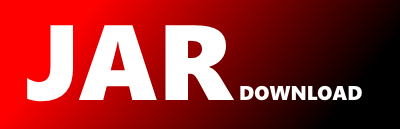
net.welen.jmole.protocols.zabbix.Zabbix Maven / Gradle / Ivy
package net.welen.jmole.protocols.zabbix;
/*
* #%L
* JMole, https://bitbucket.org/awelen/jmole
* %%
* Copyright (C) 2015 - 2017 Anders Welén, [email protected]
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.net.InetAddress;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.quigley.zabbixj.agent.ZabbixAgent;
import net.welen.jmole.JMole;
public class Zabbix implements ZabbixMBean {
private final static Logger LOG = Logger.getLogger(Zabbix.class.getName());
private static String PROPERTY_ZABBIX_ENABLED = "jmole.protocol.zabbix.enabled";
private static String PROPERTY_ZABBIX_PASSIVE = "jmole.protocol.zabbix.passive";
private static String PROPERTY_ZABBIX_LISTEN_PORT = "jmole.protocol.zabbix.listen.port";
private static String PROPERTY_ZABBIX_LISTEN_ADDRESS = "jmole.protocol.zabbix.listen.adress";
private static String PROPERTY_ZABBIX_ACTIVE = "jmole.protocol.zabbix.active";
private static String PROPERTY_ZABBIX_HOSTNAME = "jmole.protocol.zabbix.hostname";
private static String PROPERTY_ZABBIX_SERVER_ADDRESS = "jmole.protocol.zabbix.server.adress";
private static String PROPERTY_ZABBIX_SERVER_PORT = "jmole.protocol.zabbix.server.port";
private Boolean passive;
private Integer listenPort;
private String listenAddress;
private Boolean active;
private String hostName;
private String serverAddress;
private Integer serverPort;
protected JMole jmole;
private ZabbixAgent agent = null;
public boolean isEnabled() {
return Boolean.getBoolean(PROPERTY_ZABBIX_ENABLED);
}
public void setListenPort(Integer listenPort) {
this.listenPort = listenPort;
}
public Integer getListenPort() {
return listenPort;
}
public void setActive(Boolean active) {
this.active = active;
}
public Boolean getActive() {
return active;
}
public void setHostName(String hostName) {
this.hostName = hostName;
}
public String getHostName() {
return hostName;
}
public void setServerAddress(String serverAddress) {
this.serverAddress = serverAddress;
}
public String getServerAddress() {
return serverAddress;
}
public void setServerPort(Integer serverPort) {
this.serverPort = serverPort;
}
public Integer getServerPort() {
return serverPort;
}
public void setPassive(Boolean passive) {
this.passive = passive;
}
public Boolean getPassive() {
return passive;
}
public void setListenAddress(String listenAddress) {
this.listenAddress = listenAddress;
}
public String getListenAddress() {
return listenAddress;
}
@Override
public void startProtocol(JMole jmole) throws Exception {
this.jmole = jmole;
if (System.getProperty(PROPERTY_ZABBIX_PASSIVE) == null) {
passive = true;
} else {
passive = Boolean.getBoolean(PROPERTY_ZABBIX_PASSIVE);
}
listenPort = Integer.getInteger(PROPERTY_ZABBIX_LISTEN_PORT);
if (listenPort == null) {
listenPort = 10050;
}
listenAddress = System.getProperty(PROPERTY_ZABBIX_LISTEN_ADDRESS);
if (listenAddress == null) {
listenAddress = "0.0.0.0";
}
active = Boolean.getBoolean(PROPERTY_ZABBIX_ACTIVE);
hostName = System.getProperty(PROPERTY_ZABBIX_HOSTNAME);
if (hostName == null) {
hostName = "JMole";
}
serverAddress = System.getProperty(PROPERTY_ZABBIX_SERVER_ADDRESS);
if (serverAddress == null) {
serverAddress = "localhost";
}
serverPort = Integer.getInteger(PROPERTY_ZABBIX_SERVER_PORT);
if (serverPort == null) {
serverPort = 10051;
}
LOG.log(Level.INFO, "JMole Zabbix protocol starting.");
LOG.log(Level.FINE, "Setup: " + passive + ", " + listenAddress + ", " + listenPort + ", " + active + ", " + hostName + ", " + serverAddress + ", " + serverPort);
agent = new ZabbixAgent();
// Setup passive
agent.setEnablePassive(passive);
if (passive) {
agent.setListenPort(listenPort);
agent.setListenAddress(listenAddress);
}
// Setup active
agent.setEnableActive(active);
if (active) {
agent.setHostName(hostName);
agent.setServerAddress(InetAddress.getByName(serverAddress));
agent.setServerPort(serverPort);
}
// Add providers
agent.addProvider("jmole", new JMoleMetricsProvider(jmole));
// Start service
agent.start();
LOG.log(Level.INFO, "JMole Zabbix started");
}
@Override
public void stopProtocol() throws Exception {
if (agent != null) {
agent.stop();
}
LOG.info("JMole Zabbix stopped.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy