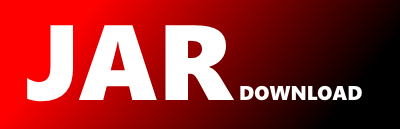
net.vidageek.mirror.invoke.ConstructorHandlerByConstructor Maven / Gradle / Ivy
/**
*
*/
package net.vidageek.mirror.invoke;
import java.lang.reflect.Constructor;
import net.vidageek.mirror.invoke.dsl.ConstructorHandler;
import net.vidageek.mirror.provider.ConstructorReflectionProvider;
import net.vidageek.mirror.provider.ReflectionProvider;
/**
* This class is responsible for invoking a constructor provided.
*
* @author jonasabreu
*/
public final class ConstructorHandlerByConstructor implements ConstructorHandler {
private final Constructor constructor;
private final Class clazz;
private final ReflectionProvider provider;
public ConstructorHandlerByConstructor(final ReflectionProvider provider, final Class clazz,
final Constructor con) {
if (clazz == null) {
throw new IllegalArgumentException("clazz cannot be null");
}
if (con == null) {
throw new IllegalArgumentException("constructor cannot be null");
}
if (!clazz.equals(con.getDeclaringClass())) {
throw new IllegalArgumentException("constructor declaring type should be " + clazz.getName() + " but was "
+ con.getDeclaringClass().getName());
}
this.provider = provider;
this.clazz = clazz;
this.constructor = con;
}
/**
* {@inheritDoc ConstructorHandler#withArgs(Object...)}
*
* @see ConstructorReflectionProvider#setAccessible()
* @see ConstructorReflectionProvider#instantiate(Object...)
*/
public T withArgs(final Object... args) {
ConstructorReflectionProvider constructorReflectionProvider = provider.getConstructorReflectionProvider(
clazz, constructor);
constructorReflectionProvider.setAccessible();
return constructorReflectionProvider.instantiate(args);
}
/**
* {@inheritDoc ConstructorHandler#withoutArgs()}
*
* This is a convenience method for
* {@link ConstructorHandlerByConstructor#withArgs(Object...)}
*
* @see ConstructorHandlerByConstructor#withArgs(Object...)
*/
public T withoutArgs() {
return withArgs(new Object[0]);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy