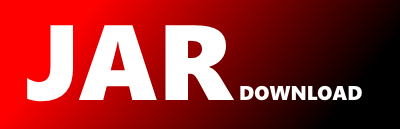
net.vieiro.toml.antlr4.TOMLAntlrParserVisitor Maven / Gradle / Ivy
// Generated from net/vieiro/toml/antlr4/TOMLAntlrParser.g4 by ANTLR 4.13.1
package net.vieiro.toml.antlr4;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link TOMLAntlrParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface TOMLAntlrParserVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#document}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDocument(TOMLAntlrParser.DocumentContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpression(TOMLAntlrParser.ExpressionContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#comment}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComment(TOMLAntlrParser.CommentContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#key_value}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitKey_value(TOMLAntlrParser.Key_valueContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#key}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitKey(TOMLAntlrParser.KeyContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#simple_key}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSimple_key(TOMLAntlrParser.Simple_keyContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#unquoted_key}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnquoted_key(TOMLAntlrParser.Unquoted_keyContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#quoted_key}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuoted_key(TOMLAntlrParser.Quoted_keyContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#dotted_key}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDotted_key(TOMLAntlrParser.Dotted_keyContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#value}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValue(TOMLAntlrParser.ValueContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#string}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitString(TOMLAntlrParser.StringContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#integer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInteger(TOMLAntlrParser.IntegerContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#floating_point}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFloating_point(TOMLAntlrParser.Floating_pointContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#bool_}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBool_(TOMLAntlrParser.Bool_Context ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#date_time}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDate_time(TOMLAntlrParser.Date_timeContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#inline_table}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInline_table(TOMLAntlrParser.Inline_tableContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#inner_array}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInner_array(TOMLAntlrParser.Inner_arrayContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#inline_value}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInline_value(TOMLAntlrParser.Inline_valueContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#array_}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArray_(TOMLAntlrParser.Array_Context ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#array_values}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArray_values(TOMLAntlrParser.Array_valuesContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#comment_or_nl}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComment_or_nl(TOMLAntlrParser.Comment_or_nlContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#table}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTable(TOMLAntlrParser.TableContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#standard_table}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStandard_table(TOMLAntlrParser.Standard_tableContext ctx);
/**
* Visit a parse tree produced by {@link TOMLAntlrParser#array_table}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArray_table(TOMLAntlrParser.Array_tableContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy