyService;
import org.apache.commons.codec.digest.DigestUtils;
import org.apache.commons.lang.StringUtils;
import org.springframework.stereotype.Component;
import org.springframework.transaction.annotation.Transactional;
import java.util.Date;
import java.util.Set;
import java.util.UUID;
@Component
public class ApiKeyServiceImpl implements ApiKeyService {
@Override
@Transactional
public ApiKey verifyApiKey(Set availableKeys, String apiKeyHashToBeVefified, ApiMode apiMode) throws ApiKeyHandlerException {
ApiKey apiKey = null;
for (ApiKey ak : availableKeys) {
if (apiKeyHashToBeVefified.equals(ak.getHash())) {
apiKey = ak;
break;
}
}
if (apiKey == null) {
throw new ApiKeyHandlerException("Api key " + apiKeyHashToBeVefified + " not found!");
} else if (apiMode != apiKey.getApiMode()) {
throw new ApiKeyHandlerException("Api key " + apiKeyHashToBeVefified + " has incorrect api mode!");
}
return apiKey;
}
@Override
public ApiKey generateNewApiKey(String name, String description, ApiMode apiMode) throws ApiKeyHandlerException {
// api key name is mandatory
if (StringUtils.isEmpty(name)) {
throw new ApiKeyHandlerException("Name must be entered");
}
// api mode must be explicitly entered
if (apiMode == null) {
throw new ApiKeyHandlerException("Api mode must be entered! Possible values are: " + ApiMode.values());
}
String hash = DigestUtils.sha1Hex("secret x" + (new Date().getTime()) + "mifss" + UUID.randomUUID() + Math.random());
ApiKey apiKey = new ApiKey();
apiKey.setApiMode(apiMode);
apiKey.setDescription(description);
apiKey.setHash(hash);
apiKey.setName(name);
return apiKey;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy