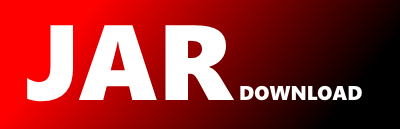
net.vieiro.toml.TOML Maven / Gradle / Ivy
/*
* Copyright 2023 Antonio Vieiro .
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.vieiro.toml;
import java.io.IOException;
import java.io.PrintStream;
import java.io.PrintWriter;
import java.io.Writer;
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
/**
* TOML is the result from parsing a TOML file. This class includes some methods
* for querying the generated object-tree, for more advanced querying consider
* using a specific object-tree querying library such as OGNL, Apache Commons
* EL, etc.
*/
public final class TOML {
final Map root;
final List errors;
TOML(Map root, List errors) {
this.root = root;
this.errors = Collections.unmodifiableList(errors);
}
/**
* Returns a list of the syntax errors generated during parsing.
*
* @return A list of errors generated during parsing.
*/
public List getErrors() {
return errors;
}
/**
* Returns the root table representing the document.
*
* @return The root table representing the parsed TOML document.
*/
public Map getRoot() {
return root;
}
/**
* Returns a new TOML subtree given a prefix.
* @param prefix The prefix.
* @return A TOML object to query the subtree.
*/
public TOML subtree(String prefix) {
Map subtree = getTable(prefix).orElse(Collections.emptyMap());
return new TOML(subtree, errors);
}
/**
* Prints out the object tree. For debugging purposes.
*
* @return A String representation of the object tree.
*/
@Override
public String toString() {
return root == null ? "null" : root.toString();
}
@SuppressWarnings("unchecked")
private Optional get(String path, Class clazz) {
return TOMLSimpleQuery.get(root, path, clazz);
}
/**
* Retrieves a String value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The String value, if any, or Optional.empty() otherwise.
*/
public Optional getString(String path) {
return get(path, String.class);
}
/**
* Retrieves a Long value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The Long value, if any, or Optional.empty() otherwise.
*/
public Optional getLong(String path) {
return get(path, Long.class);
}
/**
* Retrieves a Double value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The Double value, if any, or Optional.empty() otherwise.
*/
public Optional getDouble(String path) {
return get(path, Double.class);
}
/**
* Retrieves a List value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The List value, if any, or Optional.empty() otherwise.
*/
public Optional getArray(String path) {
return get(path, List.class);
}
/**
* Retrieves a Boolean value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The Boolean value, if any, or Optional.empty() otherwise.
*/
public Optional getBoolean(String path) {
return get(path, Boolean.class);
}
/**
* Retrieves a Instant value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The Instant value, if any, or Optional.empty() otherwise.
*/
public Optional getInstant(String path) {
return get(path, Instant.class);
}
/**
* Retrieves a OffsetDateTime value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The OffsetDateTime value, if any, or Optional.empty() otherwise.
*/
public Optional getOffsetDateTime(String path) {
return get(path, OffsetDateTime.class);
}
/**
* Retrieves a LocalDateTime value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The LocalDateTime value, if any, or Optional.empty() otherwise.
*/
public Optional getLocalDateTime(String path) {
return get(path, LocalDateTime.class);
}
/**
* Retrieves a LocalDate value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The LocalDate value, if any, or Optional.empty() otherwise.
*/
public Optional getLocalDate(String path) {
return get(path, LocalDate.class);
}
/**
* Retrieves a LocalTime value from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The LocalTime value, if any, or Optional.empty() otherwise.
*/
public Optional getLocalTime(String path) {
return get(path, LocalTime.class);
}
/**
* Retrieves a table from this TOML object.
*
* @param path The path, separated by forward slashes, as in "a/b/c"
* @return The Map stored in the given path, or Optional.empty() otherwise.
*/
@SuppressWarnings("unchecked")
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy