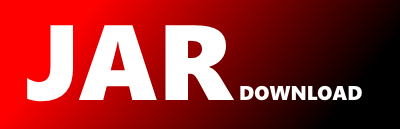
net.vidageek.mirror.ClassController Maven / Gradle / Ivy
/**
*
*/
package net.vidageek.mirror;
import net.vidageek.mirror.get.GetterHandler;
import net.vidageek.mirror.invoke.InvocationHandler;
import net.vidageek.mirror.provider.ReflectionProvider;
import net.vidageek.mirror.reflect.AllReflectionHandler;
import net.vidageek.mirror.reflect.ReflectionHandler;
import net.vidageek.mirror.set.SetterHandler;
/**
* Object to provide reflection for a Class object
*
* @author jonasabreu
*/
public final class ClassController {
private final Class clazz;
private final ReflectionProvider provider;
public ClassController(final ReflectionProvider provider, final Class clazz) {
this.provider = provider;
if (clazz == null) {
throw new IllegalArgumentException("clazz cannot be null");
}
this.clazz = clazz;
}
/**
* This part of the DSL controls invocation of methods and constructors.
*
* @return An object to control invocation.
*/
public InvocationHandler invoke() {
return new InvocationHandler(provider, clazz);
}
/**
* This part of the DSL controls setting of fields.
*
* @return An object to control setting.
*/
public SetterHandler set() {
return new SetterHandler(provider, clazz);
}
/**
* This part of the DSL controls getting field values.
*
* @return An object to control getting of values.
*/
public GetterHandler get() {
return new GetterHandler(provider, clazz);
}
/**
* This part of the DSL controls reflection of single reflection elements.
*
* @return An object to control reflection of single reflection elements.
*/
public ReflectionHandler reflect() {
return new ReflectionHandler(provider, clazz);
}
/**
* This part of the DSL controls reflection of lists of reflection elements.
*
* @return An object to control reflection of lists of reflection elements.
*/
public AllReflectionHandler reflectAll() {
return new AllReflectionHandler(provider, clazz);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy