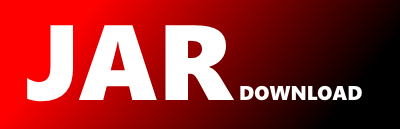
net.vrallev.android.ecc.Ecc25519Helper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecc-25519-android-facade Show documentation
Show all versions of ecc-25519-android-facade Show documentation
A library for Java and Android to use Ed25519 and Curve25518.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy