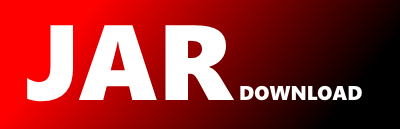
net.welen.jmole.protocols.nrpe.GetValueJNRPEPlugin Maven / Gradle / Ivy
package net.welen.jmole.protocols.nrpe;
import java.util.Map;
import java.util.Map.Entry;
/*
* #%L
* JMole, https://bitbucket.org/awelen/jmole
* %%
* Copyright (C) 2015 - 2018 Anders Welén, [email protected]
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.logging.Level;
import java.util.logging.Logger;
import net.welen.jmole.JMole;
import it.jnrpe.ICommandLine;
import it.jnrpe.ReturnValue;
import it.jnrpe.Status;
import it.jnrpe.plugins.IPluginInterface;
import it.jnrpe.utils.BadThresholdException;
import it.jnrpe.utils.ThresholdUtil;
public class GetValueJNRPEPlugin implements IPluginInterface {
private final static Logger LOG = Logger.getLogger(GetValueJNRPEPlugin.class.getName());
private JMole jmole;
public GetValueJNRPEPlugin(JMole jmole) {
this.jmole = jmole;
}
/*
* (non-Javadoc)
*
* @see it.jnrpe.plugins.IPluginInterface#execute(it.jnrpe.ICommandLine)
*/
public ReturnValue execute(ICommandLine cl) {
String category = uglyCheck(cl.getOptionValue('c'));
String name = uglyCheck(cl.getOptionValue('n'));
String attribute = uglyCheck(cl.getOptionValue('a'));
String warningThreshold = cl.getOptionValue('w');
String criticalThreshold = cl.getOptionValue('e');
if (category == null || name == null) {
LOG.log(Level.SEVERE,
"Null values not allowed: Category: " + category + ", name: " + name + ", attribute: " + attribute);
return new ReturnValue(Status.UNKNOWN, "UNKNOWN - Missing parameters");
}
LOG.log(Level.FINE, "Category: " + category + ", name: " + name + ", attribute: " + attribute + ", warning: "
+ warningThreshold + ", critical: " + criticalThreshold);
if (attribute != null) {
Object value;
try {
value = jmole.collectMeasurement(category, name, attribute);
} catch (Exception e) {
LOG.log(Level.SEVERE, e.getMessage(), e);
return new ReturnValue(Status.UNKNOWN, "UNKNOWN - " + e.getMessage());
}
LOG.log(Level.FINE, "Value=" + value);
String keyString = category + "/" + name + "/" + attribute;
if (value == null) {
return new ReturnValue(Status.UNKNOWN, "UNKNOWN - Measurement " + keyString + " not found");
}
String returnString = keyString + "=" + value + "|" + getPerformanceDataKey(category, name, attribute) + "="
+ value;
// Threshold checking
try {
long lValue = Long.parseLong(value.toString());
if (criticalThreshold != null && ThresholdUtil.isValueInRange(criticalThreshold, lValue)) {
LOG.log(Level.FINE, "Value is critical");
return new ReturnValue(Status.CRITICAL, "CRITICAL - " + returnString);
}
if (warningThreshold != null && ThresholdUtil.isValueInRange(warningThreshold, lValue)) {
LOG.log(Level.FINE, "Value is warning");
return new ReturnValue(Status.WARNING, "WARNING - " + returnString);
}
} catch (BadThresholdException e) {
LOG.log(Level.SEVERE, e.getMessage(), e);
return new ReturnValue(Status.UNKNOWN, "UNKNOWN - " + e.getMessage());
}
// OK
LOG.log(Level.FINE, "Returning value: " + value);
return new ReturnValue(Status.OK, "OK - " + returnString);
} else {
// No specific attribute provided so we return all attributes
try {
Map data = jmole.collectMeasurements(category, name);
StringBuilder infoText = new StringBuilder();
StringBuilder performanceData = new StringBuilder();
for (Entry valueEntry : data.entrySet()) {
String tmpAttribute = valueEntry.getKey();
Object value = valueEntry.getValue();
LOG.log(Level.FINE, "Attribute=" + tmpAttribute + " Value=" + value);
String keyString = category + "/" + name + "/" + tmpAttribute;
if (infoText.length() > 0) {
infoText.append(", ");
}
if (performanceData.length() > 0) {
performanceData.append(" ");
}
infoText.append(keyString + "=" + value);
performanceData.append(getPerformanceDataKey(category, name, tmpAttribute) + "=" + value);
}
return new ReturnValue(Status.OK, "OK - " + infoText.toString() + "|" + performanceData.toString());
} catch (Exception e) {
LOG.log(Level.SEVERE, e.getMessage(), e);
return new ReturnValue(Status.UNKNOWN, "UNKNOWN - " + e.getMessage());
}
}
}
// This should be done with the JNRPE API instead
private String uglyCheck(String value) {
if (value.startsWith("$") && value.endsWith("$")) {
return null;
}
return value;
}
// See: https://nagios-plugins.org/doc/guidelines.html#AEN200
private String getPerformanceDataKey(String category, String name, String attribute) {
String answer = category + "/" + name + "/" + attribute;
// Rule 2
answer = answer.replaceAll("=", "");
answer = answer.replaceAll("'", "''");
// Rule 3
return "'" + answer + "'";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy